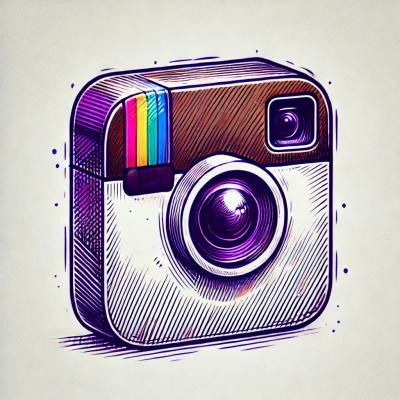
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
osm-read parses openstreetmap XML and PBF files as described in http://wiki.openstreetmap.org/wiki/OSM_XML and http://wiki.openstreetmap.org/wiki/PBF_Format
The following code is used to parse openstreetmap XML or PBF files in a SAX parser like callback way.
var parser = osmread.parse({
filePath: 'path/to/osm.xml',
endDocument: function(){
console.log('document end');
},
bounds: function(bounds){
console.log('bounds: ' + JSON.stringify(bounds));
},
node: function(node){
console.log('node: ' + JSON.stringify(node));
},
way: function(way){
console.log('way: ' + JSON.stringify(way));
},
relation: function(relation){
console.log('relation: ' + JSON.stringify(relation));
},
error: function(msg){
console.log('error: ' + msg);
}
});
// you can pause the parser
parser.pause();
// and resume it again
parser.resume();
The browser bundle 'osm-read-pbf.js' provides a global variable 'pbfParser' with a 'parse' method.
Example, see also example/pbf.html:
<script src="../osm-read-pbf.js"></script>
<script>
pbfParser.parse({
filePath: 'test.pbf',
endDocument: function(){
console.log('document end');
},
node: function(node){
console.log('node: ' + JSON.stringify(node));
},
way: function(way){
console.log('way: ' + JSON.stringify(way));
},
relation: function(relation){
console.log('relation: ' + JSON.stringify(relation));
},
error: function(msg){
console.error('error: ' + msg);
throw msg;
}
});
</script>
As an alternative to passing an URL in "filePath", the option "buffer" can be used to pass an already loaded ArrayBuffer object:
var buf = ... // e.g. xhr.response
pbfParser.parse({
buffer: buf,
...
A third alternative is to let the user choose a local file using the HTML5 File API, passing the file object as "file" option:
<input type="file" id="file" accept=".pbf">
<script>
document.getElementById("file").addEventListener("change", parse, false);
function parse(evt) {
var file = evt.target.files[0];
pbfParser.parse({
file: file,
...
See also example/file.html
Build or update the browser bundle osm-read-pbf.js
with browserify:
$ npm run browserify
To install browserify (http://browserify.org/):
$ npm install -g browserify
Currently you can only parse OSM data in XML from URLs. Here's an example:
osmread.parse({
url: 'http://overpass-api.de/api/interpreter?data=node(51.93315273540566%2C7.567176818847656%2C52.000418429293326%2C7.687854766845703)%5Bhighway%3Dtraffic_signals%5D%3Bout%3B',
format: 'xml',
endDocument: function(){
console.log('document end');
},
bounds: function(bounds){
console.log('bounds: ' + JSON.stringify(bounds));
},
node: function(node){
console.log('node: ' + JSON.stringify(node));
},
way: function(way){
console.log('way: ' + JSON.stringify(way));
},
relation: function(relation){
console.log('relation: ' + JSON.stringify(relation));
},
error: function(msg){
console.log('error: ' + msg);
}
});
The following code allows to create a random access openstreetmap PBF file parser:
osmread.createPbfParser({
filePath: 'path/to/osm.pbf',
callback: function(err, parser){
var headers;
if(err){
// TODO handle error
}
headers = parser.findFileBlocksByBlobType('OSMHeader');
parser.readBlock(headers[0], function(err, block){
console.log('header block');
console.log(block);
parser.close(function(err){
if(err){
// TODO handle error
}
});
});
}
});
Don't forget to close the parser after usage!
Sometimes APIs change... they break your code but things get easier for the rest of us. I'm sorry if a version upgrade gives you some extra hours. To makes things a little less painfull you can find migration instructions in the file ChangeLog.
XML parser:
See file COPYING for details.
author: Markus Peröbner markus.peroebner@gmail.com
FAQs
an openstreetmap XML and PBF data parser
The npm package osm-read receives a total of 4,399 weekly downloads. As such, osm-read popularity was classified as popular.
We found that osm-read demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.