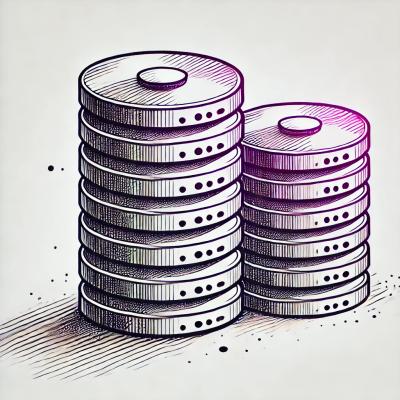
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
pagination-component
Advanced tools
A React component that provides pagination utilities
# npm
npm install --save pagination-component
# yarn
yarn add pagination-component
import React from "react";
import Pagination from "pagination-component";
const containerStyle = {
backgroundColor: "black",
padding: "10px",
borderRadius: "10px",
height: "100px"
};
const itemStyle = {
float: "left",
marginLeft: "5px",
marginRight: "5px",
backgroundColor: "white",
color: "black",
cursor: "pointer",
width: "50px",
textAlign: "center",
borderRadius: "5px"
};
const Paginator = () => (
<div style={containerStyle}>
<Pagination initialPage={1} show={10} pageCount={1024} onChange={page => console.log(page)}>
{({ setPage, page, index, currentPage, isPrev, isNext, isCurrentPage }) => {
if (isPrev)
return (
<div style={itemStyle} onClick={() => setPage({ prev: true })}>
Previous
</div>
);
if (isNext)
return (
<div style={itemStyle} onClick={() => setPage({ next: true })}>
Next
</div>
);
return (
<div
key={index}
style={{ ...itemStyle, backgroundColor: isCurrentPage ? "yellow" : "white" }}
onClick={() => {
console.log(`Navigating from page ${currentPage} to page ${page}`);
setPage({ page });
}}>
<h1>{page}</h1>
</div>
);
}}
</Pagination>
</div>
);
Prop | Type | Description | Default |
---|---|---|---|
initialPage | number | Page to start paginating from. | 1 |
pageCount | number | Total number of pages to paginate. | N/A |
show | number | Number of page controls to show at once (excluding prev and next controls). | 10 |
onChange | ((page: number) => void) | undefined | Callback that receives the next current page. | undefined |
children | (renderProps: PaginationRenderProps) => ReactNode | Render props that receives PaginationRenderProps and returns a React Node (check table below for more info). | undefined |
Render prop | Type | Description |
---|---|---|
setPage | (options: SetPageOptions) => void | Callback used to set the current page. Examples: setPage({ next: true }) // set current page to next page setPage({ prev: true }) // set current page to previous page setPage({ first: true }) // set current page to first page setPage({ last: true }) // set current page to last page setPage({ page: 4 }) // set current page to 4th page Check table below for more info. |
page | number | Indicates which page is currently being rendered. |
index | number | Indicates the index of the page that is currently being rendered (from 0 to show - 1). |
currentPage | number | Indicates the active page in pagination. |
isCurrentPage | boolean | Indicates if the current page control being rendered is the active page control. |
isPrev | boolean | Indicates if the current page control being rendered should be treated as a control to go to the previous page. |
isNext | boolean | Indicates if the current page control being rendered should be treated as a control to go to the next page. |
Option | Type | Description |
---|---|---|
next | boolean | Paginate to the next page. |
prev | boolean | Paginate to the previous page. |
first | boolean | Paginate to the first page. |
last | boolean | Paginate to the last page. |
page | number | Paginate to a specified page. |
git checkout -b my-new-feature
git commit -am 'Add some feature'
git push origin my-new-feature
For more, check out CONTRIBUTING.md
FAQs
A React component that provides pagination utilities
We found that pagination-component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.