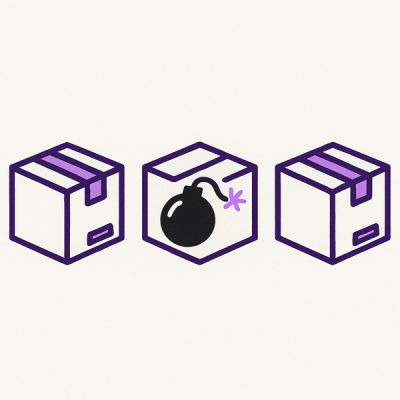
Research
Security News
The Landscape of Malicious Open Source Packages: 2025 Mid‑Year Threat Report
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
paltext-sdk
Advanced tools
A JavaScript/TypeScript SDK for integrating with the PalText Agent API. This SDK allows businesses to easily add AI-powered chat functionality to their applications, with support for user authentication and custom business actions.
A JavaScript/TypeScript SDK for integrating with the PalText Agent API. This SDK allows businesses to easily add AI-powered chat functionality to their applications, with support for user authentication and custom business actions.
npm install paltext-sdk
Or if you're using the SDK directly from the repository:
npm install file:../path/to/sdk-core
import PalTextSDK from 'paltext-sdk';
// Initialize the SDK with your API key
const sdk = new PalTextSDK('your-api-key');
// Initialize a session
const sessionId = await sdk.initSession('user123');
// Send a message
const response = await sdk.sendMessage('Hello, how can I help you?');
// Parse the response with human-readable format
const parsedResponse = sdk.parseAgentResponse(response.message);
console.log(parsedResponse.humanReadable);
import PalTextSDK from 'paltext-sdk';
// Initialize the SDK with your API key
const sdk = new PalTextSDK('your-api-key');
// Initialize a session with authentication
const sessionId = await sdk.initSession('user123', 'auth-token-from-your-system');
// Get user profile
const userProfile = await sdk.getUserProfile();
console.log(`Hello, ${userProfile.name}`);
// Send a message (context is managed by the SDK)
const response = await sdk.sendMessage('Update my shipping address');
// Parse the response
const parsedResponse = sdk.parseAgentResponse(response.message);
// If the response contains an action, execute it
if (parsedResponse.action && parsedResponse.action !== 'none') {
try {
const actionResult = await sdk.executeAction(
parsedResponse.action,
parsedResponse.parameters
);
console.log('Action result:', actionResult);
} catch (error) {
// Check if the error is due to authentication requirements
if (error.message.includes('sign up or log in')) {
console.log('Authentication required for this action');
} else {
console.error('Error executing action:', error);
}
}
}
The SDK automatically checks if actions require authentication:
// Parse the response
const parsedResponse = sdk.parseAgentResponse(response.message);
// Check if the user is authenticated
if (!sdk.isAuthenticated()) {
// If the action requires authentication, the humanReadable response will include a message
if (parsedResponse.humanReadable.includes('need to sign up or log in')) {
console.log('Please log in to perform this action');
// Show login UI or redirect to login page
}
}
// Update user profile (requires authentication)
const updatedProfile = await sdk.updateUserProfile({
name: 'John Doe',
preferences: {
theme: 'dark',
notifications: true
}
});
new PalTextSDK(apiKey: string, options?: { baseUrl?: string })
apiKey
: Your business API keyoptions.baseUrl
: Optional custom API URLasync initSession(userId: string, authToken?: string): Promise<string>
Initializes a user session and returns a session ID.
setAuthToken(authToken: string): void
Sets the authentication token for the current user.
isAuthenticated(): boolean
Returns true if the user has an authentication token.
getBusinessName(): string
Returns the business name or "this business" if not available.
async sendMessage(message: string, context?: Partial<UserContext>): Promise<ChatResponse>
Sends a message to the chat agent and returns the response.
async getUserProfile(): Promise<UserProfile>
Gets the user profile information.
getBusinessActions(): BusinessAction[]
Gets the list of available business actions.
async executeAction(actionId: string, params: Record<string, any>): Promise<any>
Executes a business action with the given parameters. Throws an error if authentication is required but not provided.
async updateUserProfile(updates: Partial<UserProfile>): Promise<UserProfile>
Updates the user profile information. Requires authentication.
parseAgentResponse(message: string): {
action: string;
text: string;
parameters?: Record<string, any>;
result?: any;
humanReadable: string;
}
Parses a JSON response from the agent and adds a human-readable version of the response.
interface UserContext {
userId: string;
userName?: string;
sessionId: string;
authToken?: string;
metadata?: Record<string, any>;
}
interface UserProfile {
userId: string;
name?: string;
email?: string;
preferences?: Record<string, any>;
[key: string]: any;
}
interface BusinessAction {
id: string;
name: string;
description: string;
endpoint: string;
method: 'GET' | 'POST' | 'PUT' | 'DELETE' | 'PATCH';
requiresAuth: boolean;
}
interface BusinessInfo {
id: string;
name: string;
description?: string;
logoUrl?: string;
}
interface ChatResponse {
message: string;
actionResult?: ActionResult;
}
MIT
FAQs
A JavaScript/TypeScript SDK for integrating with the PalText Agent API. This SDK allows businesses to easily add AI-powered chat functionality to their applications, with support for user authentication and custom business actions.
We found that paltext-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.