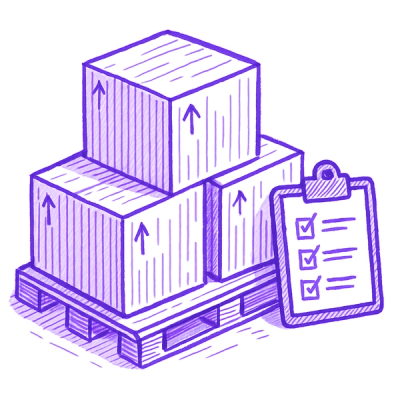
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
parse-bmfont-ascii
Advanced tools
The parse-bmfont-ascii npm package is a utility for parsing BMFont ASCII files. BMFont is a bitmap font generator that allows you to create fonts for use in games and other graphical applications. This package helps in reading and interpreting the ASCII format of BMFont files, making it easier to use these fonts in your projects.
Parsing BMFont ASCII files
This feature allows you to parse a BMFont ASCII file and convert it into a JavaScript object. The code sample demonstrates how to read a BMFont file from the filesystem and parse it using the parse-bmfont-ascii package.
const parseBMFontAscii = require('parse-bmfont-ascii');
const fs = require('fs');
fs.readFile('path/to/font.fnt', 'utf8', (err, data) => {
if (err) throw err;
const font = parseBMFontAscii(data);
console.log(font);
});
The parse-bmfont-xml package is used for parsing BMFont XML files. While parse-bmfont-ascii focuses on the ASCII format, parse-bmfont-xml is designed to handle the XML format of BMFont files. Both packages serve similar purposes but are tailored to different file formats.
The fontpath package is a more general-purpose library for working with various font formats, including BMFont. It provides a broader range of functionalities compared to parse-bmfont-ascii, which is specialized for BMFont ASCII files.
The opentype.js package is a JavaScript parser and writer for OpenType and TrueType fonts. While it does not specifically target BMFont files, it offers extensive capabilities for working with other popular font formats, making it a more versatile but less specialized tool compared to parse-bmfont-ascii.
Parses ASCII (text) BMFont files.
Takes a string or Buffer:
var fs = require('fs')
var parse = require('parse-bmfont-xml')
fs.readFileSync(__dirname+'/Arial.fnt', function(err, data) {
var result = parse(data)
console.log(result.info.face) // "Arial"
console.log(result.pages) // [ 'sheet0.png' ]
console.log(result.chars) // [ ... char data ... ]
console.log(result.kernings) // [ ... kernings data ... ]
})
The spec for the returned JSON object is here. The input data should match the spec, see [test/Nexa Light-32.fnt](test/Nexa Light-32.fnt) for an example.
See text-modules for related modules.
result = parse(data)
Parses data
, a string or Buffer that represents ASCII (text) data of an AngelCode BMFont file. The returned result
object looks like this:
{
pages: [
"sheet_0.png",
"sheet_1.png"
],
chars: [
{ chnl, height, id, page, width, x, y, xoffset, yoffset, xadvance },
...
],
info: { ... },
common: { ... },
kernings: [
{ first, second, amount }
]
}
MIT, see LICENSE.md for details.
FAQs
parses ASCII BMFont files to a JavaScript object
The npm package parse-bmfont-ascii receives a total of 1,087,011 weekly downloads. As such, parse-bmfont-ascii popularity was classified as popular.
We found that parse-bmfont-ascii demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.