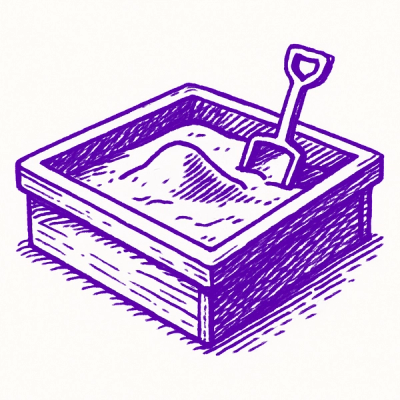
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
passport-oauth1
Advanced tools
The `passport-oauth1` package is a Passport strategy for authenticating with OAuth 1.0a providers. It allows applications to authenticate users using OAuth 1.0a, a protocol for authorization that enables third-party applications to obtain limited access to a user's resources without exposing their credentials.
OAuth 1.0a Authentication
This feature allows you to set up OAuth 1.0a authentication in your application. The code sample demonstrates how to configure the OAuth strategy with the necessary URLs and credentials, and how to handle the authenticated user's profile.
const passport = require('passport');
const OAuthStrategy = require('passport-oauth1').Strategy;
passport.use(new OAuthStrategy({
requestTokenURL: 'https://www.example.com/oauth/request_token',
accessTokenURL: 'https://www.example.com/oauth/access_token',
userAuthorizationURL: 'https://www.example.com/oauth/authorize',
consumerKey: 'your-consumer-key',
consumerSecret: 'your-consumer-secret',
callbackURL: 'https://www.example.com/auth/example/callback'
},
function(token, tokenSecret, profile, done) {
User.findOrCreate({ exampleId: profile.id }, function (err, user) {
return done(err, user);
});
}
));
Token Handling
This feature demonstrates how to handle and store OAuth tokens. The code sample shows how to save the token and tokenSecret for later use, which is essential for making authenticated requests on behalf of the user.
passport.use(new OAuthStrategy({
requestTokenURL: 'https://www.example.com/oauth/request_token',
accessTokenURL: 'https://www.example.com/oauth/access_token',
userAuthorizationURL: 'https://www.example.com/oauth/authorize',
consumerKey: 'your-consumer-key',
consumerSecret: 'your-consumer-secret',
callbackURL: 'https://www.example.com/auth/example/callback'
},
function(token, tokenSecret, profile, done) {
// Store the token and tokenSecret for later use
saveToken(token, tokenSecret);
User.findOrCreate({ exampleId: profile.id }, function (err, user) {
return done(err, user);
});
}
));
function saveToken(token, tokenSecret) {
// Save the token and tokenSecret to your database or session
console.log('Token:', token);
console.log('Token Secret:', tokenSecret);
}
The `passport-oauth2` package is a Passport strategy for authenticating with OAuth 2.0 providers. It is similar to `passport-oauth1` but uses the OAuth 2.0 protocol, which is more modern and widely adopted. It provides a simpler and more secure way to handle authentication compared to OAuth 1.0a.
The `passport-twitter` package is a Passport strategy specifically for authenticating with Twitter using OAuth 1.0a. It is built on top of `passport-oauth1` and provides a more streamlined and easier-to-use interface for integrating Twitter authentication into your application.
The `passport-linkedin-oauth2` package is a Passport strategy for authenticating with LinkedIn using OAuth 2.0. It is similar to `passport-oauth1` in terms of functionality but uses the OAuth 2.0 protocol, which is more secure and easier to implement. It is specifically tailored for LinkedIn authentication.
General-purpose OAuth 1.0 authentication strategy for Passport.
This module lets you authenticate using OAuth in your Node.js applications. By plugging into Passport, OAuth-based sign in can be easily and unobtrusively integrated into any application or framework that supports Connect-style middleware, including Express.
Note that this strategy provides generic OAuth support. In many cases, a provider-specific strategy can be used instead, which cuts down on unnecessary configuration, and accommodates any provider-specific quirks. See the list for supported providers.
Developers who need to implement authentication against an OAuth provider that is not already supported are encouraged to sub-class this strategy. If you choose to open source the new provider-specific strategy, please add it to the list so other people can find it.
:heart: Sponsors
Advertisement
Learn OAuth 2.0 - Get started as an API Security Expert
Just imagine what could happen to YOUR professional career if you had skills in OAuth > 8500 satisfied students
$ npm install passport-oauth1
The OAuth authentication strategy authenticates users using a third-party
account and OAuth tokens. The provider's OAuth endpoints, as well as the
consumer key and secret, are specified as options. The strategy requires a
verify
callback, which receives a token and profile, and calls cb
providing a user.
passport.use(new OAuth1Strategy({
requestTokenURL: 'https://www.example.com/oauth/request_token',
accessTokenURL: 'https://www.example.com/oauth/access_token',
userAuthorizationURL: 'https://www.example.com/oauth/authorize',
consumerKey: EXAMPLE_CONSUMER_KEY,
consumerSecret: EXAMPLE_CONSUMER_SECRET,
callbackURL: "http://127.0.0.1:3000/auth/example/callback",
signatureMethod: "RSA-SHA1"
},
function(token, tokenSecret, profile, cb) {
User.findOrCreate({ exampleId: profile.id }, function (err, user) {
return cb(err, user);
});
}
));
Use passport.authenticate()
, specifying the 'oauth'
strategy, to
authenticate requests.
For example, as route middleware in an Express application:
app.get('/auth/example',
passport.authenticate('oauth'));
app.get('/auth/example/callback',
passport.authenticate('oauth', { failureRedirect: '/login' }),
function(req, res) {
// Successful authentication, redirect home.
res.redirect('/');
});
The test suite is located in the test/
directory. All new features are
expected to have corresponding test cases. Ensure that the complete test suite
passes by executing:
$ make test
All new feature development is expected to have test coverage. Patches that increse test coverage are happily accepted. Coverage reports can be viewed by executing:
$ make test-cov
$ make view-cov
Copyright (c) 2011-2016 Jared Hanson <http://jaredhanson.net/>
[1.3.0] - 2023-03-01
callbackURL
property added to metadata passed to request token store.FAQs
OAuth 1.0 authentication strategy for Passport.
The npm package passport-oauth1 receives a total of 187,910 weekly downloads. As such, passport-oauth1 popularity was classified as popular.
We found that passport-oauth1 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.