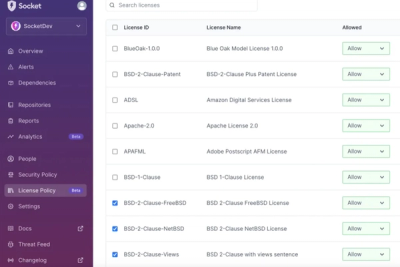
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
periodicjs.core.protocols
Advanced tools
Core protocols is a component of periodicjs.core.controller that exposes a standard set of methods across different transport protcols with options for implementing different API strategies. With core protocols implementing a RESTful API for HTTP requests looks no different than standing up a JSONRPC API for websocket based communications.
const express = require('express');
const mongoose = require('mongoose');
const ProtocolInterface = require('periodicjs.core.protocols');
const DBInterface = require('periodicjs.core.data');
const ResponderInterface = require('periodicjs.core.responder');
//Connect to the DB and start a HTTP server
mongoose.connect();
mongoose.connection.once('open', () => {
//Get the registered model or register a model
let Example = mongoose.model('Example');
let Server = express().listen(3000);
//This configuration will allow for RESTful routes to be generated and mounted for the "Example" mongo collection and will by default respond in JSON
let http_adapter = ProtocolInterface.create({
express: Server,
resources: {},
db: {
example: DBInterface.create({ adapter: 'mongo', model: Example });
},
responder: ResponderInterface.create({ adapter: 'json' }),
api: 'rest',
adapter: 'http'
});
//The implement method called with no arguments will mount a sub-router for each db adapter indexed by model name in the db object
http_adapter.implement();
//http adapter will now have a .router if you wish to access the express router directly
});
/*
With a JSON responder requests will by default receive a JSON response unless it requires a view to be rendered
Implemented RESTful routes
GET localhost:3000/example => Get All
POST localhost:3000/example => Create One
GET localhost:3000/example/:id => Get One
PUT localhost:3000/example/:id => Update One
DELETE localhost:3000/example/:id => Remove One
*/
//In this example assume that mongo is already connected and the HTTP server is already started
let http_adapter = ProtocolInterface.create({
express: require('express'), //Instead of the running server provide the express module
resources: {},
db: {
example: DBInterface.create({ adapter: 'mongo', model: Example })
},
responder: ResponderInterface.create({ adapter: 'json' }),
api: 'rest',
adapter: 'http'
});
//This implements a router specifically for the example model. The .dirname option specifies a view directory if your view files are not in one of the default directories
let router = http_adapter.api.implement({ model_name: 'example', dirname: ['./some/path/to/view/dir'] });
//Because this returns a router you must manually mount it on the applications main router this does however allow for more control over the path
Server.use('/api/v1', router);
/*
Once again the JSON responder will by default be used unless a view must be rendered. If .strict option is passed when constructing the protocol adapter or in the this.api.implement call or this.implement call all responses will come a JSON. Additionally, the JSON responder will be used if req.query.format = "json"
Implemented RESTful routes
GET localhost:3000/api/v1/example?format=json => Get All
POST localhost:3000/api/v1/example?format=json => Create One
GET localhost:3000/api/v1/example/:id?format=json => Get One
PUT localhost:3000/api/v1/example/:id?format=json => Update One
DELETE localhost:3000/api/v1/example/:id?format=json => Remove One
*/
//In this example we are again assuming that mongo is connected and the server is started
let http_adapter = ProtocolInterface.create({
express: Server, //Instead of the running server provide the express module
resources: {},
db: {
example: DBInterface.create({ adapter: 'mongo', model: Example })
},
responder: ResponderInterface.create({ adapter: 'json' }),
api: 'rest',
adapter: 'http'
});
/*
There are two implement methods exposed by this constructed object the first is accessed by .implement and is a method for both implementing an API strategy and mounting whatever is generated from the implementation.
*/
http_adapter.implement();
/*
This method has two potential outcomes dependent on if the http_adapter.express property is an already running express server or the express module itself. Another key difference is that this .implement method only uses the .express property of the protocol adapter.
Case 1 (express server):
.implement() directly mounts routes on the application
sets the .router property equal to the ._router property of the express application
Case 2 (express module):
.implement() creates an express router and mounts routes
sets the .router property equal to the generated router
must separately mount router to application main router
Both
defines http_adapter.controller[model_name] as the generated controller methods for model
*/
let router = http_adapter.api.implement({ model_name: 'example' });
/*
This method is similar to http_adapter.implement with the key difference being that it implements an API strategy given any router and model and returns the values so that they can be used outside of the ecosystem of the protocol adapter. As such this method can be passed an optional .router property and will mount routes on the provided router. Only when this option is not defined will this method use the http_adapter.express router.
Optionally .router can also be set to false in which case no router will be created and only controller methods are returned.
*/
console.log(router);
/*
{
new,
show,
edit,
index,
remove,
search,
create,
update,
load,
load_with_count,
load_with_limit,
paginate,
router <- When options.router is not false
}
*/
Make sure you have grunt installed
$ npm install -g grunt-cli jsdoc-to-markdown
For generating documentation
$ grunt doc
$ jsdoc2md adapters/**/*.js api_adapters/**/*.js utility/**/*.js index.js > doc/api.md
$ npm i
$ grunt test
MIT
FAQs
Customizable CMS platform
The npm package periodicjs.core.protocols receives a total of 5 weekly downloads. As such, periodicjs.core.protocols popularity was classified as not popular.
We found that periodicjs.core.protocols demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.