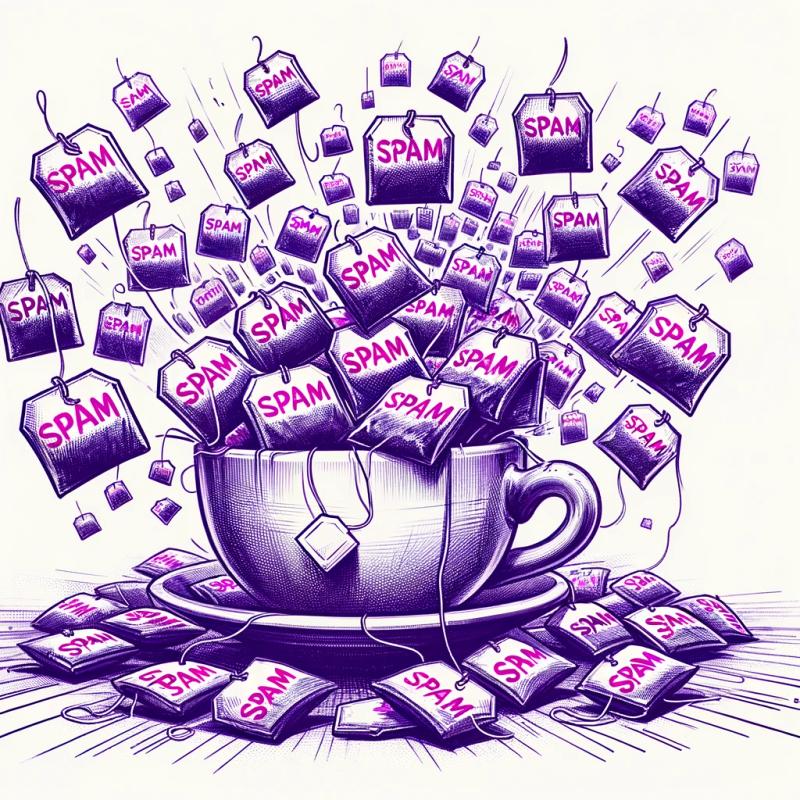
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
piopiy
Advanced tools
Readme
The Node.js SDK is used to integrate communications into your Node.js applications using the PIOPIY REST API. Using the SDK, you will be able to make voice calls and can control your call flows.
Follow the below installation instructions
Prerequisites for javascript web server.
Install the SDK using npm
$ npm install piopiy
In order to authenticate your app, and to make an API request, you should have an app id and secret for authentication. Find your App ID and secret in your PIOPIY dashboard
Specifiy the authentication credentials
const Piopiy = require( 'piopiy' );
const piopiy = new Piopiy( 'your_app_id', 'your_app_secret' );
To make a call , mention the to_number, piopiy_phone_number and .
piopiy.voice.call(
'your_leg_a_to_number',
'your_piopiy_phone_number',
'your_leg_b_to_number'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
To make a call using PCMO, mention the to_number, piopiy_phone_number and .
piopiy.voice.callPCMO(
'your_to_number',
'your_piopiy_phone_number',
'your_PCMO_Object'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
To make a call with answer, mention the to_number, piopiy_phone_number and answer_url.
piopiy.voice.make(
'your_to_number',
'your_piopiy_phone_number',
'your_answer_url'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
To hold a call, mention the cmiuuid of the call.
piopiy.voice.hold(
'cmiuuid'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
To unhold a call, mention the cmiuuid of the call.
piopiy.voice.unhold(
'cmiuuid'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
To toggle a call, mention the cmiuuid of the call.
piopiy.voice.toggle(
'cmiuuid'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
To hangup a call, mention the cmiuuid of the call.
piopiy.voice.hangup(
'cmiuuid'
).then( ( result ) => {
console.log( result )
} ).catch( ( error ) => {
console.log( error )
} );
Refer to the piopiy docs for more examples. Now create the PIOPIY account and setup the express server and test out your integration in few minutes.
For any feedbacks and problems, you can open an issue on github.
FAQs
PIOPIY REST API client for nodejs,It support Voice,SMS and more.
The npm package piopiy receives a total of 0 weekly downloads. As such, piopiy popularity was classified as not popular.
We found that piopiy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.