Comparing version
@@ -0,1 +1,8 @@ | ||
## Version 0.2.1, 2018.11.23 | ||
* replaced grunt build with npm scripts [07044b1](https://github.com/neocotic/pollock/commit/07044b124149435ecb5a0f44c8295dff758302f2) | ||
* replaced chai with assert [0acf083](https://github.com/neocotic/pollock/commit/0acf083255f6514e9f324585241a4b621706377a) | ||
* switched download links from RawGit to unpkg [4c47295](https://github.com/neocotic/pollock/commit/4c47295fa7ded4cccb0db38b7a4e6d859da95011) | ||
* switched image link from RawGit to GitHack [3b08d42](https://github.com/neocotic/pollock/commit/3b08d42bf38896efe23564aface93e155ee92720) | ||
## Version 0.2.0, 2018.11.22 | ||
@@ -2,0 +9,0 @@ |
(function (global, factory) { | ||
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() : | ||
typeof define === 'function' && define.amd ? define(factory) : | ||
typeof define === 'function' && define.amd ? define('pollock', factory) : | ||
(global.pollock = factory()); | ||
@@ -97,6 +97,7 @@ }(this, (function () { 'use strict'; | ||
return pollock_1; | ||
var pollock$1 = pollock_1; | ||
return pollock$1; | ||
}))); | ||
//# sourceMappingURL=pollock.js.map | ||
//# sourceMappingURL=pollock.js.map |
@@ -1,102 +0,3 @@ | ||
/*! pollock v0.2.0 | (C) 2018 Alasdair Mercer | MIT License */ | ||
(function (global, factory) { | ||
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() : | ||
typeof define === 'function' && define.amd ? define(factory) : | ||
(global.pollock = factory()); | ||
}(this, (function () { 'use strict'; | ||
/* | ||
* Copyright (C) 2018 Alasdair Mercer | ||
* | ||
* Permission is hereby granted, free of charge, to any person obtaining a copy | ||
* of this software and associated documentation files (the "Software"), to deal | ||
* in the Software without restriction, including without limitation the rights | ||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
* copies of the Software, and to permit persons to whom the Software is | ||
* furnished to do so, subject to the following conditions: | ||
* | ||
* The above copyright notice and this permission notice shall be included in all | ||
* copies or substantial portions of the Software. | ||
* | ||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
* SOFTWARE. | ||
*/ | ||
/** | ||
* Assigns an abstract method with a given name to the specified <code>type</code> based on the <code>options</code> | ||
* provided which, when called, will result in an error containing a suitable error being thrown. The aim is to quickly | ||
* highlight to consumers of your API that they an abstract method has not been implemented in a fail-fast fashion. | ||
* | ||
* If the <code>typeName</code> option is specified, it will be used instead of the name of <code>type</code>. If | ||
* <code>type</code> has no name, for whatever reason, <code>"<anonymous>"</code> will be used. | ||
* | ||
* If the <code>static</code> option is enabled, the created abstract method will assigned directly to <code>type</code> | ||
* instead of <code>type.prototype</code>. | ||
* | ||
* While the default behaviour is synchronous in design, as it simply throws the error, it may be necessary to support | ||
* reporting the error using asynchronous patterns. The <code>callback</code> option can be specifed to indicate the | ||
* expected index of the callback function to be passed to the abstract method. This index can be negative if it should | ||
* be applied from the end of the argument list instead of the beginning. Once identified, the abstract method will | ||
* invoke the callback function with the error as the first argument. Alternatively, the <code>promise</code> option can | ||
* be enabled for the method to return a rejected ECMAScript 2015 <code>Promise</code> instead. | ||
* | ||
* Regardless of which asynchronous pattern is used, both options only indicate a preference. If no callback function is | ||
* found at the specified index or if the current environment does not support promises, the abstract method will fall | ||
* back on the default behaviour of simply throwing the error. | ||
* | ||
* @param {Function} type - the constructor function to which the abstract method is to be assigned | ||
* @param {string} methodName - the name of the abstract method to be assigned | ||
* @param {pollock~Options} [options] - the options to be used | ||
* @return {void} | ||
* @public | ||
*/ | ||
function pollock(type, methodName, options) { | ||
if (!options) { | ||
options = {}; | ||
} | ||
function abstractMethod() { | ||
var typeName = options.typeName || type.name || '<anonymous>'; | ||
var separator = options.static ? '.' : '#'; | ||
var error = new Error(typeName + separator + methodName + ' abstract method is not implemented'); | ||
var callback; | ||
if (options.callback != null && (callback = findCallback(arguments, options.callback))) { | ||
return callback(error); | ||
} else if (options.promise && typeof Promise !== 'undefined') { | ||
return Promise.reject(error); | ||
} | ||
throw error; | ||
} | ||
if (options.static) { | ||
type[methodName] = abstractMethod; | ||
} else { | ||
type.prototype[methodName] = abstractMethod; | ||
} | ||
} | ||
function findCallback(args, index) { | ||
if (index < 0) { | ||
index = args.length + index; | ||
} | ||
index = Math.max(index, 0); | ||
var callback = args[index]; | ||
return typeof callback === 'function' ? callback : null; | ||
} | ||
var pollock_1 = pollock; | ||
return pollock_1; | ||
}))); | ||
//# sourceMappingURL=pollock.min.js.map | ||
/*! pollock v0.2.1 | (C) 2018 Alasdair Mercer | MIT License */ | ||
!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?module.exports=t():"function"==typeof define&&define.amd?define("pollock",t):e.pollock=t()}(this,function(){"use strict";return function(i,r,c){function e(){var e,t=c.typeName||i.name||"<anonymous>",n=c.static?".":"#",o=new Error(t+n+r+" abstract method is not implemented");if(null!=c.callback&&(e=function(e,t){t<0&&(t=e.length+t),t=Math.max(t,0);var n=e[t];return"function"==typeof n?n:null}(arguments,c.callback)))return e(o);if(c.promise&&"undefined"!=typeof Promise)return Promise.reject(o);throw o}c||(c={}),c.static?i[r]=e:i.prototype[r]=e}}); | ||
//# sourceMappingURL=pollock.min.js.map |
{ | ||
"name": "pollock", | ||
"version": "0.2.0", | ||
"version": "0.2.1", | ||
"description": "Simple lightweight library for adding abstract methods to types", | ||
@@ -24,26 +24,29 @@ "homepage": "https://github.com/neocotic/pollock", | ||
"devDependencies": { | ||
"chai": "^4.2.0", | ||
"codecov": "^3.1.0", | ||
"eslint": "^5.9.0", | ||
"eslint-config-notninja": "^0.4.0", | ||
"grunt": "^1.0.3", | ||
"grunt-cli": "^1.3.2", | ||
"grunt-contrib-clean": "^2.0.0", | ||
"grunt-eslint": "^21.0.0", | ||
"grunt-mocha-istanbul": "^5.0.2", | ||
"grunt-rollup": "^9.0.0", | ||
"istanbul": "^0.4.5", | ||
"load-grunt-tasks": "^4.0.0", | ||
"mocha": "^5.2.0", | ||
"rollup-plugin-commonjs": "^8.4.1", | ||
"npm-run-all": "^4.1.3", | ||
"nyc": "^13.1.0", | ||
"rimraf": "^2.6.2", | ||
"rollup": "^0.67.3", | ||
"rollup-plugin-commonjs": "^9.2.0", | ||
"rollup-plugin-node-resolve": "^3.4.0", | ||
"rollup-plugin-uglify": "^6.0.0" | ||
}, | ||
"main": "src/pollock.js", | ||
"main": "index.js", | ||
"browser": "dist/pollock.js", | ||
"scripts": { | ||
"build": "grunt build", | ||
"ci": "grunt ci", | ||
"report-coverage": "codecov", | ||
"test": "grunt test" | ||
"build": "npm-run-all lint compile test", | ||
"clean": "npm-run-all clean:*", | ||
"clean:coverage": "rimraf coverage", | ||
"clean:dist": "rimraf dist", | ||
"compile": "npm run clean:dist && rollup --config", | ||
"coverage": "nyc report && codecov", | ||
"lint": "npm-run-all lint:*", | ||
"lint:src": "eslint \"src/**/*.js\"", | ||
"lint:test": "eslint \"test/**/*.js\"", | ||
"test": "npm run clean:coverage && nyc mocha -R list \"test/**/*.spec.js\"", | ||
"posttest": "nyc check-coverage" | ||
} | ||
} |
@@ -1,2 +0,2 @@ | ||
[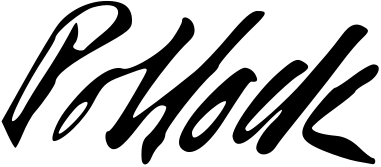](https://github.com/neocotic/pollock) | ||
[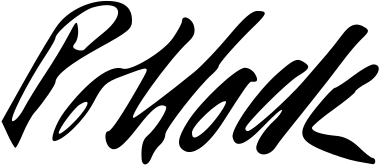](https://github.com/neocotic/pollock) | ||
@@ -30,4 +30,4 @@ A simple lightweight JavaScript library for adding abstract methods to types which, when called, report a useful error | ||
* [Development Version](https://cdn.rawgit.com/neocotic/pollock/master/dist/pollock.js) (4.6kb - [Source Map](https://cdn.rawgit.com/neocotic/pollock/master/dist/pollock.js.map)) | ||
* [Production Version](https://cdn.rawgit.com/neocotic/pollock/master/dist/pollock.min.js) (722b - [Source Map](https://cdn.rawgit.com/neocotic/pollock/master/dist/pollock.min.js.map)) | ||
* [Development Version](https://unpkg.com/pollock/dist/pollock.js) (4.6kb - [Source Map](https://unpkg.com/pollock/dist/pollock.js.map)) | ||
* [Production Version](https://unpkg.com/pollock/dist/pollock.min.js) (723b - [Source Map](https://unpkg.com/pollock/dist/pollock.min.js.map)) | ||
@@ -34,0 +34,0 @@ ## API |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
39287
2.88%11
-26.67%12
20%280
3.7%