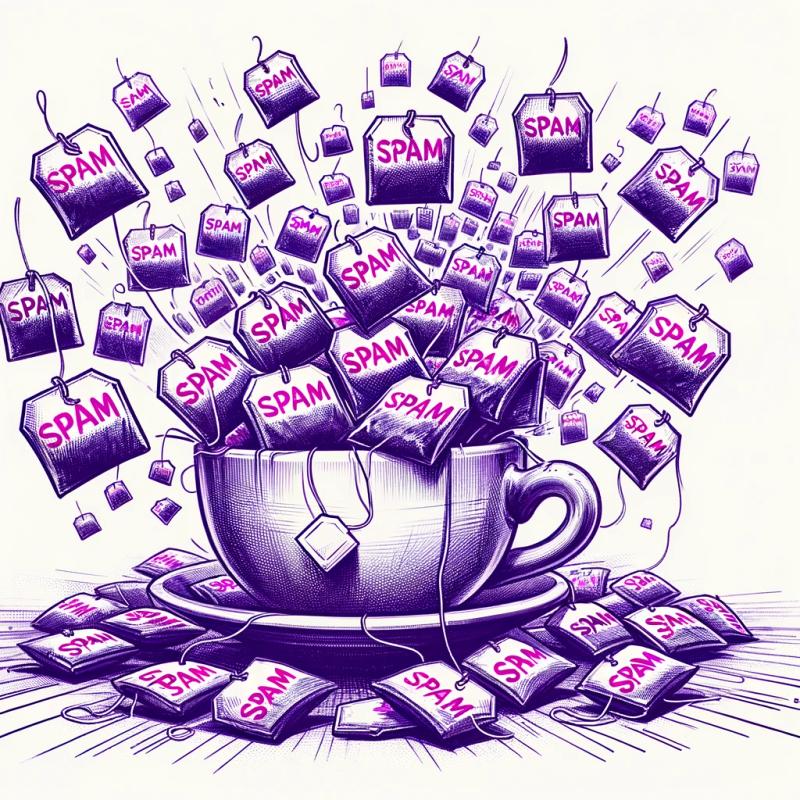
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
postgres-range
Advanced tools
Package description
The postgres-range npm package is designed to parse and serialize PostgreSQL range types. It supports various operations on range data, such as checking if a range contains a value, if one range contains another, if ranges overlap, and more. This package is particularly useful when working with PostgreSQL databases in Node.js applications that require manipulation or interpretation of range data.
Parsing range strings
This feature allows for the parsing of PostgreSQL range strings into Range objects. The example demonstrates parsing an integer range string.
"const { Range } = require('postgres-range');
const intRange = Range.parse('[1,5)');
console.log(intRange);"
Checking if a range contains a value
This feature enables checking whether a given value is contained within a range. The example shows how to check if the number 3 is within the range [1,5), which returns true, and if the number 5 is within the same range, which returns false.
"const { Range } = require('postgres-range');
const intRange = new Range(1, 5);
console.log(intRange.contains(3)); // true
console.log(intRange.contains(5)); // false"
Serializing range objects to strings
This feature allows for the serialization of Range objects back into PostgreSQL range strings. The example demonstrates serializing a Range object representing the range [1,5).
"const { Range } = require('postgres-range');
const intRange = new Range(1, 5, '[)');
console.log(intRange.toString()); // '[1,5)'"
Similar to postgres-range, pg-range is designed to work with PostgreSQL range types in Node.js. It provides functionality for parsing and formatting range types. The main difference is in the API design and the extent of utility functions provided for range manipulation.
While sequelize-range is built to integrate PostgreSQL range types with Sequelize, an ORM for Node.js, it offers similar parsing and serialization capabilities for range types. The key difference lies in its integration with Sequelize, making it more suitable for applications that use Sequelize for database interactions.
Readme
Parse postgres range columns
npm install --save postgres-range
const range = require('postgres-range')
const rng = range.parse('[0,5)', (value) => parseInt(value, 10))
rng.isBounded()
// => true
rng.isLowerBoundClosed()
// => true
rng.isUpperBoundClosed()
// => false
rng.hasLowerBound()
// => true
rng.hasUpperBound()
// => true
rng.containsPoint(4)
// => true
rng.containsRange(range.parse('[1,2]', x => parseInt(x)))
// => true
range.parse('empty').isEmpty()
// => true
range.serialize(new range.Range(0, 5))
// => '(0,5)'
range.serialize(new range.Range(0, 5, range.RANGE_LB_INC | RANGE_UB_INC))
// => '[0,5]'
parse(input, [transform])
-> Range
Required
Type: string
A Postgres range string.
Type: function
Default: identity
A function that transforms non-null bounds of the range.
serialize(range, [format])
-> string
Required
Type: Range
A Range
object.
Type: function
Default: identity
A function that formats non-null bounds of the range.
MIT © Abbas Mashayekh
FAQs
Range data type parser and serializer for PostgreSQL
The npm package postgres-range receives a total of 1,300,269 weekly downloads. As such, postgres-range popularity was classified as popular.
We found that postgres-range demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.