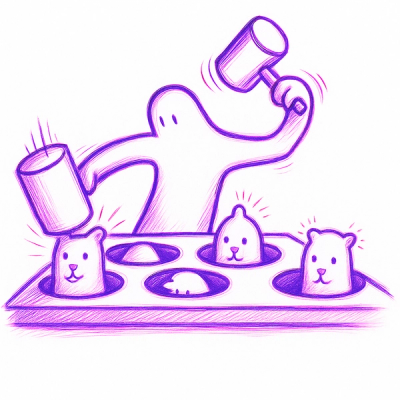
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
precise-typeof
Advanced tools
Better 'typeof'. Detects real type of the objects like 'Array()', 'new Number(1)', 'new Boolean(true)', etc.
Better typeof
. Detects real type of the objects like Array()
, new Number(1)
, new Boolean(true)
, etc.
compression | size |
---|---|
precise-typeof.js | 1.69 kB |
precise-typeof.min.js | 809 B |
precise-typeof.min.js.gz | 391 B |
$ yarn add precise-typeof
or
$ npm install --save precise-typeof
var typeOf = require('precise-typeof');
typeOf({}); // -> 'object'
typeOf(new function(){}); // -> 'object'
typeOf([]); // -> 'array'
typeOf(25); // -> 'number'
typeOf(Infinity); // -> 'number'
typeOf('ABC'); // -> 'string'
typeOf(function(){}); // -> 'function'
typeOf(Math.sin); // -> 'function'
typeOf(undefined); // -> 'undefined'
typeOf(true); // -> 'boolean'
typeOf(null); // -> 'null'
typeOf(NaN); // -> 'nan'
// object values
typeOf(new Object()); // -> 'object'
typeOf(new Array()); // -> 'array'
typeOf(new Number(5)); // -> 'number'
typeOf(new Number(Infinity)); // -> 'number'
typeOf(new String('ABC')); // -> 'string'
typeOf(new Function('a', 'b', 'return a + b')); // -> 'function'
typeOf(new Boolean()); // -> 'boolean'
typeOf(new Number('blabla')); // -> 'nan'
// instances
typeOf(new function Moment(){}); // -> 'object'
// special objects
typeOf(/s/); // -> 'regexp'
typeOf(new Date()); // -> 'date'
typeOf(Math); // -> 'math'
typeOf(new Error()); // -> 'error'
typeOf(arguments); // -> 'arguments'
// node
typeOf(global); // -> 'global'
typeOf(process); // -> 'process'
typeOf(Buffer('B')); // -> 'buffer'
typeOf(new Buffer(2)); // -> 'buffer'
typeOf(Buffer([62, 64, 66])); // -> 'buffer'
// es6
typeOf(Symbol('A')); // -> 'symbol'
// browser
typeOf(window); // -> 'global'
typeOf(document); // -> 'html'
typeOf(document.body); // -> 'html'
typeOf(document.getElementsByTagName('html')[0]); // -> 'html'
typeOf(document.getElementsByTagName('div')); // -> 'html'
typeOf(document.createElement('a')); // -> 'html'
typeOf(document.createTextNode('Abcd')); // -> 'text'
typeOf(document.createComment('abcd')); // -> 'comment'
typeOf(document.createEvent('Event')); // -> 'event'
typeOf(document.createEvent('UIEvents')); // -> 'event'
typeOf(document.createEvent('HTMLEvents')); // -> 'event'
typeOf(document.createEvent('MouseEvents')); // -> 'event'
{ pojoOnly: true }
With pojoOnly
flag it only reports Plain-Old Javascript Objects as object
,
and reports "instances" by their constructor names (e.g. Moment
for moment
instance).
In case if object was created from the nameless function, it will be reported as unknown
.
var typeOf = require('precise-typeof');
// reported differently with `{pojoOnly: true}`
typeOf(new function Moment(){}, {pojoOnly: true}); // -> 'Moment'
typeOf(new function ABC(){}, {pojoOnly: true}); // -> 'ABC'
typeOf(new function(){}, {pojoOnly: true}); // -> 'unknown'
// same with or without `{pojoOnly: true}`
typeOf({}, {pojoOnly: true}); // -> 'object'
typeOf([], {pojoOnly: true}); // -> 'array'
typeOf(25, {pojoOnly: true}); // -> 'number'
typeOf(Infinity, {pojoOnly: true}); // -> 'number'
typeOf('ABC', {pojoOnly: true}); // -> 'string'
typeOf(function(){}, {pojoOnly: true}); // -> 'function'
typeOf(Math.sin, {pojoOnly: true}); // -> 'function'
typeOf(undefined, {pojoOnly: true}); // -> 'undefined'
typeOf(true, {pojoOnly: true}); // -> 'boolean'
typeOf(null, {pojoOnly: true}); // -> 'null'
typeOf(NaN, {pojoOnly: true}); // -> 'nan'
typeOf(new Object(), {pojoOnly: true}); // -> 'object'
typeOf(new Array(), {pojoOnly: true}); // -> 'array'
typeOf(new Number(5), {pojoOnly: true}); // -> 'number'
typeOf(new Number(Infinity), {pojoOnly: true}); // -> 'number'
typeOf(new String('ABC'), {pojoOnly: true}); // -> 'string'
typeOf(new Function(), {pojoOnly: true}); // -> 'function'
typeOf(new Boolean(), {pojoOnly: true}); // -> 'boolean'
typeOf(new Number('blabla'), {pojoOnly: true}); // -> 'nan'
Precise-TypeOf is released under the MIT license.
FAQs
Better 'typeof'. Detects real type of the objects like 'Array()', 'new Number(1)', 'new Boolean(true)', etc.
The npm package precise-typeof receives a total of 842 weekly downloads. As such, precise-typeof popularity was classified as not popular.
We found that precise-typeof demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.