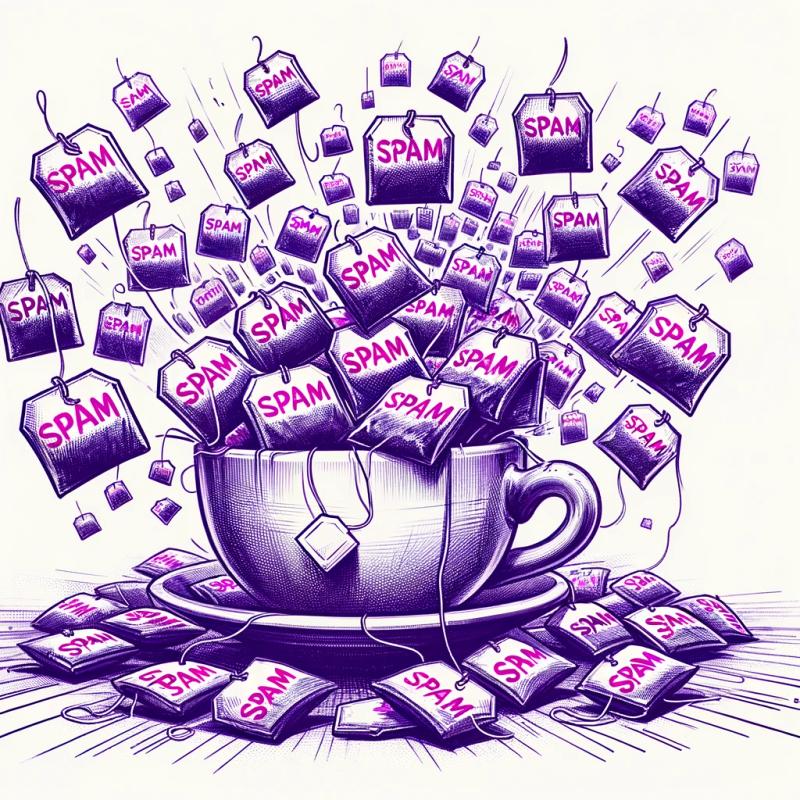
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
priorityqueue
Advanced tools
Readme
An implementation of priority queue in javascript.
npm install priorityqueue
import PriorityQueue from "priorityqueue";
class Point {
constructor(x, y) {
this.x = x;
this.y = y;
}
}
const numericCompare = (a, b) => (a > b ? 1 : a < b ? -1 : 0);
const comparator = (a, b) => {
const x = numericCompare(a.x, b.x);
const y = numericCompare(a.y, b.y);
return x ? x : y;
};
const pq = new PriorityQueue({ comparator });
pq.push(new Point(4, 6));
pq.push(new Point(2, 3));
pq.push(new Point(5, 1));
pq.push(new Point(1, 2));
console.log(pq.pop()); // => {x: 5, y: 1}
console.log(pq.top()); // => {x: 4, y: 6}
pq.push(new Point(3, 4));
pq.push(new Point(6, 5));
console.log(pq.length); // => 5
console.log(pq.top()); // => {x: 6, y: 5}
Binary heap is a simple and efficient in almost cases.
cons:
merge
operation especiallypros:
merge
operation(constant time)pros:
merge
operation(constant time)Not to use:
import PriorityQueue from "priorityqueue";
import BinaryHeap from "priorityqueue/BinaryHeap";
import PairingHeap from "priorityqueue/PairingHeap";
import SkewHeap from "priorityqueue/SkewHeap";
console.log(PriorityQueue === BinaryHeap); // => true
FAQs
An implementation of Priority Queue
The npm package priorityqueue receives a total of 477 weekly downloads. As such, priorityqueue popularity was classified as not popular.
We found that priorityqueue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.