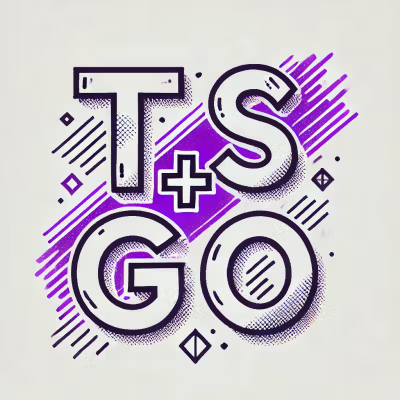
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
prisma-class-dto-generator
Advanced tools
Generate Prisma DTOs with seamless class-validator and class-transformer integration for TypeScript applications.
❗🔴❗ Starting from version 7.0.0, prisma-class-dto-generator
is no longer a Prisma generator but a standalone CLI tool and library that can be used independently. The package has been migrated to ESM (ECMAScript Modules) with NextNode to ensure compatibility with modern Node.js environments.
New: Prisma DTO Generator UI Constructor is an intuitive UI tool that helps generate DTO configurations for Prisma without manual edits. Define your settings visually, export a generator-config.json
, and seamlessly integrate DTO generation into your workflow.
You can use prisma-class-dto-generator
in two ways:
npx prisma-class-dto-generator --path=./prisma/schema.prisma --output=./dto_generated
or install globally:
npm install -g prisma-class-dto-generator
prismadtogen --path=./prisma/schema.prisma --output=./dto_generated
Usage: prismadtogen --path=[path_to_schema]
Options:
--help, -h Show this help message
--version, -v Show the installed version
--path=[path] Specify a Prisma schema file (default: ./prisma/schema.prisma)
--output=[path] Specify the output directory (default: ./dto_generated)
✅ Standalone & Flexible – Not tied to Prisma's generator system. ✅ ESM & NextNode Support – Fully compatible with modern Node.js environments. ✅ Error-Free DTO Generation – Automates repetitive DTO creation. ✅ Consistent & Maintainable – Ensures uniform DTO structures.
You can also use it inside a Node.js project:
import { generate } from "prisma-class-dto-generator";
await generate({
cwd: process.cwd(),
schemaPath: "./prisma/schema.prisma",
output: "./dto_generated"
});
@filterable
, @exclude input|output
, @listable
, and @orderable
annotations.@IsFile
and @IsFiles
decorators for file uploads.class-validator
, class-transformer
, and routing-controllers-openapi
.npm install prisma-class-dto-generator
or
yarn add prisma-class-dto-generator
The tool allows configuring DTO generation via a JSON file:
{
"input": {
"extendModels": {
"Item": {
"fields": [
{ "name": "title", "isRequired": false }
]
}
}
}
}
To integrate with Prisma, add a generator entry in schema.prisma
:
generator class_validator {
provider = "node node_modules/prisma-class-dto-generator"
output = "../src/dto_sources"
configPath = "./"
}
Developed by unbywyd.
FAQs
Generate Prisma DTOs with seamless class-validator and class-transformer integration for TypeScript applications.
We found that prisma-class-dto-generator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.