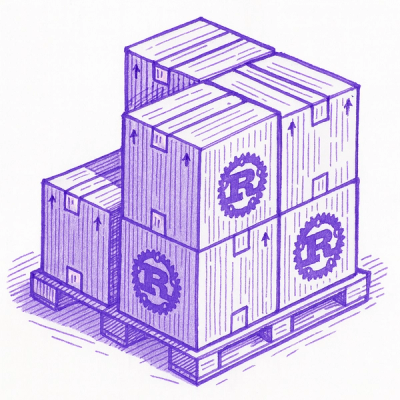
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
promise-limit
Advanced tools
The promise-limit npm package allows you to limit the number of concurrent promises that are executed. This is particularly useful when dealing with a large number of asynchronous operations that could overwhelm system resources if executed all at once.
Limit Concurrent Promises
This feature allows you to limit the number of concurrent promises. In this example, only 2 promises will run at the same time. The `limit.map` function takes an array of tasks and ensures that only the specified number of tasks run concurrently.
const limit = require('promise-limit')(2);
const tasks = [
() => new Promise(resolve => setTimeout(() => resolve('Task 1'), 1000)),
() => new Promise(resolve => setTimeout(() => resolve('Task 2'), 500)),
() => new Promise(resolve => setTimeout(() => resolve('Task 3'), 300)),
() => new Promise(resolve => setTimeout(() => resolve('Task 4'), 200))
];
limit.map(tasks, task => task()).then(results => {
console.log(results); // ['Task 1', 'Task 2', 'Task 3', 'Task 4']
});
Limit Concurrent Promises with Error Handling
This feature demonstrates how to handle errors when limiting concurrent promises. If any promise rejects, the error will be caught and handled appropriately.
const limit = require('promise-limit')(2);
const tasks = [
() => new Promise((resolve, reject) => setTimeout(() => reject('Error in Task 1'), 1000)),
() => new Promise(resolve => setTimeout(() => resolve('Task 2'), 500)),
() => new Promise(resolve => setTimeout(() => resolve('Task 3'), 300)),
() => new Promise(resolve => setTimeout(() => resolve('Task 4'), 200))
];
limit.map(tasks, task => task()).then(results => {
console.log(results); // ['Error in Task 1', 'Task 2', 'Task 3', 'Task 4']
}).catch(error => {
console.error(error);
});
The p-limit package provides similar functionality by limiting the number of concurrent promise executions. It offers a more flexible API and better integration with async/await syntax. Unlike promise-limit, p-limit returns a function that you can call with your promise-returning functions.
The promise-pool package also limits the number of concurrent promises. It provides a more feature-rich API, including support for retries and timeouts. It is more suitable for complex scenarios where additional control over promise execution is required.
The async package is a utility module that provides various functions for working with asynchronous JavaScript. It includes a `queue` function that can limit the number of concurrent tasks, similar to promise-limit. However, async is a more comprehensive library with many other utilities for managing asynchronous code.
Limit outstanding calls to promise returning functions, or a semaphore for promises. You might want to do this to reduce load on external services, or reduce memory usage when processing large batches of jobs.
npm install promise-limit
var promiseLimit = require('promise-limit')
var limit = promiseLimit(2)
var jobs = ['a', 'b', 'c', 'd', 'e']
Promise.all(jobs.map((name) => {
return limit(() => job(name))
})).then(results => {
console.log()
console.log('results:', results)
})
function job (name) {
var text = `job ${name}`
console.log('started', text)
return new Promise(function (resolve) {
setTimeout(() => {
console.log(' ', text, 'finished')
resolve(text)
}, 100)
})
}
will output:
started job a
started job b
job a finished
job b finished
started job c
started job d
job c finished
job d finished
started job e
job e finished
results: [ 'job a', 'job b', 'job c', 'job d', 'job e' ]
var promiseLimit = require('promise-limit')
promiseLimit(concurrency?: Number) -> limit
Returns a function that can be used to wrap promise returning functions, limiting them to concurrency
outstanding calls.
concurrency
the concurrency, i.e. 1 will limit calls to one at a time, effectively in sequence or serial. 2 will allow two at a time, etc. 0 or undefined
specify no limit, and all calls will be run in parallel.limit(fn: () -> Promise<T>) -> Promise<T>
A function that limits calls to fn
, based on concurrency
above. Returns a promise that resolves or rejects the same value or error as fn
. All functions are executed in the same order in which they were passed to limit
. fn
must return a promise.
fn
a function that is called with no arguments and returns a promise. You can pass arguments to your function by putting it inside another function, i.e. () -> myfunc(a, b, c)
.limit.map(items: [T], mapper: (T) -> Promise<R>) -> Promise<[R]>
Maps an array of items using mapper
, but limiting the number of concurrent calls to mapper
with the concurrency
of limit
. If at least one call to mapper
returns a rejected promise, the result of map
is a the same rejected promise, and no further calls to mapper
are made.
limit.queue: Number
Returns the queue length, the number of jobs that are waiting to be started. You could use this to throttle incoming jobs, so the queue doesn't overwhealm the available memory - for e.g. pause()
a stream.
Featurist provides full stack, feature driven development teams. Want to join us? Check out our career opportunities.
FAQs
limits calls to functions that return promises
The npm package promise-limit receives a total of 578,081 weekly downloads. As such, promise-limit popularity was classified as popular.
We found that promise-limit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.