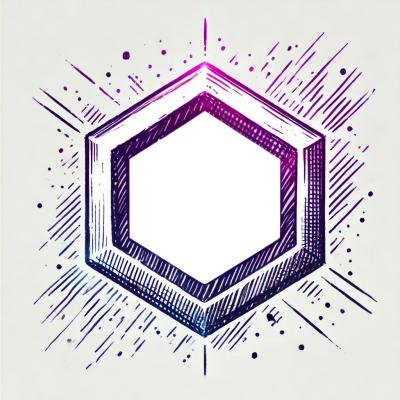
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
proxy-agent
Advanced tools
The proxy-agent npm package is a module that provides an HTTP(s) proxy Agent which can be used to proxy HTTP and HTTPS requests through a specified proxy server. This is useful in scenarios where network requests need to be routed through a proxy for reasons such as security, privacy, or circumventing network restrictions.
Creating an HTTP proxy agent
This feature allows you to create an HTTP proxy agent that will route HTTP requests through the specified proxy server.
const ProxyAgent = require('proxy-agent');
const agent = new ProxyAgent('http://proxy-server.com:8080');
const http = require('http');
const requestOptions = {
host: 'example.com',
port: 80,
path: '/',
agent: agent
};
http.get(requestOptions, (res) => {
console.log(`Got response: ${res.statusCode}`);
});
Creating an HTTPS proxy agent
This feature allows you to create an HTTPS proxy agent that will route HTTPS requests through the specified proxy server using TLS/SSL encryption.
const ProxyAgent = require('proxy-agent');
const agent = new ProxyAgent('https://proxy-server.com:443');
const https = require('https');
const requestOptions = {
host: 'example.com',
port: 443,
path: '/',
agent: agent
};
https.get(requestOptions, (res) => {
console.log(`Got response: ${res.statusCode}`);
});
Support for different proxy protocols
The proxy-agent package supports various proxy protocols including HTTP, HTTPS, SOCKS, and PAC (Proxy Auto-Config).
const ProxyAgent = require('proxy-agent');
const httpAgent = new ProxyAgent('http://proxy-server.com:8080');
const httpsAgent = new ProxyAgent('https://proxy-server.com:443');
const socksAgent = new ProxyAgent('socks://proxy-server.com:1080');
const pacAgent = new ProxyAgent('pac+http://proxy-server.com/proxy.pac');
// Use the appropriate agent for the request protocol
This package provides an HTTP agent to proxy HTTP requests. It is similar to proxy-agent but is limited to HTTP protocol only, whereas proxy-agent supports multiple protocols.
This package provides an HTTPS agent to proxy HTTPS requests. Like http-proxy-agent, it is protocol-specific and does not offer the multi-protocol support that proxy-agent does.
This package is designed to provide an agent that proxies through a SOCKS proxy. It is specifically tailored for SOCKS protocol, unlike proxy-agent which handles SOCKS along with other protocols.
This package provides a proxy agent that can use a PAC file to determine the appropriate proxy for a given URL. It is focused on PAC file support, which is just one of the features available in proxy-agent.
http.Agent
implementationsThis module provides an http.Agent
implementation which automatically uses
proxy servers based off of the various proxy-related environment variables
(HTTP_PROXY
, HTTPS_PROXY
and NO_PROXY
among others).
Which proxy is used for each HTTP request is determined by the
proxy-from-env
module, so
check its documentation for instructions on configuring your environment variables.
An LRU cache is used so that http.Agent
instances are transparently re-used for
subsequent HTTP requests to the same proxy server.
The currently implemented protocol mappings are listed in the table below:
Protocol | Proxy Agent for http requests | Proxy Agent for https requests | Example |
---|---|---|---|
http | http-proxy-agent | https-proxy-agent | http://proxy-server-over-tcp.com:3128 |
https | http-proxy-agent | https-proxy-agent | https://proxy-server-over-tls.com:3129 |
socks(v5) | socks-proxy-agent | socks-proxy-agent | socks://username:password@some-socks-proxy.com:9050 (username & password are optional) |
socks5 | socks-proxy-agent | socks-proxy-agent | socks5://username:password@some-socks-proxy.com:9050 (username & password are optional) |
socks4 | socks-proxy-agent | socks-proxy-agent | socks4://some-socks-proxy.com:9050 |
pac-* | pac-proxy-agent | pac-proxy-agent | pac+http://www.example.com/proxy.pac |
import * as https from 'https';
import { ProxyAgent } from 'proxy-agent';
// The correct proxy `Agent` implementation to use will be determined
// via the `http_proxy` / `https_proxy` / `no_proxy` / etc. env vars
const agent = new ProxyAgent();
// The rest works just like any other normal HTTP request
https.get('https://jsonip.com', { agent }, (res) => {
console.log(res.statusCode, res.headers);
res.pipe(process.stdout);
});
Creates an http.Agent
instance which relies on the various proxy-related
environment variables. An LRU cache is used, so the same http.Agent
instance
will be returned if identical args are passed in.
FAQs
Maps proxy protocols to `http.Agent` implementations
The npm package proxy-agent receives a total of 0 weekly downloads. As such, proxy-agent popularity was classified as not popular.
We found that proxy-agent demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.