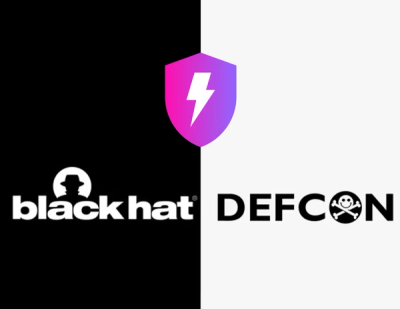
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
proxy-validator
Advanced tools
Small package that leverages the power of ES6 Proxy to validate and sanitize objects.
Small package that leverages the power of ES6 Proxy to validate and sanitize objects.
Learn More, read the article:
How I made a validation library using ES6 Proxy
$ npm i proxy-validator
The API is fairly simple. Create a validator by providing a validation schema and/or a sanitizing schema.
import ProxyValidator from 'proxy-validator';
// The schema props correspond to the props that will be validated.
const validators = {
// Define a set of rules to apply for each prop, each rule is formed by a key and a value.
name: {
// The key corresponds to a validator function.
isLength: {
// The value can be either an object, containing options and an errorMessage...
options: {
min: 6
},
errorMessage: 'Minimum length 6 characters.'
},
// ...or a boolean, in which case the message will be the default one.
isUppercase: true
},
mobile: {
isMobilePhone: {
options: 'en-GB',
errorMessage: 'Invalid mobile number.'
},
isAlphanumeric: true
}
};
const sanitizers = {
// As with the validation, the sanitizing schema is formed by a a key/value pair.
name: {
// The key corresponds with the sanitizing function
trim: true // and the value can be either boolean (use defaults)
},
email: {
normalizeEmail: {
options: { // ...or a config options object.
all_lowercase: true
}
}
}
};
// Creates a validator
const ContactValidator = ProxyValidator(validators, sanitizers);
// Creates a proxy that will enforce the validator rules.
const contact = ContactValidator();
contact.name = 'Mike'; // throws errors
contact.name = ' MICHAEL';
console.log(contact); // { name: 'MICHAEL' };
The validation is based on the amazing lib validator
by chriso. Find the complete list of available validators and sanitizers in here.
FAQs
Small package that leverages the power of ES6 Proxy to validate and sanitize objects.
The npm package proxy-validator receives a total of 3 weekly downloads. As such, proxy-validator popularity was classified as not popular.
We found that proxy-validator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.