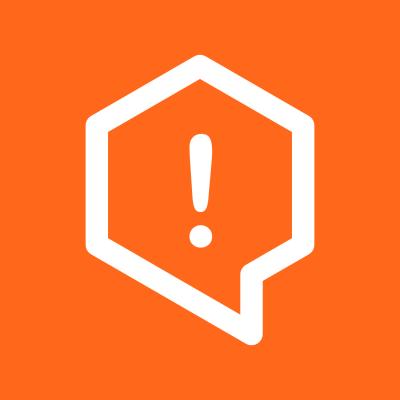
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
querystring
Advanced tools
The querystring npm package is used for parsing and formatting URL query strings. It provides utilities for working with the query string of a URL, such as parsing a query string into an object, stringifying an object into a query string, and handling escape and unescape of URL query strings.
Parsing a query string
This feature is used to parse a URL query string into an object where each key-value pair is represented as a property.
const querystring = require('querystring');
const parsed = querystring.parse('foo=bar&abc=xyz&abc=123');
Stringifying an object
This feature converts an object into a URL query string, with proper formatting and escaping.
const querystring = require('querystring');
const stringified = querystring.stringify({ foo: 'bar', baz: ['qux', 'quux'], corge: '' });
Escaping and unescaping
These functions are used to manually escape and unescape query string values.
const querystring = require('querystring');
const escaped = querystring.escape('foo@bar.com');
const unescaped = querystring.unescape('foo%40bar.com');
The 'qs' package is an advanced query string parser that supports nested objects and arrays, and offers more in-depth control over the parsing and stringifying of query strings compared to 'querystring'.
Node's querystring module for all engines.
If you want to help with evolution of this package, please see https://github.com/Gozala/querystring/issues/20 PR's welcome!
npm i querystring
Refer to Node's documentation for querystring
.
MIT © Gozala
FAQs
Node's querystring module for all engines.
We found that querystring demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.