queue-typed
Advanced tools
Comparing version
@@ -712,12 +712,10 @@ /** | ||
* | ||
* The indexOf function in TypeScript returns the index of a specified element or node in a Doubly | ||
* Linked List. | ||
* @param {E | DoublyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the | ||
* `indexOf` method can be either an element of type `E` or a `DoublyLinkedListNode` containing an | ||
* element of type `E`. | ||
* @returns The `indexOf` method is returning the index of the element or node in the doubly linked | ||
* list. If the element or node is found in the list, the method returns the index of that element or | ||
* node. If the element or node is not found in the list, the method returns -1. | ||
* This function finds the index of a specified element, node, or predicate in a doubly linked list. | ||
* @param {E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `indexOf` method takes in a parameter `elementNodeOrPredicate`, which | ||
* can be one of the following: | ||
* @returns The `indexOf` method returns the index of the element in the doubly linked list that | ||
* matches the provided element, node, or predicate. If no match is found, it returns -1. | ||
*/ | ||
indexOf(elementOrNode: E | DoublyLinkedListNode<E>): number; | ||
indexOf(elementNodeOrPredicate: E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)): number; | ||
/** | ||
@@ -727,4 +725,2 @@ * Time Complexity: O(n) | ||
* | ||
*/ | ||
/** | ||
* This function retrieves an element from a doubly linked list based on a given element | ||
@@ -827,2 +823,8 @@ * node or predicate. | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(1) | ||
* | ||
*/ | ||
countOccurrences(elementOrNode: E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)): number; | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
@@ -829,0 +831,0 @@ * |
@@ -787,9 +787,3 @@ "use strict"; | ||
addBefore(existingElementOrNode, newElementOrNode) { | ||
let existingNode; | ||
if (existingElementOrNode instanceof DoublyLinkedListNode) { | ||
existingNode = existingElementOrNode; | ||
} | ||
else { | ||
existingNode = this.getNode(existingElementOrNode); | ||
} | ||
const existingNode = this.getNode(existingElementOrNode); | ||
if (existingNode) { | ||
@@ -828,9 +822,3 @@ const newNode = this._ensureNode(newElementOrNode); | ||
addAfter(existingElementOrNode, newElementOrNode) { | ||
let existingNode; | ||
if (existingElementOrNode instanceof DoublyLinkedListNode) { | ||
existingNode = existingElementOrNode; | ||
} | ||
else { | ||
existingNode = this.getNode(existingElementOrNode); | ||
} | ||
const existingNode = this.getNode(existingElementOrNode); | ||
if (existingNode) { | ||
@@ -941,13 +929,11 @@ const newNode = this._ensureNode(newElementOrNode); | ||
* | ||
* The indexOf function in TypeScript returns the index of a specified element or node in a Doubly | ||
* Linked List. | ||
* @param {E | DoublyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the | ||
* `indexOf` method can be either an element of type `E` or a `DoublyLinkedListNode` containing an | ||
* element of type `E`. | ||
* @returns The `indexOf` method is returning the index of the element or node in the doubly linked | ||
* list. If the element or node is found in the list, the method returns the index of that element or | ||
* node. If the element or node is not found in the list, the method returns -1. | ||
* This function finds the index of a specified element, node, or predicate in a doubly linked list. | ||
* @param {E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `indexOf` method takes in a parameter `elementNodeOrPredicate`, which | ||
* can be one of the following: | ||
* @returns The `indexOf` method returns the index of the element in the doubly linked list that | ||
* matches the provided element, node, or predicate. If no match is found, it returns -1. | ||
*/ | ||
indexOf(elementOrNode) { | ||
const predicate = this._ensurePredicate(elementOrNode); | ||
indexOf(elementNodeOrPredicate) { | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let index = 0; | ||
@@ -968,4 +954,2 @@ let current = this.head; | ||
* | ||
*/ | ||
/** | ||
* This function retrieves an element from a doubly linked list based on a given element | ||
@@ -1131,2 +1115,19 @@ * node or predicate. | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(1) | ||
* | ||
*/ | ||
countOccurrences(elementOrNode) { | ||
const predicate = this._ensurePredicate(elementOrNode); | ||
let count = 0; | ||
let current = this.head; | ||
while (current) { | ||
if (predicate(current)) { | ||
count++; | ||
} | ||
current = current.next; | ||
} | ||
return count; | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
@@ -1133,0 +1134,0 @@ * |
@@ -76,21 +76,11 @@ /** | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
* | ||
* The `fromArray` function creates a new SinglyLinkedList instance and populates it with the elements from the given | ||
* array. | ||
* @param {E[]} data - The `data` parameter is an array of elements of type `E`. | ||
* @returns The `fromArray` function returns a `SinglyLinkedList` object. | ||
*/ | ||
static fromArray<E>(data: E[]): SinglyLinkedList<E, any>; | ||
/** | ||
* Time Complexity: O(1) | ||
* Space Complexity: O(1) | ||
* | ||
* The push function adds a new element to the end of a singly linked list. | ||
* @param {E} element - The "element" parameter represents the value of the element that you want to | ||
* add to the linked list. | ||
* @returns The `push` method is returning a boolean value, `true`. | ||
* The `push` function adds a new element or node to the end of a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the `push` | ||
* method can accept either an element of type `E` or a `SinglyLinkedListNode<E>` object. | ||
* @returns The `push` method is returning a boolean value, specifically `true`. | ||
*/ | ||
push(element: E): boolean; | ||
push(elementOrNode: E | SinglyLinkedListNode<E>): boolean; | ||
/** | ||
@@ -117,8 +107,10 @@ * Time Complexity: O(n) | ||
* | ||
* The unshift function adds a new element to the beginning of a singly linked list. | ||
* @param {E} element - The "element" parameter represents the value of the element that you want to | ||
* add to the beginning of the singly linked list. | ||
* @returns The `unshift` method is returning a boolean value, `true`. | ||
* The unshift function adds a new element or node to the beginning of a singly linked list in | ||
* TypeScript. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the | ||
* `unshift` method can be either an element of type `E` or a `SinglyLinkedListNode` containing an | ||
* element of type `E`. | ||
* @returns The `unshift` method is returning a boolean value, specifically `true`. | ||
*/ | ||
unshift(element: E): boolean; | ||
unshift(elementOrNode: E | SinglyLinkedListNode<E>): boolean; | ||
/** | ||
@@ -128,2 +120,15 @@ * Time Complexity: O(n) | ||
* | ||
* This function searches for a specific element in a singly linked list based on a given node or | ||
* predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `get` method can be one of | ||
* the following types: | ||
* @returns The `get` method returns the value of the first node in the singly linked list that | ||
* satisfies the provided predicate function. If no such node is found, it returns `undefined`. | ||
*/ | ||
get(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): E | undefined; | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(1) | ||
* | ||
* The function `at` returns the value at a specified index in a linked list, or undefined if the index is out of range. | ||
@@ -137,2 +142,16 @@ * @param {number} index - The index parameter is a number that represents the position of the element we want to | ||
/** | ||
* Time Complexity: O(1) | ||
* Space Complexity: O(1) | ||
* | ||
* The function `isNode` in TypeScript checks if the input is an instance of `SinglyLinkedListNode`. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `isNode` function can be | ||
* one of the following types: | ||
* @returns The `isNode` function is checking if the `elementNodeOrPredicate` parameter is an | ||
* instance of `SinglyLinkedListNode<E>`. If it is, the function returns `true`, indicating that the | ||
* parameter is a `SinglyLinkedListNode<E>`. If it is not an instance of `SinglyLinkedListNode<E>`, | ||
* the function returns `false`. | ||
*/ | ||
isNode(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): elementNodeOrPredicate is SinglyLinkedListNode<E>; | ||
/** | ||
* Time Complexity: O(n) | ||
@@ -164,3 +183,3 @@ * Space Complexity: O(1) | ||
* The delete function removes a node with a specific value from a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} valueOrNode - The `valueOrNode` parameter can accept either a value of type `E` | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter can accept either a value of type `E` | ||
* or a `SinglyLinkedListNode<E>` object. | ||
@@ -170,3 +189,3 @@ * @returns The `delete` method returns a boolean value. It returns `true` if the value or node is found and | ||
*/ | ||
delete(valueOrNode: E | SinglyLinkedListNode<E> | undefined): boolean; | ||
delete(elementOrNode: E | SinglyLinkedListNode<E> | undefined): boolean; | ||
/** | ||
@@ -176,11 +195,14 @@ * Time Complexity: O(n) | ||
* | ||
* The `addAt` function inserts a value at a specified index in a singly linked list. | ||
* @param {number} index - The index parameter represents the position at which the new value should be inserted in the | ||
* linked list. It is of type number. | ||
* @param {E} value - The `value` parameter represents the value that you want to insert into the linked list at the | ||
* specified index. | ||
* @returns The `insert` method returns a boolean value. It returns `true` if the insertion is successful, and `false` | ||
* if the index is out of bounds. | ||
* The `addAt` function inserts a new element or node at a specified index in a singly linked list. | ||
* @param {number} index - The `index` parameter represents the position at which you want to add a | ||
* new element or node in the linked list. It is a number that indicates the index where the new | ||
* element or node should be inserted. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addAt` method can be either a value of type `E` or a `SinglyLinkedListNode<E>` object. This | ||
* parameter represents the element or node that you want to add to the linked list at the specified | ||
* index. | ||
* @returns The `addAt` method returns a boolean value - `true` if the element or node was | ||
* successfully added at the specified index, and `false` if the index is out of bounds. | ||
*/ | ||
addAt(index: number, value: E): boolean; | ||
addAt(index: number, newElementOrNode: E | SinglyLinkedListNode<E>): boolean; | ||
/** | ||
@@ -216,8 +238,12 @@ * The function checks if the length of a data structure is equal to zero and returns a boolean value indicating | ||
* | ||
* The `indexOf` function returns the index of the first occurrence of a given value in a linked list. | ||
* @param {E} value - The value parameter is the value that you want to find the index of in the linked list. | ||
* @returns The method is returning the index of the first occurrence of the specified value in the linked list. If the | ||
* value is not found, it returns -1. | ||
* The `indexOf` function in TypeScript searches for a specific element or node in a singly linked | ||
* list and returns its index if found. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `indexOf` method can be one | ||
* of the following types: | ||
* @returns The `indexOf` method returns the index of the first occurrence of the element that | ||
* matches the provided predicate in the singly linked list. If no matching element is found, it | ||
* returns -1. | ||
*/ | ||
indexOf(value: E): number; | ||
indexOf(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): number; | ||
/** | ||
@@ -227,9 +253,12 @@ * Time Complexity: O(n) | ||
* | ||
* The function finds a node in a singly linked list by its value and returns the node if found, otherwise returns | ||
* undefined. | ||
* @param {E} value - The value parameter is the value that we want to search for in the linked list. | ||
* @returns a `SinglyLinkedListNode<E>` if a node with the specified value is found in the linked list. If no node with | ||
* the specified value is found, the function returns `undefined`. | ||
* The function `getNode` in TypeScript searches for a node in a singly linked list based on a given | ||
* element, node, or predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | undefined} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `getNode` method can be one | ||
* of the following types: | ||
* @returns The `getNode` method returns either a `SinglyLinkedListNode<E>` if a matching node is | ||
* found based on the provided predicate, or it returns `undefined` if no matching node is found or | ||
* if the input parameter is `undefined`. | ||
*/ | ||
getNode(value: E): SinglyLinkedListNode<E> | undefined; | ||
getNode(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | undefined): SinglyLinkedListNode<E> | undefined; | ||
/** | ||
@@ -239,10 +268,15 @@ * Time Complexity: O(n) | ||
* | ||
* The `addBefore` function inserts a new value before an existing value in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingValueOrNode - The existing value or node that you want to insert the | ||
* new value before. It can be either the value itself or a node containing the value in the linked list. | ||
* @param {E} newValue - The `newValue` parameter represents the value that you want to insert into the linked list. | ||
* @returns The method `addBefore` returns a boolean value. It returns `true` if the new value was successfully | ||
* inserted before the existing value, and `false` otherwise. | ||
* The function `addBefore` in TypeScript adds a new element or node before an existing element or | ||
* node in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingElementOrNode - existingElementOrNode represents the | ||
* element or node in the linked list before which you want to add a new element or node. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addBefore` method represents the element or node that you want to insert before the existing | ||
* element or node in the linked list. This new element can be of type `E` or a | ||
* `SinglyLinkedListNode<E>`. | ||
* @returns The `addBefore` method returns a boolean value - `true` if the new element or node was | ||
* successfully added before the existing element or node, and `false` if the operation was | ||
* unsuccessful. | ||
*/ | ||
addBefore(existingValueOrNode: E | SinglyLinkedListNode<E>, newValue: E): boolean; | ||
addBefore(existingElementOrNode: E | SinglyLinkedListNode<E>, newElementOrNode: E | SinglyLinkedListNode<E>): boolean; | ||
/** | ||
@@ -252,10 +286,15 @@ * Time Complexity: O(n) | ||
* | ||
* The `addAfter` function inserts a new node with a given value after an existing node in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingValueOrNode - The existing value or node in the linked list after which | ||
* the new value will be inserted. It can be either the value of the existing node or the existing node itself. | ||
* @param {E} newValue - The value that you want to insert into the linked list after the existing value or node. | ||
* @returns The method returns a boolean value. It returns true if the new value was successfully inserted after the | ||
* existing value or node, and false if the existing value or node was not found in the linked list. | ||
* The `addAfter` function in TypeScript adds a new element or node after an existing element or node | ||
* in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingElementOrNode - existingElementOrNode can be either | ||
* an element of type E or a SinglyLinkedListNode of type E. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addAfter` method represents the element or node that you want to add after the existing element | ||
* or node in a singly linked list. This parameter can be either the value of the new element or a | ||
* reference to a `SinglyLinkedListNode` containing | ||
* @returns The `addAfter` method returns a boolean value - `true` if the new element or node was | ||
* successfully added after the existing element or node, and `false` if the existing element or node | ||
* was not found. | ||
*/ | ||
addAfter(existingValueOrNode: E | SinglyLinkedListNode<E>, newValue: E): boolean; | ||
addAfter(existingElementOrNode: E | SinglyLinkedListNode<E>, newElementOrNode: E | SinglyLinkedListNode<E>): boolean; | ||
/** | ||
@@ -265,7 +304,10 @@ * Time Complexity: O(n) | ||
* | ||
* The function counts the number of occurrences of a given value in a linked list. | ||
* @param {E} value - The value parameter is the value that you want to count the occurrences of in the linked list. | ||
* @returns The count of occurrences of the given value in the linked list. | ||
* The function `countOccurrences` iterates through a singly linked list and counts the occurrences | ||
* of a specified element or nodes that satisfy a given predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementOrNode | ||
* - The `elementOrNode` parameter in the `countOccurrences` method can accept three types of values: | ||
* @returns The `countOccurrences` method returns the number of occurrences of the specified element, | ||
* node, or predicate function in the singly linked list. | ||
*/ | ||
countOccurrences(value: E): number; | ||
countOccurrences(elementOrNode: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): number; | ||
/** | ||
@@ -324,2 +366,42 @@ * Time Complexity: O(n) | ||
protected _getIterator(): IterableIterator<E>; | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
* | ||
* The `fromArray` function creates a new SinglyLinkedList instance and populates it with the elements from the given | ||
* array. | ||
* @param {E[]} data - The `data` parameter is an array of elements of type `E`. | ||
* @returns The `fromArray` function returns a `SinglyLinkedList` object. | ||
*/ | ||
static fromArray<E>(data: E[]): SinglyLinkedList<E, any>; | ||
/** | ||
* The _isPredicate function in TypeScript checks if the input is a function that takes a | ||
* SinglyLinkedListNode as an argument and returns a boolean. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter can be one of the following types: | ||
* @returns The _isPredicate method is returning a boolean value based on whether the | ||
* elementNodeOrPredicate parameter is a function or not. If the elementNodeOrPredicate is a | ||
* function, the method will return true, indicating that it is a predicate function. If it is not a | ||
* function, the method will return false. | ||
*/ | ||
protected _isPredicate(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): elementNodeOrPredicate is (node: SinglyLinkedListNode<E>) => boolean; | ||
/** | ||
* The function `_ensureNode` ensures that the input is a valid node and returns it, creating a new | ||
* node if necessary. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter can be either | ||
* an element of type `E` or a `SinglyLinkedListNode` containing an element of type `E`. | ||
* @returns A SinglyLinkedListNode<E> object is being returned. | ||
*/ | ||
protected _ensureNode(elementOrNode: E | SinglyLinkedListNode<E>): SinglyLinkedListNode<E>; | ||
/** | ||
* The function `_ensurePredicate` in TypeScript ensures that the input is either a node, a predicate | ||
* function, or a value to compare with the node's value. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter can be one of the following types: | ||
* @returns A function is being returned. If the input `elementNodeOrPredicate` is already a node, a | ||
* function is returned that checks if a given node is equal to the input node. If the input is a | ||
* predicate function, it is returned as is. If the input is neither a node nor a predicate function, | ||
* a function is returned that checks if a given node's value is equal to the input | ||
*/ | ||
protected _ensurePredicate(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): (node: SinglyLinkedListNode<E>) => boolean; | ||
} |
@@ -103,28 +103,12 @@ "use strict"; | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
* | ||
* The `fromArray` function creates a new SinglyLinkedList instance and populates it with the elements from the given | ||
* array. | ||
* @param {E[]} data - The `data` parameter is an array of elements of type `E`. | ||
* @returns The `fromArray` function returns a `SinglyLinkedList` object. | ||
*/ | ||
static fromArray(data) { | ||
const singlyLinkedList = new SinglyLinkedList(); | ||
for (const item of data) { | ||
singlyLinkedList.push(item); | ||
} | ||
return singlyLinkedList; | ||
} | ||
/** | ||
* Time Complexity: O(1) | ||
* Space Complexity: O(1) | ||
* | ||
* The push function adds a new element to the end of a singly linked list. | ||
* @param {E} element - The "element" parameter represents the value of the element that you want to | ||
* add to the linked list. | ||
* @returns The `push` method is returning a boolean value, `true`. | ||
* The `push` function adds a new element or node to the end of a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the `push` | ||
* method can accept either an element of type `E` or a `SinglyLinkedListNode<E>` object. | ||
* @returns The `push` method is returning a boolean value, specifically `true`. | ||
*/ | ||
push(element) { | ||
const newNode = new SinglyLinkedListNode(element); | ||
push(elementOrNode) { | ||
const newNode = this._ensureNode(elementOrNode); | ||
if (!this.head) { | ||
@@ -188,9 +172,11 @@ this._head = newNode; | ||
* | ||
* The unshift function adds a new element to the beginning of a singly linked list. | ||
* @param {E} element - The "element" parameter represents the value of the element that you want to | ||
* add to the beginning of the singly linked list. | ||
* @returns The `unshift` method is returning a boolean value, `true`. | ||
* The unshift function adds a new element or node to the beginning of a singly linked list in | ||
* TypeScript. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the | ||
* `unshift` method can be either an element of type `E` or a `SinglyLinkedListNode` containing an | ||
* element of type `E`. | ||
* @returns The `unshift` method is returning a boolean value, specifically `true`. | ||
*/ | ||
unshift(element) { | ||
const newNode = new SinglyLinkedListNode(element); | ||
unshift(elementOrNode) { | ||
const newNode = this._ensureNode(elementOrNode); | ||
if (!this.head) { | ||
@@ -211,2 +197,24 @@ this._head = newNode; | ||
* | ||
* This function searches for a specific element in a singly linked list based on a given node or | ||
* predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `get` method can be one of | ||
* the following types: | ||
* @returns The `get` method returns the value of the first node in the singly linked list that | ||
* satisfies the provided predicate function. If no such node is found, it returns `undefined`. | ||
*/ | ||
get(elementNodeOrPredicate) { | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let current = this.head; | ||
while (current) { | ||
if (predicate(current)) | ||
return current.value; | ||
current = current.next; | ||
} | ||
return undefined; | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(1) | ||
* | ||
* The function `at` returns the value at a specified index in a linked list, or undefined if the index is out of range. | ||
@@ -228,2 +236,18 @@ * @param {number} index - The index parameter is a number that represents the position of the element we want to | ||
/** | ||
* Time Complexity: O(1) | ||
* Space Complexity: O(1) | ||
* | ||
* The function `isNode` in TypeScript checks if the input is an instance of `SinglyLinkedListNode`. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `isNode` function can be | ||
* one of the following types: | ||
* @returns The `isNode` function is checking if the `elementNodeOrPredicate` parameter is an | ||
* instance of `SinglyLinkedListNode<E>`. If it is, the function returns `true`, indicating that the | ||
* parameter is a `SinglyLinkedListNode<E>`. If it is not an instance of `SinglyLinkedListNode<E>`, | ||
* the function returns `false`. | ||
*/ | ||
isNode(elementNodeOrPredicate) { | ||
return elementNodeOrPredicate instanceof SinglyLinkedListNode; | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
@@ -277,3 +301,3 @@ * Space Complexity: O(1) | ||
* The delete function removes a node with a specific value from a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} valueOrNode - The `valueOrNode` parameter can accept either a value of type `E` | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter can accept either a value of type `E` | ||
* or a `SinglyLinkedListNode<E>` object. | ||
@@ -283,11 +307,11 @@ * @returns The `delete` method returns a boolean value. It returns `true` if the value or node is found and | ||
*/ | ||
delete(valueOrNode) { | ||
if (valueOrNode === undefined) | ||
delete(elementOrNode) { | ||
if (elementOrNode === undefined) | ||
return false; | ||
let value; | ||
if (valueOrNode instanceof SinglyLinkedListNode) { | ||
value = valueOrNode.value; | ||
if (elementOrNode instanceof SinglyLinkedListNode) { | ||
value = elementOrNode.value; | ||
} | ||
else { | ||
value = valueOrNode; | ||
value = elementOrNode; | ||
} | ||
@@ -321,22 +345,25 @@ let current = this.head, prev = undefined; | ||
* | ||
* The `addAt` function inserts a value at a specified index in a singly linked list. | ||
* @param {number} index - The index parameter represents the position at which the new value should be inserted in the | ||
* linked list. It is of type number. | ||
* @param {E} value - The `value` parameter represents the value that you want to insert into the linked list at the | ||
* specified index. | ||
* @returns The `insert` method returns a boolean value. It returns `true` if the insertion is successful, and `false` | ||
* if the index is out of bounds. | ||
* The `addAt` function inserts a new element or node at a specified index in a singly linked list. | ||
* @param {number} index - The `index` parameter represents the position at which you want to add a | ||
* new element or node in the linked list. It is a number that indicates the index where the new | ||
* element or node should be inserted. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addAt` method can be either a value of type `E` or a `SinglyLinkedListNode<E>` object. This | ||
* parameter represents the element or node that you want to add to the linked list at the specified | ||
* index. | ||
* @returns The `addAt` method returns a boolean value - `true` if the element or node was | ||
* successfully added at the specified index, and `false` if the index is out of bounds. | ||
*/ | ||
addAt(index, value) { | ||
addAt(index, newElementOrNode) { | ||
if (index < 0 || index > this._size) | ||
return false; | ||
if (index === 0) { | ||
this.unshift(value); | ||
this.unshift(newElementOrNode); | ||
return true; | ||
} | ||
if (index === this._size) { | ||
this.push(value); | ||
this.push(newElementOrNode); | ||
return true; | ||
} | ||
const newNode = new SinglyLinkedListNode(value); | ||
const newNode = this._ensureNode(newElementOrNode); | ||
const prevNode = this.getNodeAt(index - 1); | ||
@@ -406,12 +433,17 @@ newNode.next = prevNode.next; | ||
* | ||
* The `indexOf` function returns the index of the first occurrence of a given value in a linked list. | ||
* @param {E} value - The value parameter is the value that you want to find the index of in the linked list. | ||
* @returns The method is returning the index of the first occurrence of the specified value in the linked list. If the | ||
* value is not found, it returns -1. | ||
* The `indexOf` function in TypeScript searches for a specific element or node in a singly linked | ||
* list and returns its index if found. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `indexOf` method can be one | ||
* of the following types: | ||
* @returns The `indexOf` method returns the index of the first occurrence of the element that | ||
* matches the provided predicate in the singly linked list. If no matching element is found, it | ||
* returns -1. | ||
*/ | ||
indexOf(value) { | ||
indexOf(elementNodeOrPredicate) { | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let index = 0; | ||
let current = this.head; | ||
while (current) { | ||
if (current.value === value) { | ||
if (predicate(current)) { | ||
return index; | ||
@@ -428,12 +460,18 @@ } | ||
* | ||
* The function finds a node in a singly linked list by its value and returns the node if found, otherwise returns | ||
* undefined. | ||
* @param {E} value - The value parameter is the value that we want to search for in the linked list. | ||
* @returns a `SinglyLinkedListNode<E>` if a node with the specified value is found in the linked list. If no node with | ||
* the specified value is found, the function returns `undefined`. | ||
* The function `getNode` in TypeScript searches for a node in a singly linked list based on a given | ||
* element, node, or predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | undefined} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `getNode` method can be one | ||
* of the following types: | ||
* @returns The `getNode` method returns either a `SinglyLinkedListNode<E>` if a matching node is | ||
* found based on the provided predicate, or it returns `undefined` if no matching node is found or | ||
* if the input parameter is `undefined`. | ||
*/ | ||
getNode(value) { | ||
getNode(elementNodeOrPredicate) { | ||
if (elementNodeOrPredicate === undefined) | ||
return; | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let current = this.head; | ||
while (current) { | ||
if (current.value === value) { | ||
if (predicate(current)) { | ||
return current; | ||
@@ -449,21 +487,26 @@ } | ||
* | ||
* The `addBefore` function inserts a new value before an existing value in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingValueOrNode - The existing value or node that you want to insert the | ||
* new value before. It can be either the value itself or a node containing the value in the linked list. | ||
* @param {E} newValue - The `newValue` parameter represents the value that you want to insert into the linked list. | ||
* @returns The method `addBefore` returns a boolean value. It returns `true` if the new value was successfully | ||
* inserted before the existing value, and `false` otherwise. | ||
* The function `addBefore` in TypeScript adds a new element or node before an existing element or | ||
* node in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingElementOrNode - existingElementOrNode represents the | ||
* element or node in the linked list before which you want to add a new element or node. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addBefore` method represents the element or node that you want to insert before the existing | ||
* element or node in the linked list. This new element can be of type `E` or a | ||
* `SinglyLinkedListNode<E>`. | ||
* @returns The `addBefore` method returns a boolean value - `true` if the new element or node was | ||
* successfully added before the existing element or node, and `false` if the operation was | ||
* unsuccessful. | ||
*/ | ||
addBefore(existingValueOrNode, newValue) { | ||
addBefore(existingElementOrNode, newElementOrNode) { | ||
if (!this.head) | ||
return false; | ||
let existingValue; | ||
if (existingValueOrNode instanceof SinglyLinkedListNode) { | ||
existingValue = existingValueOrNode.value; | ||
if (this.isNode(existingElementOrNode)) { | ||
existingValue = existingElementOrNode.value; | ||
} | ||
else { | ||
existingValue = existingValueOrNode; | ||
existingValue = existingElementOrNode; | ||
} | ||
if (this.head.value === existingValue) { | ||
this.unshift(newValue); | ||
this.unshift(newElementOrNode); | ||
return true; | ||
@@ -474,3 +517,3 @@ } | ||
if (current.next.value === existingValue) { | ||
const newNode = new SinglyLinkedListNode(newValue); | ||
const newNode = this._ensureNode(newElementOrNode); | ||
newNode.next = current.next; | ||
@@ -489,19 +532,18 @@ current.next = newNode; | ||
* | ||
* The `addAfter` function inserts a new node with a given value after an existing node in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingValueOrNode - The existing value or node in the linked list after which | ||
* the new value will be inserted. It can be either the value of the existing node or the existing node itself. | ||
* @param {E} newValue - The value that you want to insert into the linked list after the existing value or node. | ||
* @returns The method returns a boolean value. It returns true if the new value was successfully inserted after the | ||
* existing value or node, and false if the existing value or node was not found in the linked list. | ||
* The `addAfter` function in TypeScript adds a new element or node after an existing element or node | ||
* in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingElementOrNode - existingElementOrNode can be either | ||
* an element of type E or a SinglyLinkedListNode of type E. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addAfter` method represents the element or node that you want to add after the existing element | ||
* or node in a singly linked list. This parameter can be either the value of the new element or a | ||
* reference to a `SinglyLinkedListNode` containing | ||
* @returns The `addAfter` method returns a boolean value - `true` if the new element or node was | ||
* successfully added after the existing element or node, and `false` if the existing element or node | ||
* was not found. | ||
*/ | ||
addAfter(existingValueOrNode, newValue) { | ||
let existingNode; | ||
if (existingValueOrNode instanceof SinglyLinkedListNode) { | ||
existingNode = existingValueOrNode; | ||
} | ||
else { | ||
existingNode = this.getNode(existingValueOrNode); | ||
} | ||
addAfter(existingElementOrNode, newElementOrNode) { | ||
const existingNode = this.getNode(existingElementOrNode); | ||
if (existingNode) { | ||
const newNode = new SinglyLinkedListNode(newValue); | ||
const newNode = this._ensureNode(newElementOrNode); | ||
newNode.next = existingNode.next; | ||
@@ -521,11 +563,15 @@ existingNode.next = newNode; | ||
* | ||
* The function counts the number of occurrences of a given value in a linked list. | ||
* @param {E} value - The value parameter is the value that you want to count the occurrences of in the linked list. | ||
* @returns The count of occurrences of the given value in the linked list. | ||
* The function `countOccurrences` iterates through a singly linked list and counts the occurrences | ||
* of a specified element or nodes that satisfy a given predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementOrNode | ||
* - The `elementOrNode` parameter in the `countOccurrences` method can accept three types of values: | ||
* @returns The `countOccurrences` method returns the number of occurrences of the specified element, | ||
* node, or predicate function in the singly linked list. | ||
*/ | ||
countOccurrences(value) { | ||
countOccurrences(elementOrNode) { | ||
const predicate = this._ensurePredicate(elementOrNode); | ||
let count = 0; | ||
let current = this.head; | ||
while (current) { | ||
if (current.value === value) { | ||
if (predicate(current)) { | ||
count++; | ||
@@ -616,3 +662,61 @@ } | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
* | ||
* The `fromArray` function creates a new SinglyLinkedList instance and populates it with the elements from the given | ||
* array. | ||
* @param {E[]} data - The `data` parameter is an array of elements of type `E`. | ||
* @returns The `fromArray` function returns a `SinglyLinkedList` object. | ||
*/ | ||
static fromArray(data) { | ||
const singlyLinkedList = new SinglyLinkedList(); | ||
for (const item of data) { | ||
singlyLinkedList.push(item); | ||
} | ||
return singlyLinkedList; | ||
} | ||
/** | ||
* The _isPredicate function in TypeScript checks if the input is a function that takes a | ||
* SinglyLinkedListNode as an argument and returns a boolean. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter can be one of the following types: | ||
* @returns The _isPredicate method is returning a boolean value based on whether the | ||
* elementNodeOrPredicate parameter is a function or not. If the elementNodeOrPredicate is a | ||
* function, the method will return true, indicating that it is a predicate function. If it is not a | ||
* function, the method will return false. | ||
*/ | ||
_isPredicate(elementNodeOrPredicate) { | ||
return typeof elementNodeOrPredicate === 'function'; | ||
} | ||
/** | ||
* The function `_ensureNode` ensures that the input is a valid node and returns it, creating a new | ||
* node if necessary. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter can be either | ||
* an element of type `E` or a `SinglyLinkedListNode` containing an element of type `E`. | ||
* @returns A SinglyLinkedListNode<E> object is being returned. | ||
*/ | ||
_ensureNode(elementOrNode) { | ||
if (this.isNode(elementOrNode)) | ||
return elementOrNode; | ||
return new SinglyLinkedListNode(elementOrNode); | ||
} | ||
/** | ||
* The function `_ensurePredicate` in TypeScript ensures that the input is either a node, a predicate | ||
* function, or a value to compare with the node's value. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter can be one of the following types: | ||
* @returns A function is being returned. If the input `elementNodeOrPredicate` is already a node, a | ||
* function is returned that checks if a given node is equal to the input node. If the input is a | ||
* predicate function, it is returned as is. If the input is neither a node nor a predicate function, | ||
* a function is returned that checks if a given node's value is equal to the input | ||
*/ | ||
_ensurePredicate(elementNodeOrPredicate) { | ||
if (this.isNode(elementNodeOrPredicate)) | ||
return (node) => node === elementNodeOrPredicate; | ||
if (this._isPredicate(elementNodeOrPredicate)) | ||
return elementNodeOrPredicate; | ||
return (node) => node.value === elementNodeOrPredicate; | ||
} | ||
} | ||
exports.SinglyLinkedList = SinglyLinkedList; |
{ | ||
"name": "queue-typed", | ||
"version": "1.53.5", | ||
"version": "1.53.6", | ||
"description": "Queue, ArrayQueue. Javascript & Typescript Data Structure.", | ||
@@ -118,4 +118,4 @@ "main": "dist/index.js", | ||
"dependencies": { | ||
"data-structure-typed": "^1.53.5" | ||
"data-structure-typed": "^1.53.6" | ||
} | ||
} |
@@ -55,9 +55,2 @@  | ||
### methods | ||
Queue | ||
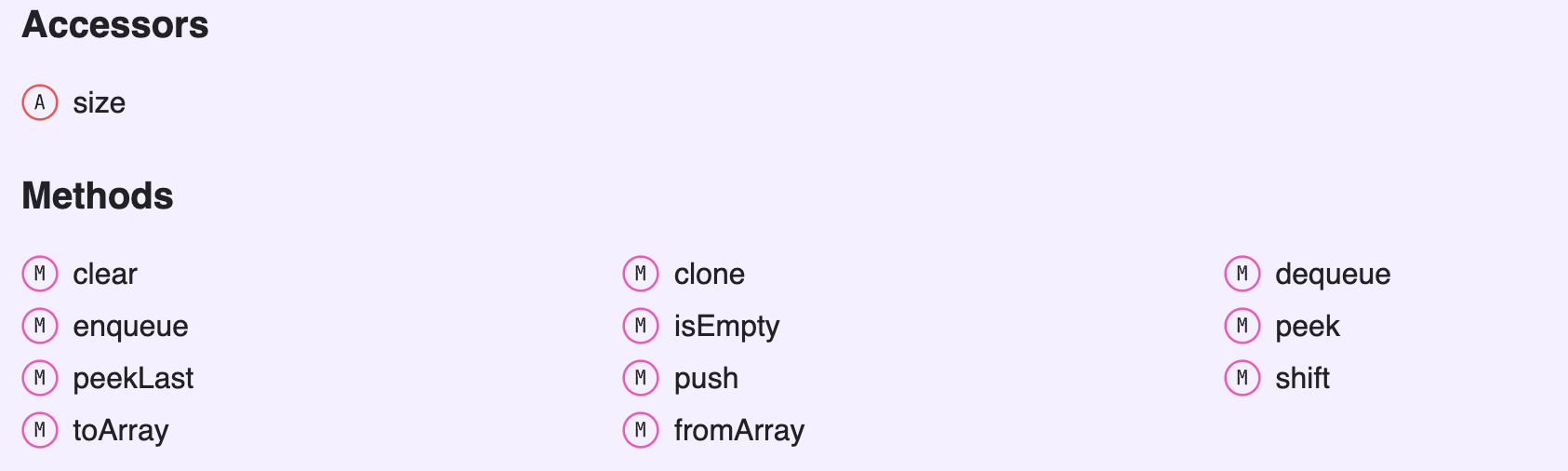 | ||
LinkedListQueue | ||
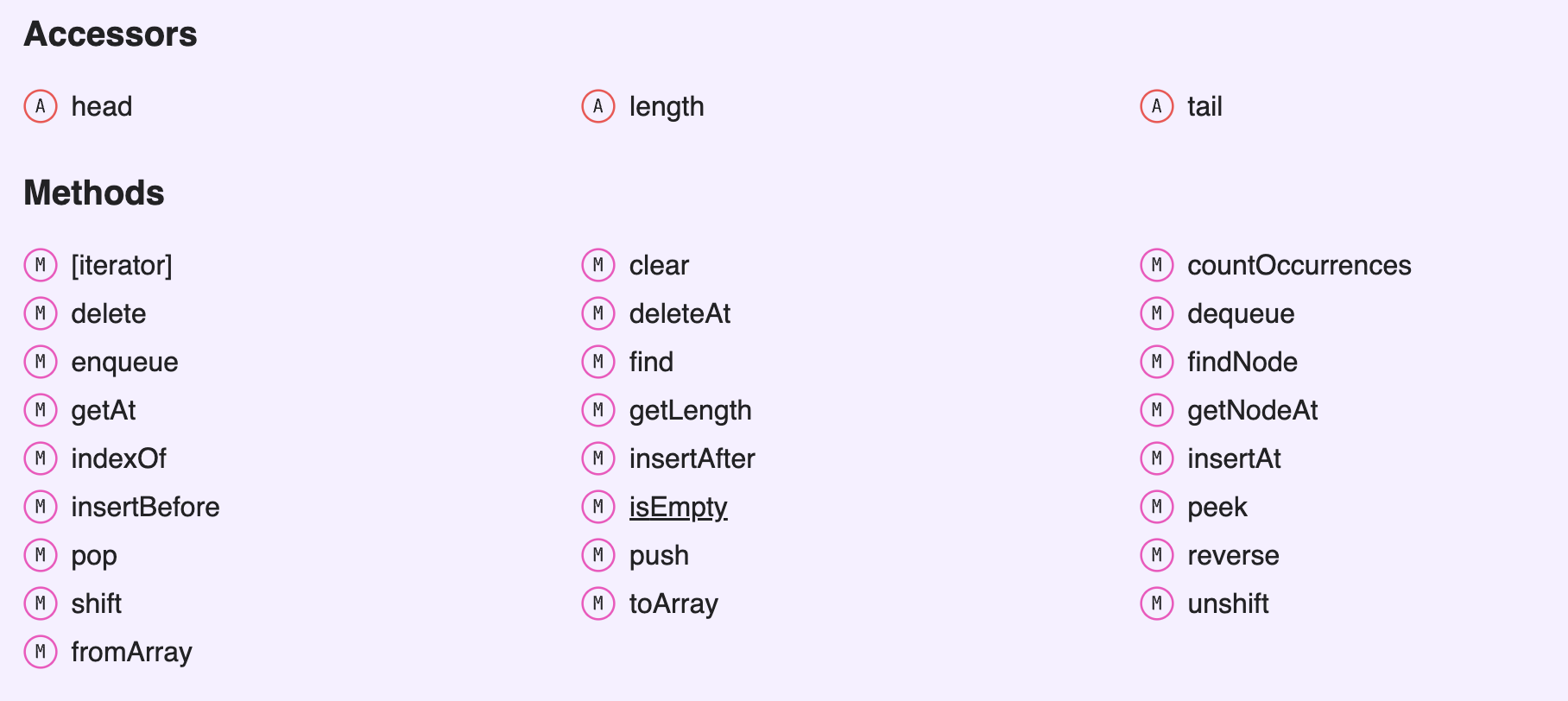 | ||
### snippet | ||
@@ -103,3 +96,8 @@ | ||
[//]: # (No deletion!!! Start of Example Replace Section) | ||
[//]: # (No deletion!!! End of Example Replace Section) | ||
## API docs & Examples | ||
@@ -106,0 +104,0 @@ |
@@ -822,10 +822,4 @@ /** | ||
): boolean { | ||
let existingNode; | ||
const existingNode: DoublyLinkedListNode<E> | undefined = this.getNode(existingElementOrNode); | ||
if (existingElementOrNode instanceof DoublyLinkedListNode) { | ||
existingNode = existingElementOrNode; | ||
} else { | ||
existingNode = this.getNode(existingElementOrNode); | ||
} | ||
if (existingNode) { | ||
@@ -866,10 +860,4 @@ const newNode = this._ensureNode(newElementOrNode); | ||
addAfter(existingElementOrNode: E | DoublyLinkedListNode<E>, newElementOrNode: E | DoublyLinkedListNode<E>): boolean { | ||
let existingNode; | ||
const existingNode: DoublyLinkedListNode<E> | undefined = this.getNode(existingElementOrNode); | ||
if (existingElementOrNode instanceof DoublyLinkedListNode) { | ||
existingNode = existingElementOrNode; | ||
} else { | ||
existingNode = this.getNode(existingElementOrNode); | ||
} | ||
if (existingNode) { | ||
@@ -983,13 +971,11 @@ const newNode = this._ensureNode(newElementOrNode); | ||
* | ||
* The indexOf function in TypeScript returns the index of a specified element or node in a Doubly | ||
* Linked List. | ||
* @param {E | DoublyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the | ||
* `indexOf` method can be either an element of type `E` or a `DoublyLinkedListNode` containing an | ||
* element of type `E`. | ||
* @returns The `indexOf` method is returning the index of the element or node in the doubly linked | ||
* list. If the element or node is found in the list, the method returns the index of that element or | ||
* node. If the element or node is not found in the list, the method returns -1. | ||
* This function finds the index of a specified element, node, or predicate in a doubly linked list. | ||
* @param {E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `indexOf` method takes in a parameter `elementNodeOrPredicate`, which | ||
* can be one of the following: | ||
* @returns The `indexOf` method returns the index of the element in the doubly linked list that | ||
* matches the provided element, node, or predicate. If no match is found, it returns -1. | ||
*/ | ||
indexOf(elementOrNode: E | DoublyLinkedListNode<E>): number { | ||
const predicate = this._ensurePredicate(elementOrNode); | ||
indexOf(elementNodeOrPredicate: E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)): number { | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let index = 0; | ||
@@ -1011,4 +997,2 @@ let current = this.head; | ||
* | ||
*/ | ||
/** | ||
* This function retrieves an element from a doubly linked list based on a given element | ||
@@ -1189,2 +1173,22 @@ * node or predicate. | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(1) | ||
* | ||
*/ | ||
countOccurrences(elementOrNode: E | DoublyLinkedListNode<E> | ((node: DoublyLinkedListNode<E>) => boolean)): number { | ||
const predicate = this._ensurePredicate(elementOrNode); | ||
let count = 0; | ||
let current = this.head; | ||
while (current) { | ||
if (predicate(current)) { | ||
count++; | ||
} | ||
current = current.next; | ||
} | ||
return count; | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
@@ -1191,0 +1195,0 @@ * |
@@ -125,29 +125,12 @@ /** | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
* | ||
* The `fromArray` function creates a new SinglyLinkedList instance and populates it with the elements from the given | ||
* array. | ||
* @param {E[]} data - The `data` parameter is an array of elements of type `E`. | ||
* @returns The `fromArray` function returns a `SinglyLinkedList` object. | ||
*/ | ||
static fromArray<E>(data: E[]) { | ||
const singlyLinkedList = new SinglyLinkedList<E>(); | ||
for (const item of data) { | ||
singlyLinkedList.push(item); | ||
} | ||
return singlyLinkedList; | ||
} | ||
/** | ||
* Time Complexity: O(1) | ||
* Space Complexity: O(1) | ||
* | ||
* The push function adds a new element to the end of a singly linked list. | ||
* @param {E} element - The "element" parameter represents the value of the element that you want to | ||
* add to the linked list. | ||
* @returns The `push` method is returning a boolean value, `true`. | ||
* The `push` function adds a new element or node to the end of a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the `push` | ||
* method can accept either an element of type `E` or a `SinglyLinkedListNode<E>` object. | ||
* @returns The `push` method is returning a boolean value, specifically `true`. | ||
*/ | ||
push(element: E): boolean { | ||
const newNode = new SinglyLinkedListNode(element); | ||
push(elementOrNode: E | SinglyLinkedListNode<E>): boolean { | ||
const newNode = this._ensureNode(elementOrNode); | ||
if (!this.head) { | ||
@@ -212,9 +195,11 @@ this._head = newNode; | ||
* | ||
* The unshift function adds a new element to the beginning of a singly linked list. | ||
* @param {E} element - The "element" parameter represents the value of the element that you want to | ||
* add to the beginning of the singly linked list. | ||
* @returns The `unshift` method is returning a boolean value, `true`. | ||
* The unshift function adds a new element or node to the beginning of a singly linked list in | ||
* TypeScript. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter in the | ||
* `unshift` method can be either an element of type `E` or a `SinglyLinkedListNode` containing an | ||
* element of type `E`. | ||
* @returns The `unshift` method is returning a boolean value, specifically `true`. | ||
*/ | ||
unshift(element: E): boolean { | ||
const newNode = new SinglyLinkedListNode(element); | ||
unshift(elementOrNode: E | SinglyLinkedListNode<E>): boolean { | ||
const newNode = this._ensureNode(elementOrNode); | ||
if (!this.head) { | ||
@@ -235,2 +220,26 @@ this._head = newNode; | ||
* | ||
* This function searches for a specific element in a singly linked list based on a given node or | ||
* predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `get` method can be one of | ||
* the following types: | ||
* @returns The `get` method returns the value of the first node in the singly linked list that | ||
* satisfies the provided predicate function. If no such node is found, it returns `undefined`. | ||
*/ | ||
get( | ||
elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | ||
): E | undefined { | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let current = this.head; | ||
while (current) { | ||
if (predicate(current)) return current.value; | ||
current = current.next; | ||
} | ||
return undefined; | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(1) | ||
* | ||
* The function `at` returns the value at a specified index in a linked list, or undefined if the index is out of range. | ||
@@ -252,2 +261,21 @@ * @param {number} index - The index parameter is a number that represents the position of the element we want to | ||
/** | ||
* Time Complexity: O(1) | ||
* Space Complexity: O(1) | ||
* | ||
* The function `isNode` in TypeScript checks if the input is an instance of `SinglyLinkedListNode`. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `isNode` function can be | ||
* one of the following types: | ||
* @returns The `isNode` function is checking if the `elementNodeOrPredicate` parameter is an | ||
* instance of `SinglyLinkedListNode<E>`. If it is, the function returns `true`, indicating that the | ||
* parameter is a `SinglyLinkedListNode<E>`. If it is not an instance of `SinglyLinkedListNode<E>`, | ||
* the function returns `false`. | ||
*/ | ||
isNode( | ||
elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | ||
): elementNodeOrPredicate is SinglyLinkedListNode<E> { | ||
return elementNodeOrPredicate instanceof SinglyLinkedListNode; | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
@@ -303,3 +331,3 @@ * Space Complexity: O(1) | ||
* The delete function removes a node with a specific value from a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} valueOrNode - The `valueOrNode` parameter can accept either a value of type `E` | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter can accept either a value of type `E` | ||
* or a `SinglyLinkedListNode<E>` object. | ||
@@ -309,9 +337,9 @@ * @returns The `delete` method returns a boolean value. It returns `true` if the value or node is found and | ||
*/ | ||
delete(valueOrNode: E | SinglyLinkedListNode<E> | undefined): boolean { | ||
if (valueOrNode === undefined) return false; | ||
delete(elementOrNode: E | SinglyLinkedListNode<E> | undefined): boolean { | ||
if (elementOrNode === undefined) return false; | ||
let value: E; | ||
if (valueOrNode instanceof SinglyLinkedListNode) { | ||
value = valueOrNode.value; | ||
if (elementOrNode instanceof SinglyLinkedListNode) { | ||
value = elementOrNode.value; | ||
} else { | ||
value = valueOrNode; | ||
value = elementOrNode; | ||
} | ||
@@ -348,22 +376,26 @@ let current = this.head, | ||
* | ||
* The `addAt` function inserts a value at a specified index in a singly linked list. | ||
* @param {number} index - The index parameter represents the position at which the new value should be inserted in the | ||
* linked list. It is of type number. | ||
* @param {E} value - The `value` parameter represents the value that you want to insert into the linked list at the | ||
* specified index. | ||
* @returns The `insert` method returns a boolean value. It returns `true` if the insertion is successful, and `false` | ||
* if the index is out of bounds. | ||
* The `addAt` function inserts a new element or node at a specified index in a singly linked list. | ||
* @param {number} index - The `index` parameter represents the position at which you want to add a | ||
* new element or node in the linked list. It is a number that indicates the index where the new | ||
* element or node should be inserted. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addAt` method can be either a value of type `E` or a `SinglyLinkedListNode<E>` object. This | ||
* parameter represents the element or node that you want to add to the linked list at the specified | ||
* index. | ||
* @returns The `addAt` method returns a boolean value - `true` if the element or node was | ||
* successfully added at the specified index, and `false` if the index is out of bounds. | ||
*/ | ||
addAt(index: number, value: E): boolean { | ||
addAt(index: number, newElementOrNode: E | SinglyLinkedListNode<E>): boolean { | ||
if (index < 0 || index > this._size) return false; | ||
if (index === 0) { | ||
this.unshift(value); | ||
this.unshift(newElementOrNode); | ||
return true; | ||
} | ||
if (index === this._size) { | ||
this.push(value); | ||
this.push(newElementOrNode); | ||
return true; | ||
} | ||
const newNode = new SinglyLinkedListNode(value); | ||
const newNode = this._ensureNode(newElementOrNode); | ||
const prevNode = this.getNodeAt(index - 1); | ||
@@ -440,8 +472,13 @@ newNode.next = prevNode!.next; | ||
* | ||
* The `indexOf` function returns the index of the first occurrence of a given value in a linked list. | ||
* @param {E} value - The value parameter is the value that you want to find the index of in the linked list. | ||
* @returns The method is returning the index of the first occurrence of the specified value in the linked list. If the | ||
* value is not found, it returns -1. | ||
* The `indexOf` function in TypeScript searches for a specific element or node in a singly linked | ||
* list and returns its index if found. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `indexOf` method can be one | ||
* of the following types: | ||
* @returns The `indexOf` method returns the index of the first occurrence of the element that | ||
* matches the provided predicate in the singly linked list. If no matching element is found, it | ||
* returns -1. | ||
*/ | ||
indexOf(value: E): number { | ||
indexOf(elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): number { | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let index = 0; | ||
@@ -451,3 +488,3 @@ let current = this.head; | ||
while (current) { | ||
if (current.value === value) { | ||
if (predicate(current)) { | ||
return index; | ||
@@ -466,13 +503,20 @@ } | ||
* | ||
* The function finds a node in a singly linked list by its value and returns the node if found, otherwise returns | ||
* undefined. | ||
* @param {E} value - The value parameter is the value that we want to search for in the linked list. | ||
* @returns a `SinglyLinkedListNode<E>` if a node with the specified value is found in the linked list. If no node with | ||
* the specified value is found, the function returns `undefined`. | ||
* The function `getNode` in TypeScript searches for a node in a singly linked list based on a given | ||
* element, node, or predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | undefined} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter in the `getNode` method can be one | ||
* of the following types: | ||
* @returns The `getNode` method returns either a `SinglyLinkedListNode<E>` if a matching node is | ||
* found based on the provided predicate, or it returns `undefined` if no matching node is found or | ||
* if the input parameter is `undefined`. | ||
*/ | ||
getNode(value: E): SinglyLinkedListNode<E> | undefined { | ||
getNode( | ||
elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | undefined | ||
): SinglyLinkedListNode<E> | undefined { | ||
if (elementNodeOrPredicate === undefined) return; | ||
const predicate = this._ensurePredicate(elementNodeOrPredicate); | ||
let current = this.head; | ||
while (current) { | ||
if (current.value === value) { | ||
if (predicate(current)) { | ||
return current; | ||
@@ -490,20 +534,28 @@ } | ||
* | ||
* The `addBefore` function inserts a new value before an existing value in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingValueOrNode - The existing value or node that you want to insert the | ||
* new value before. It can be either the value itself or a node containing the value in the linked list. | ||
* @param {E} newValue - The `newValue` parameter represents the value that you want to insert into the linked list. | ||
* @returns The method `addBefore` returns a boolean value. It returns `true` if the new value was successfully | ||
* inserted before the existing value, and `false` otherwise. | ||
* The function `addBefore` in TypeScript adds a new element or node before an existing element or | ||
* node in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingElementOrNode - existingElementOrNode represents the | ||
* element or node in the linked list before which you want to add a new element or node. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addBefore` method represents the element or node that you want to insert before the existing | ||
* element or node in the linked list. This new element can be of type `E` or a | ||
* `SinglyLinkedListNode<E>`. | ||
* @returns The `addBefore` method returns a boolean value - `true` if the new element or node was | ||
* successfully added before the existing element or node, and `false` if the operation was | ||
* unsuccessful. | ||
*/ | ||
addBefore(existingValueOrNode: E | SinglyLinkedListNode<E>, newValue: E): boolean { | ||
addBefore( | ||
existingElementOrNode: E | SinglyLinkedListNode<E>, | ||
newElementOrNode: E | SinglyLinkedListNode<E> | ||
): boolean { | ||
if (!this.head) return false; | ||
let existingValue: E; | ||
if (existingValueOrNode instanceof SinglyLinkedListNode) { | ||
existingValue = existingValueOrNode.value; | ||
if (this.isNode(existingElementOrNode)) { | ||
existingValue = existingElementOrNode.value; | ||
} else { | ||
existingValue = existingValueOrNode; | ||
existingValue = existingElementOrNode; | ||
} | ||
if (this.head.value === existingValue) { | ||
this.unshift(newValue); | ||
this.unshift(newElementOrNode); | ||
return true; | ||
@@ -515,3 +567,3 @@ } | ||
if (current.next.value === existingValue) { | ||
const newNode = new SinglyLinkedListNode(newValue); | ||
const newNode = this._ensureNode(newElementOrNode); | ||
newNode.next = current.next; | ||
@@ -532,20 +584,19 @@ current.next = newNode; | ||
* | ||
* The `addAfter` function inserts a new node with a given value after an existing node in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingValueOrNode - The existing value or node in the linked list after which | ||
* the new value will be inserted. It can be either the value of the existing node or the existing node itself. | ||
* @param {E} newValue - The value that you want to insert into the linked list after the existing value or node. | ||
* @returns The method returns a boolean value. It returns true if the new value was successfully inserted after the | ||
* existing value or node, and false if the existing value or node was not found in the linked list. | ||
* The `addAfter` function in TypeScript adds a new element or node after an existing element or node | ||
* in a singly linked list. | ||
* @param {E | SinglyLinkedListNode<E>} existingElementOrNode - existingElementOrNode can be either | ||
* an element of type E or a SinglyLinkedListNode of type E. | ||
* @param {E | SinglyLinkedListNode<E>} newElementOrNode - The `newElementOrNode` parameter in the | ||
* `addAfter` method represents the element or node that you want to add after the existing element | ||
* or node in a singly linked list. This parameter can be either the value of the new element or a | ||
* reference to a `SinglyLinkedListNode` containing | ||
* @returns The `addAfter` method returns a boolean value - `true` if the new element or node was | ||
* successfully added after the existing element or node, and `false` if the existing element or node | ||
* was not found. | ||
*/ | ||
addAfter(existingValueOrNode: E | SinglyLinkedListNode<E>, newValue: E): boolean { | ||
let existingNode: E | SinglyLinkedListNode<E> | undefined; | ||
addAfter(existingElementOrNode: E | SinglyLinkedListNode<E>, newElementOrNode: E | SinglyLinkedListNode<E>): boolean { | ||
const existingNode: SinglyLinkedListNode<E> | undefined = this.getNode(existingElementOrNode); | ||
if (existingValueOrNode instanceof SinglyLinkedListNode) { | ||
existingNode = existingValueOrNode; | ||
} else { | ||
existingNode = this.getNode(existingValueOrNode); | ||
} | ||
if (existingNode) { | ||
const newNode = new SinglyLinkedListNode(newValue); | ||
const newNode = this._ensureNode(newElementOrNode); | ||
newNode.next = existingNode.next; | ||
@@ -567,7 +618,11 @@ existingNode.next = newNode; | ||
* | ||
* The function counts the number of occurrences of a given value in a linked list. | ||
* @param {E} value - The value parameter is the value that you want to count the occurrences of in the linked list. | ||
* @returns The count of occurrences of the given value in the linked list. | ||
* The function `countOccurrences` iterates through a singly linked list and counts the occurrences | ||
* of a specified element or nodes that satisfy a given predicate. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementOrNode | ||
* - The `elementOrNode` parameter in the `countOccurrences` method can accept three types of values: | ||
* @returns The `countOccurrences` method returns the number of occurrences of the specified element, | ||
* node, or predicate function in the singly linked list. | ||
*/ | ||
countOccurrences(value: E): number { | ||
countOccurrences(elementOrNode: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)): number { | ||
const predicate = this._ensurePredicate(elementOrNode); | ||
let count = 0; | ||
@@ -577,3 +632,3 @@ let current = this.head; | ||
while (current) { | ||
if (current.value === value) { | ||
if (predicate(current)) { | ||
count++; | ||
@@ -675,2 +730,68 @@ } | ||
} | ||
/** | ||
* Time Complexity: O(n) | ||
* Space Complexity: O(n) | ||
* | ||
* The `fromArray` function creates a new SinglyLinkedList instance and populates it with the elements from the given | ||
* array. | ||
* @param {E[]} data - The `data` parameter is an array of elements of type `E`. | ||
* @returns The `fromArray` function returns a `SinglyLinkedList` object. | ||
*/ | ||
static fromArray<E>(data: E[]) { | ||
const singlyLinkedList = new SinglyLinkedList<E>(); | ||
for (const item of data) { | ||
singlyLinkedList.push(item); | ||
} | ||
return singlyLinkedList; | ||
} | ||
/** | ||
* The _isPredicate function in TypeScript checks if the input is a function that takes a | ||
* SinglyLinkedListNode as an argument and returns a boolean. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter can be one of the following types: | ||
* @returns The _isPredicate method is returning a boolean value based on whether the | ||
* elementNodeOrPredicate parameter is a function or not. If the elementNodeOrPredicate is a | ||
* function, the method will return true, indicating that it is a predicate function. If it is not a | ||
* function, the method will return false. | ||
*/ | ||
protected _isPredicate( | ||
elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | ||
): elementNodeOrPredicate is (node: SinglyLinkedListNode<E>) => boolean { | ||
return typeof elementNodeOrPredicate === 'function'; | ||
} | ||
/** | ||
* The function `_ensureNode` ensures that the input is a valid node and returns it, creating a new | ||
* node if necessary. | ||
* @param {E | SinglyLinkedListNode<E>} elementOrNode - The `elementOrNode` parameter can be either | ||
* an element of type `E` or a `SinglyLinkedListNode` containing an element of type `E`. | ||
* @returns A SinglyLinkedListNode<E> object is being returned. | ||
*/ | ||
protected _ensureNode(elementOrNode: E | SinglyLinkedListNode<E>) { | ||
if (this.isNode(elementOrNode)) return elementOrNode; | ||
return new SinglyLinkedListNode<E>(elementOrNode); | ||
} | ||
/** | ||
* The function `_ensurePredicate` in TypeScript ensures that the input is either a node, a predicate | ||
* function, or a value to compare with the node's value. | ||
* @param {E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean)} elementNodeOrPredicate | ||
* elementNodeOrPredicate - The `elementNodeOrPredicate` parameter can be one of the following types: | ||
* @returns A function is being returned. If the input `elementNodeOrPredicate` is already a node, a | ||
* function is returned that checks if a given node is equal to the input node. If the input is a | ||
* predicate function, it is returned as is. If the input is neither a node nor a predicate function, | ||
* a function is returned that checks if a given node's value is equal to the input | ||
*/ | ||
protected _ensurePredicate( | ||
elementNodeOrPredicate: E | SinglyLinkedListNode<E> | ((node: SinglyLinkedListNode<E>) => boolean) | ||
) { | ||
if (this.isNode(elementNodeOrPredicate)) return (node: SinglyLinkedListNode<E>) => node === elementNodeOrPredicate; | ||
if (this._isPredicate(elementNodeOrPredicate)) return elementNodeOrPredicate; | ||
return (node: SinglyLinkedListNode<E>) => node.value === elementNodeOrPredicate; | ||
} | ||
} |
2140186
0.93%41784
0.74%231
-0.86%Updated