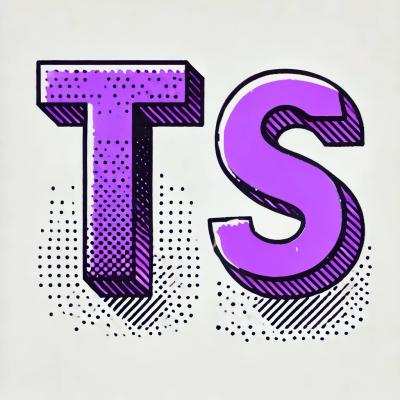
Security News
Node.js Moves Toward Stable TypeScript Support with Amaro 1.0
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
A lightweight ORM toolkit for SQLite in Node.js and Electron applications.
npm install quicklite better-sqlite3
Requires Node.js v14.21.1 or later. The toolkit uses better-sqlite3 v11.8.1 (with SQLite 3.48.0).
import { DatabaseManager } from 'quicklite';
// In Node.js
const dbManager = DatabaseManager.getInstance({
dbPath: './myapp.db',
enableWAL: true,
enableForeignKeys: true
});
// In Electron, typically in main process
import path from 'path';
import { app } from 'electron';
const userDataPath = app.getPath('userData');
const dbPath = path.join(userDataPath, 'database/myapp.db');
const dbManager = DatabaseManager.getInstance({
dbPath,
enableWAL: true,
enableForeignKeys: true
});
// Get the database instance
const db = dbManager.getDatabase();
import { BaseEntity, TableInfo } from 'quicklite';
export class User extends BaseEntity {
id?: number;
username!: string;
email?: string;
createdAt?: number;
// Define table schema
static getTableInfo(): TableInfo {
return {
name: 'users',
primaryKey: 'id',
columns: [
{
name: 'id',
type: 'INTEGER',
primaryKey: true,
autoIncrement: true
},
{
name: 'username',
type: 'TEXT',
notNull: true,
unique: true
},
{
name: 'email',
type: 'TEXT',
unique: true
},
{
name: 'createdAt',
type: 'INTEGER',
default: 'CURRENT_TIMESTAMP'
}
],
indices: [
{
name: 'idx_users_email',
columns: ['email'],
unique: true
}
]
};
}
}
import { DbInitializer } from 'quicklite';
import { User } from './models/User';
import { Post } from './models/Post';
// Get database instance
const db = dbManager.getDatabase();
// Initialize tables
const dbInitializer = new DbInitializer(db);
dbInitializer
.register(User)
.register(Post)
.initTables();
import { BaseService } from 'quicklite';
import { User } from './models/User';
export class UserService extends BaseService<User> {
constructor(db) {
super(db, User);
}
// Custom method to find a user by username
findByUsername(username: string): User | null {
return this.findOne({
where: { username }
});
}
// Add more custom methods as needed
}
// Usage
const userService = new UserService(db);
// Create a new user
const userId = userService.insert({
username: 'johndoe',
email: 'john@example.com'
});
// Get user by ID
const user = userService.getById(userId);
// Find users with conditions
const users = userService.find({
where: { email: 'john@example.com' },
orderBy: 'createdAt DESC',
limit: 10
});
// Update a user
userService.update({
id: userId,
email: 'newemail@example.com'
});
// Delete a user
userService.deleteById(userId);
import { QueryBuilder } from 'quicklite';
// Get database instance
const db = dbManager.getDatabase();
// Build a complex query
const query = new QueryBuilder(db, 'users')
.select('users.*', 'COUNT(posts.id) as postCount')
.leftJoin('posts', 'posts.userId = users.id')
.where('users.createdAt', '>', Date.now() - 30 * 24 * 60 * 60 * 1000)
.andWhere(qb => {
qb.where('users.username', 'LIKE', '%john%')
.or(subQb => {
subQb.where('users.email', 'LIKE', '%john%');
});
})
.groupBy('users.id')
.having('postCount', '>', 5)
.orderBy('postCount', 'DESC')
.limit(10);
// Execute the query
const activeUsers = query.all();
// Get the first result
const topUser = query.first();
// Count matching records
const userCount = query.count();
// Get database instance
const db = dbManager.getDatabase();
// Create a transaction
const transaction = db.transaction(() => {
userService.insert({ username: 'user1' });
userService.insert({ username: 'user2' });
// If any operation fails, all changes will be rolled back
});
// Execute the transaction
transaction();
QuickLite提供了一系列实用工具类,用于辅助数据库操作、性能优化和数据管理。
提供SQLite数据库备份和恢复功能:
import { BackupUtil } from 'quicklite';
// 备份数据库
await BackupUtil.backup(sourceDb, 'backup.db');
// 恢复数据库
await BackupUtil.restore('backup.db', targetDb);
提供数据导入导出和数据传输功能:
import { DataTransferUtil } from 'quicklite';
// 将表数据导出为JSON
await DataTransferUtil.exportToJson(userService, 'users.json');
// 从JSON导入数据
await DataTransferUtil.importFromJson(userService, 'users.json');
// 将查询结果导出为CSV
await DataTransferUtil.exportQueryToCsv(
db,
'SELECT * FROM users WHERE age > 30',
'filtered_users.csv'
);
// 在数据库之间复制表数据
await DataTransferUtil.copyTableData(
sourceDb,
targetDb,
'users',
'users'
);
提供SQL查询性能分析和优化建议:
import { QueryAnalyzer } from 'quicklite';
// 分析SQL查询
const analysis = QueryAnalyzer.analyze(
db,
'SELECT * FROM users WHERE age > 30'
);
console.log('执行时间:', analysis.executionTime, 'ms');
console.log('性能建议:', analysis.suggestions);
// 获取索引建议
const indexSuggestions = QueryAnalyzer.suggestIndices(
db,
`SELECT u.name, o.product, SUM(o.amount) as total
FROM users u
JOIN orders o ON u.id = o.user_id
WHERE u.age > 30
GROUP BY u.id
ORDER BY total DESC`
);
console.log('索引建议:', indexSuggestions);
MIT
FAQs
A lightweight ORM toolkit for SQLite in Node.js applications
The npm package quicklite receives a total of 2 weekly downloads. As such, quicklite popularity was classified as not popular.
We found that quicklite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.