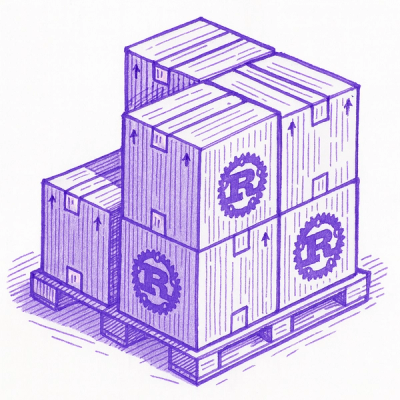
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
QUnit is a powerful, easy-to-use JavaScript unit testing framework. It is used to test any generic JavaScript code, including code running in the browser or in Node.js. QUnit is especially useful for testing jQuery projects.
Basic Test
This feature allows you to write a basic test case. The `QUnit.test` function defines a test with a name and a callback function. The `assert.ok` method checks if the given expression is true.
QUnit.test('hello test', function(assert) {
assert.ok(1 == '1', 'Passed!');
});
Asynchronous Testing
QUnit supports asynchronous testing. You can use async/await to handle asynchronous operations within your tests. The test will wait for the promise to resolve before making assertions.
QUnit.test('asynchronous test: async and await', async function(assert) {
const result = await new Promise(resolve => setTimeout(() => resolve('done'), 1000));
assert.equal(result, 'done', 'Passed!');
});
Module Grouping
QUnit allows you to group related tests using `QUnit.module`. You can also define setup and teardown logic using hooks like `beforeEach` and `afterEach`.
QUnit.module('group a', function(hooks) {
hooks.beforeEach(function(assert) {
assert.ok(true, 'beforeEach called');
});
QUnit.test('a basic test example', function(assert) {
assert.ok(true, 'this test is fine');
});
});
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple and fun. Mocha tests run serially, allowing for flexible and accurate reporting, while mapping uncaught exceptions to the correct test cases. Compared to QUnit, Mocha is more flexible and can be paired with various assertion libraries like Chai.
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. It works out of the box for most JavaScript projects and provides a rich API for writing tests. Jest includes built-in assertion libraries and mocking capabilities, making it a more integrated solution compared to QUnit.
Jasmine is a behavior-driven development framework for testing JavaScript code. It does not depend on any other JavaScript frameworks and does not require a DOM. Jasmine is known for its easy-to-read syntax and is often compared to QUnit for its simplicity and ease of use.
QUnit is a powerful, easy-to-use JavaScript testing framework. It was originally developed for the jQuery project and has since evolved to test any client-side or server-side JavaScript code. QUnit has no dependencies and supports Node.js, SpiderMonkey, and all major web browsers.
To report a bug or request a new feature, open an issue.
If you need help using QUnit, chat with us on Gitter.
If you are interested in helping develop QUnit, check out our contributing guide.
FAQs
The powerful, easy-to-use testing framework.
The npm package qunit receives a total of 302,976 weekly downloads. As such, qunit popularity was classified as popular.
We found that qunit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.