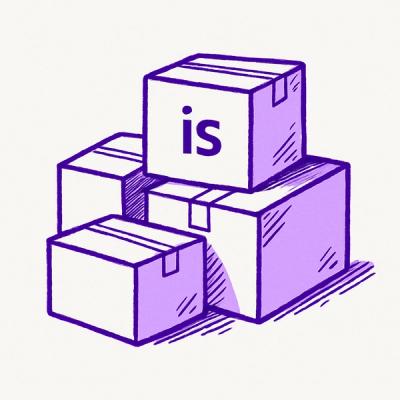
Security News
npm βisβ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
rc-trigger
Advanced tools
The rc-trigger npm package is a React component that is used for positioning and aligning popups, tooltips, dropdowns, and other floating elements relative to a target element. It provides a flexible way to control the visibility and behavior of these overlay components.
Popup Alignment
This feature allows you to align the popup content to the target element. In the code sample, the popup is aligned to the bottom left of the button when clicked, with a small offset.
{"import React from 'react';\nimport Trigger from 'rc-trigger';\nimport 'rc-trigger/assets/index.css';\n\nconst popupContent = <div>Popup Content</div>;\n\nconst Demo = () => (\n <Trigger\n action={['click']}\n popup={popupContent}\n popupAlign={{\n points: ['tl', 'bl'],\n offset: [0, 3],\n }}\n >\n <button>Click me</button>\n </Trigger>\n);\n\nexport default Demo;"}
Trigger Actions
This feature allows you to specify the actions that will trigger the popup. In the code sample, the popup appears when the input field is either hovered over or focused.
{"import React from 'react';\nimport Trigger from 'rc-trigger';\nimport 'rc-trigger/assets/index.css';\n\nconst popupContent = <div>Popup Content</div>;\n\nconst Demo = () => (\n <Trigger\n action={['hover', 'focus']}\n popup={popupContent}\n popupAlign={{\n points: ['tl', 'bl'],\n offset: [0, 3],\n }}\n >\n <input placeholder='Hover or focus me'/>\n </Trigger>\n);\n\nexport default Demo;"}
Built-in Animations
This feature allows you to add animations to the popup when it appears or disappears. In the code sample, the 'slide-up' animation is used when the popup is shown or hidden.
{"import React from 'react';\nimport Trigger from 'rc-trigger';\nimport 'rc-trigger/assets/index.css';\n\nconst popupContent = <div>Popup Content</div>;\n\nconst Demo = () => (\n <Trigger\n action={['click']}\n popup={popupContent}\n popupAlign={{\n points: ['tl', 'bl'],\n offset: [0, 3],\n }}\n popupAnimation='slide-up'\n >\n <button>Click me</button>\n </Trigger>\n);\n\nexport default Demo;"}
react-tether is a React wrapper around the Tether positioning engine. It provides similar functionality to rc-trigger for positioning and aligning elements. However, react-tether is more focused on the Tether library's API and may offer more advanced positioning options.
react-popper is a React wrapper for the Popper.js library. It is used for the same purposes as rc-trigger, such as tooltips and popovers. react-popper leverages Popper.js for positioning, which might give it an edge in terms of positioning accuracy and performance.
react-portal-tooltip is a React component for creating tooltips that are appended to the body of the page. It is similar to rc-trigger in that it helps with creating floating UI elements, but it is specifically tailored for tooltips and may not offer as much flexibility for other types of overlays.
React Trigger Component
Include the default styling and then:
import React from 'react';
import ReactDOM from 'react-dom';
import Trigger from 'rc-trigger';
ReactDOM.render((
<Trigger
action={['click']}
popup={<span>popup</span>}
popupAlign={{
points: ['tl', 'bl'],
offset: [0, 3]
}}
>
<a href='#'>hover</a>
</Trigger>
), container);
![]() IE / Edge | ![]() Firefox | ![]() Chrome | ![]() Safari | ![]() Electron |
---|---|---|---|---|
IE11, Edge | last 2 versions | last 2 versions | last 2 versions | last 2 versions |
online example: http://react-component.github.io/trigger/
npm install
npm start
name | type | default | description |
---|---|---|---|
alignPoint | bool | false | Popup will align with mouse position (support action of 'click', 'hover' and 'contextMenu') |
popupClassName | string | additional className added to popup | |
forceRender | boolean | false | whether render popup before first show |
destroyPopupOnHide | boolean | false | whether destroy popup when hide |
getPopupClassNameFromAlign | getPopupClassNameFromAlign(align: Object):String | additional className added to popup according to align | |
action | string[] | ['hover'] | which actions cause popup shown. enum of 'hover','click','focus','contextMenu' |
mouseEnterDelay | number | 0 | delay time to show when mouse enter. unit: s. |
mouseLeaveDelay | number | 0.1 | delay time to hide when mouse leave. unit: s. |
popupStyle | Object | additional style of popup | |
prefixCls | String | rc-trigger-popup | prefix class name |
popupTransitionName | String|Object | https://github.com/react-component/animate | |
maskTransitionName | String|Object | https://github.com/react-component/animate | |
onPopupVisibleChange | Function | call when popup visible is changed | |
mask | boolean | false | whether to support mask |
maskClosable | boolean | true | whether to support click mask to hide |
popupVisible | boolean | whether popup is visible | |
zIndex | number | popup's zIndex | |
defaultPopupVisible | boolean | whether popup is visible initially | |
popupAlign | Object: alignConfig of [dom-align](https://github.com/yiminghe/dom-align) | popup 's align config | |
onPopupAlign | function(popupDomNode, align) | callback when popup node is aligned | |
popup | React.Element | function() => React.Element | popup content | |
getPopupContainer | getPopupContainer(): HTMLElement | function returning html node which will act as popup container | |
getDocument | getDocument(): HTMLElement | function returning document node which will be attached click event to close trigger | |
popupPlacement | string | use preset popup align config from builtinPlacements, can be merged by popupAlign prop | |
builtinPlacements | object | builtin placement align map. used by placement prop | |
stretch | string | Let popup div stretch with trigger element. enums of 'width', 'minWidth', 'height', 'minHeight'. (You can also mixed with 'height minWidth') |
npm test
npm run chrome-test
npm run coverage
open coverage/ dir
After React 16, you won't access popup element's ref in parent component's componentDidMount, which means following code won't work.
class App extends React.Component {
componentDidMount() {
this.input.focus(); // error, this.input is undefined.
}
render() {
return (
<Trigger
action={['click']}
popup={<div><input ref={node => this.input = node} type="text" /></div>}
>
<button>click</button>
</Trigger>
)
}
}
Consider wrap your popup element to a separate component:
class InputPopup extends React.Component {
componentDidMount() {
this.props.onMount();
}
render() {
return (
<div>
<input ref={this.props.inputRef} type="text" />
</div>
);
}
}
class App extends React.Component {
handlePopupMount() {
this.input.focus(); // error, this.input is undefined.
}
render() {
return (
<Trigger
action={['click']}
popup={<InputPopup inputRef={node => this.input = node} onMount={this.handlePopupMount} />}
>
<button>click</button>
</Trigger>
)
}
}
rc-trigger is released under the MIT license.
FAQs
base abstract trigger component for react
The npm package rc-trigger receives a total of 1,146,180 weekly downloads. As such, rc-trigger popularity was classified as popular.
We found that rc-trigger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.