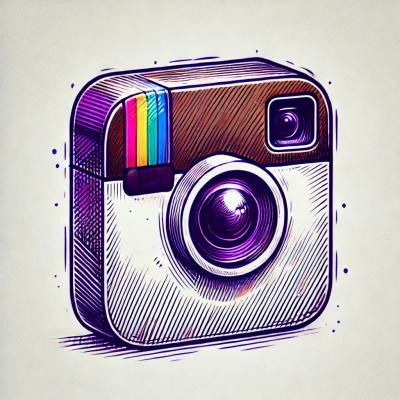
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
react-debounce-input
Advanced tools
The react-debounce-input package provides a React component that debounces the input value change events. This is useful for scenarios where you want to limit the rate at which a function is executed, such as when making API calls based on user input.
Basic Debounced Input
This example demonstrates a basic usage of the DebounceInput component. The input value is debounced with a timeout of 300 milliseconds, meaning the onChange event will only be triggered if the user stops typing for 300 milliseconds.
import React, { useState } from 'react';
import { DebounceInput } from 'react-debounce-input';
function App() {
const [value, setValue] = useState('');
return (
<div>
<DebounceInput
minLength={2}
debounceTimeout={300}
onChange={(event) => setValue(event.target.value)}
/>
<p>Value: {value}</p>
</div>
);
}
export default App;
Debounced Input with Min Length
This example shows how to use the DebounceInput component with a minimum length requirement. The onChange event will only be triggered if the input value has at least 5 characters and the user stops typing for 500 milliseconds.
import React, { useState } from 'react';
import { DebounceInput } from 'react-debounce-input';
function App() {
const [value, setValue] = useState('');
return (
<div>
<DebounceInput
minLength={5}
debounceTimeout={500}
onChange={(event) => setValue(event.target.value)}
/>
<p>Value: {value}</p>
</div>
);
}
export default App;
Debounced Input with Element
This example demonstrates how to use the DebounceInput component with a different HTML element, such as a textarea. The input value is debounced with a timeout of 300 milliseconds.
import React, { useState } from 'react';
import { DebounceInput } from 'react-debounce-input';
function App() {
const [value, setValue] = useState('');
return (
<div>
<DebounceInput
element="textarea"
minLength={2}
debounceTimeout={300}
onChange={(event) => setValue(event.target.value)}
/>
<p>Value: {value}</p>
</div>
);
}
export default App;
The lodash.debounce package provides a function to debounce any function, not just input events. It is more versatile as it can be used in various scenarios beyond React components. However, it requires more manual setup compared to react-debounce-input.
The react-throttle package provides components for throttling and debouncing events in React. It offers similar functionality to react-debounce-input but also includes throttling capabilities, which can be useful for different use cases.
The use-debounce package provides a React hook for debouncing values and functions. It offers a more modern approach using hooks, which can be more convenient and flexible compared to the component-based approach of react-debounce-input.
React component that renders an Input, Textarea or other element with debounced onChange. Can be used as drop-in replacement for <input type="text" />
or <textarea />
http://nkbt.github.io/react-debounce-input
http://codepen.io/nkbt/pen/VvmzLQ
npm install --save react-debounce-input
yarn add react-debounce-input
<script src="https://unpkg.com/react@16.0.0/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-debounce-input/build/react-debounce-input.js"></script>
(Module exposed as `DebounceInput`)
import React from 'react';
import ReactDOM from 'react-dom';
import {DebounceInput} from 'react-debounce-input';
class App extends React.Component {
state = {
value: ''
};
render() {
return (
<div>
<DebounceInput
minLength={2}
debounceTimeout={300}
onChange={event => this.setState({value: event.target.value})} />
<p>Value: {this.state.value}</p>
</div>
);
}
}
const appRoot = document.createElement('div');
document.body.appendChild(appRoot);
ReactDOM.render(<App />, appRoot);
element
: PropTypes.string or React.PropTypes.func (default: "input")You can specify element="textarea". For Example:
<DebounceInput element="textarea" />
Will result in
<textarea />
Note: when rendering a <textarea />
you may wish to set forceNotifyByEnter = {false}
so the user can make new lines without forcing notification of the current value.
This package has only been tested with <input />
and <textarea />
but should work with any element which has value
and onChange
props.
You can also use a custom react component as the element. For Example:
<DebounceInput element={CustomReactComponent} />
Will result in
<CustomReactComponent />
onChange
: PropTypes.func.isRequiredFunction called when value is changed (debounced) with original event passed through
value
: PropTypes.stringValue of the Input box. Can be omitted, so component works as usual non-controlled input.
minLength
: PropTypes.number (default: 0)Minimal length of text to start notify, if value becomes shorter then minLength
(after removing some characters), there will be a notification with empty value ''
.
debounceTimeout
: PropTypes.number (default: 100)Notification debounce timeout in ms. If set to -1
, disables automatic notification completely. Notification will only happen by pressing Enter
then.
forceNotifyByEnter
: PropTypes.bool (default: true)Notification of current value will be sent immediately by hitting Enter
key. Enabled by-default. Notification value follows the same rule as with debounced notification, so if Length is less, then minLength
- empty value ''
will be sent back.
NOTE if onKeyDown
callback prop was present, it will be still invoked transparently.
forceNotifyOnBlur
: PropTypes.bool (default: true)Same as forceNotifyByEnter
, but notification will be sent when focus leaves the input field.
inputRef
: PropTypes.func (default: undefined)Will pass ref={inputRef}
to generated input element. We needed to rename ref
to inputRef
since ref
is a special prop in React and cannot be passed to children.
See ./example/Ref.js for usage example.
<input>
<DebounceInput
type="number"
onChange={event => this.setState({value: event.target.value})}
placeholder="Name"
className="user-name" />
Will result in
<input
type="number"
placeholder="Name"
className="user-name" />
This library has typescript typings, import them the same way as in javascript:
import {DebounceInput} from 'react-debounce-input';
Also there are helper types DebounceTextArea
and Debounced
to provide strict interfaces for wrapping components different from standard <input />
. Check usage examples in example/typescript-example.tsx
.
NOTE library author is not using Typescript, so if you are using typings and found an issue, please submit a PR with fix. Thanks @iyegoroff for the initial TS support!
Currently is being developed and tested with the latest stable Node
on OSX
.
To run example covering all DebounceInput
features, use yarn start
, which will compile example/Example.js
git clone git@github.com:nkbt/react-debounce-input.git
cd react-debounce-input
yarn install
yarn start
# then
open http://localhost:8080
# to run ESLint check
yarn lint
# to run tests
yarn test
MIT
FAQs
React component that renders Input with debounced onChange
The npm package react-debounce-input receives a total of 458,349 weekly downloads. As such, react-debounce-input popularity was classified as popular.
We found that react-debounce-input demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.