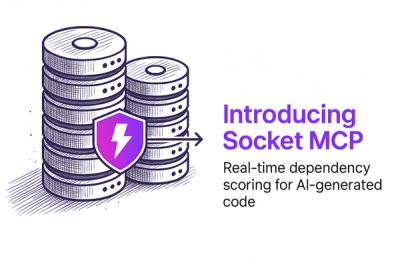
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
react-dom
Advanced tools
The react-dom package provides DOM-specific methods that can be used at the top level of a web app to enable an efficient way of managing DOM elements in response to data changes. It is a companion package to React that facilitates rendering components to the DOM and interacting with the DOM tree.
Rendering React Elements
This feature allows you to render a React element into the DOM in the supplied container and return a reference to the component (or returns null for stateless components).
ReactDOM.render(
<h1>Hello, world!</h1>,
document.getElementById('root')
);
Component Lifecycle Management
react-dom manages the lifecycle of components, including mounting, updating, and unmounting components.
class MyComponent extends React.Component {
componentDidMount() {
// Code to run when the component is mounted
}
componentWillUnmount() {
// Code to run before the component is unmounted and destroyed
}
}
Handling Events
react-dom provides a synthetic event system that wraps the native event system, providing a cross-browser interface to native events.
function MyComponent() {
function handleClick(e) {
e.preventDefault();
console.log('The link was clicked.');
}
return (
<a href="#" onClick={handleClick}>
Click me
</a>
);
}
Server-side Rendering
react-dom/server provides methods for rendering components to static markup (typically used on the server) such as renderToString and renderToStaticMarkup.
ReactDOMServer.renderToString(
<MyComponent />
);
Portals
Portals provide a way to render children into a DOM node that exists outside the DOM hierarchy of the parent component.
ReactDOM.createPortal(
child,
container
);
Preact is a fast 3kB alternative to React with the same modern API. It provides similar functionalities for rendering UIs but with a smaller footprint, making it a good choice for performance-sensitive applications.
Inferno is an extremely fast, React-like library for building high-performance user interfaces on both the client and server. It offers a similar component-based UI building experience but focuses on performance optimizations.
This package is part of the Vue ecosystem and provides server-side rendering capabilities similar to react-dom/server. It's used to render Vue components on the server and send the static markup to the client.
react-dom
This package serves as the entry point to the DOM and server renderers for React. It is intended to be paired with the generic React package, which is shipped as react
to npm.
npm install react react-dom
import { createRoot } from 'react-dom/client';
function App() {
return <div>Hello World</div>;
}
const root = createRoot(document.getElementById('root'));
root.render(<App />);
import { renderToPipeableStream } from 'react-dom/server';
function App() {
return <div>Hello World</div>;
}
function handleRequest(res) {
// ... in your server handler ...
const stream = renderToPipeableStream(<App />, {
onShellReady() {
res.statusCode = 200;
res.setHeader('Content-type', 'text/html');
stream.pipe(res);
},
// ...
});
}
react-dom
See https://react.dev/reference/react-dom
react-dom/client
See https://react.dev/reference/react-dom/client
react-dom/server
19.1.0 (March 28, 2025)
An Owner Stack is a string representing the components that are directly responsible for rendering a particular component. You can log Owner Stacks when debugging or use Owner Stacks to enhance error overlays or other development tools. Owner Stacks are only available in development builds. Component Stacks in production are unchanged.
useId
to use valid CSS selectors, changing format from :r123:
to «r123»
. #32001href
attribute is an empty string #31783getHoistableRoot()
didn’t work properly when the container was a Document #32321<!-- -->
) as a DOM container. #32250<script>
and <template>
tags to be nested within <select>
tags. #31837exports
field to package.json
for use-sync-external-store
to support various entrypoints. #25231unstable_prerender
, a new experimental API for prerendering React Server Components on the server #31724registerServerReference
in client builds to handle server references in different environments. #32534FAQs
React package for working with the DOM.
The npm package react-dom receives a total of 23,600,389 weekly downloads. As such, react-dom popularity was classified as popular.
We found that react-dom demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.