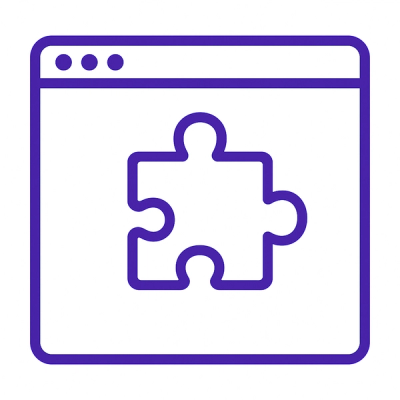
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
react-grid-dnd
Advanced tools
Grid style drag and drop, built with React. See a live example on codesandbox. You can also see it in action here.
Install react-grid-dnd
and react-gesture-responder
using yarn or npm.
yarn add react-grid-dnd react-gesture-responder
Because GridItem
components are rendered with absolute positioning, you need to ensure that GridDropZone
has a specified height or flex, like in the example below.
import {
GridContextProvider,
GridDropZone,
GridItem,
swap
} from "react-grid-dnd";
function Example() {
const [items, setItems] = React.useState([1, 2, 3, 4]); // supply your own state
// target id will only be set if dragging from one dropzone to another.
function onChange(sourceId, sourceIndex, targetIndex, targetId) {
const nextState = swap(items, sourceIndex, targetIndex);
setItems(nextState);
}
return (
<GridContextProvider onChange={onChange}>
<GridDropZone
id="items"
boxesPerRow={4}
rowHeight={100}
style={{ height: "400px" }}
>
{items.map(item => (
<GridItem key={item}>
<div
style={{
width: "100%",
height: "100%"
}}
>
{item}
</div>
</GridItem>
))}
</GridDropZone>
</GridContextProvider>
);
}
You can see this example in action on codesandbox.
import {
GridContextProvider,
GridDropZone,
GridItem,
swap,
move
} from "react-grid-dnd";
function App() {
const [items, setItems] = React.useState({
left: [
{ id: 1, name: "ben" },
{ id: 2, name: "joe" },
{ id: 3, name: "jason" },
{ id: 4, name: "chris" },
{ id: 5, name: "heather" },
{ id: 6, name: "Richard" }
],
right: [
{ id: 7, name: "george" },
{ id: 8, name: "rupert" },
{ id: 9, name: "alice" },
{ id: 10, name: "katherine" },
{ id: 11, name: "pam" },
{ id: 12, name: "katie" }
]
});
function onChange(sourceId, sourceIndex, targetIndex, targetId) {
if (targetId) {
const result = move(
items[sourceId],
items[targetId],
sourceIndex,
targetIndex
);
return setItems({
...items,
[sourceId]: result[0],
[targetId]: result[1]
});
}
const result = swap(items[sourceId], sourceIndex, targetIndex);
return setItems({
...items,
[sourceId]: result
});
}
return (
<GridContextProvider onChange={onChange}>
<div className="container">
<GridDropZone
className="dropzone left"
id="left"
boxesPerRow={4}
rowHeight={70}
>
{items.left.map(item => (
<GridItem key={item.name}>
<div className="grid-item">
<div className="grid-item-content">
{item.name[0].toUpperCase()}
</div>
</div>
</GridItem>
))}
</GridDropZone>
<GridDropZone
className="dropzone right"
id="right"
boxesPerRow={4}
rowHeight={70}
>
{items.right.map(item => (
<GridItem key={item.name}>
<div className="grid-item">
<div className="grid-item-content">
{item.name[0].toUpperCase()}
</div>
</div>
</GridItem>
))}
</GridDropZone>
</div>
</GridContextProvider>
);
}
FAQs
grid style drag and drop for react
The npm package react-grid-dnd receives a total of 7,244 weekly downloads. As such, react-grid-dnd popularity was classified as popular.
We found that react-grid-dnd demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.