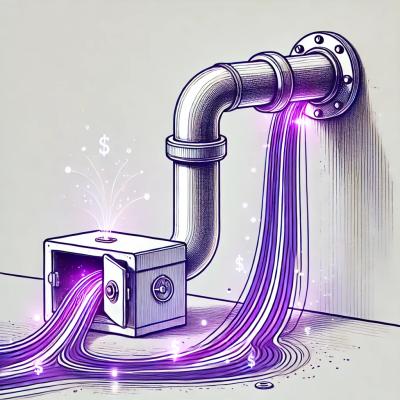
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
react-html-parser
Advanced tools
The react-html-parser npm package is used to parse HTML strings into React components. This allows developers to dynamically render HTML content within their React applications.
Basic HTML Parsing
This feature allows you to parse a basic HTML string and render it as React components. The HTML string is converted into React elements that can be rendered in the application.
const React = require('react');
const ReactDOM = require('react-dom');
const ReactHtmlParser = require('react-html-parser');
const htmlString = '<div><h1>Hello, World!</h1><p>This is a paragraph.</p></div>';
const App = () => (
<div>
{ReactHtmlParser(htmlString)}
</div>
);
ReactDOM.render(<App />, document.getElementById('root'));
Handling Inline Styles
This feature allows you to parse HTML strings that include inline styles. The styles are preserved and applied to the rendered React components.
const React = require('react');
const ReactDOM = require('react-dom');
const ReactHtmlParser = require('react-html-parser');
const htmlString = '<div style="color: red;"><h1>Hello, World!</h1><p>This is a paragraph with inline styles.</p></div>';
const App = () => (
<div>
{ReactHtmlParser(htmlString)}
</div>
);
ReactDOM.render(<App />, document.getElementById('root'));
Custom Transformations
This feature allows you to apply custom transformations to specific HTML elements during the parsing process. In this example, the <h1> tag is transformed to have a blue color.
const React = require('react');
const ReactDOM = require('react-dom');
const ReactHtmlParser = require('react-html-parser');
const htmlString = '<div><h1>Hello, World!</h1><p>This is a paragraph.</p></div>';
const transform = (node) => {
if (node.type === 'tag' && node.name === 'h1') {
return <h1 style={{ color: 'blue' }}>{node.children[0].data}</h1>;
}
};
const App = () => (
<div>
{ReactHtmlParser(htmlString, { transform })}
</div>
);
ReactDOM.render(<App />, document.getElementById('root'));
html-react-parser is a similar package that parses HTML strings into React components. It offers a more modern and actively maintained alternative to react-html-parser, with additional features like support for custom processing instructions and better handling of complex HTML structures.
react-dom-parser is another package that converts HTML strings into React elements. It focuses on providing a simple and lightweight solution for rendering HTML content in React applications, with a straightforward API and minimal dependencies.
react-render-html is a package that allows you to render HTML strings as React components. It provides a simple API for converting HTML content into React elements, with support for custom transformations and event handling.
A utility for converting HTML strings into React components. Avoids the use of dangerouslySetInnerHTML and converts standard HTML elements, attributes and inline styles into their React equivalents.
npm install react-html-parser
# or
yarn add react-html-parser
import React from 'react';
import ReactHtmlParser, { processNodes, convertNodeToElement, htmlparser2 } from 'react-html-parser';
class HtmlComponent extends React.Component {
render() {
const html = '<div>Example HTML string</div>';
return <div>{ ReactHtmlParser(html) }</div>;
}
}
function ReactHtmlParser(html, [options])
Takes an HTML string and returns equivalent React elements
import ReactHtmlParser from 'react-html-parser';
html
: The HTML string to parseoptions
: Options object
htmlparser2
The transform function will be called for every node that is parsed by the library.
function transform(node, index)
node
: The node being parsed. This is the htmlparser2 node object. Full details can be found on their project page but important properties are:
type
(string): The type of node (tag, text, style etc)name
(string): The name of the nodechildren
(array): Array of children nodesnext
(node): The node's next siblingprev
(node): The node's previous siblingparent
(node): The node's parentdata
(string): The text content, if the type
is textindex
(number): The index of the node in relation to it's parentreturn null
Returning null will prevent the node and all of it's children from being rendered.
function transform(node) {
// do not render any <span> tags
if (node.type === 'tag' && node.name === 'span') {
return null;
}
}
return undefined
If the function does not return anything, or returns undefined, then the default behaviour will occur and the parser will continue was usual.
return React element
React elements can be returned directly
import React from 'react';
function transform(node) {
if (node.type === 'tag' && node.name === 'b') {
return <div>This was a bold tag</div>;
}
}
Allows pre-processing the nodes generated from the html by htmlparser2
before being passed to the library and converted to React elements.
function preprocessNodes(nodes)
nodes
: The entire node tree generated by htmlparser2
.The preprocessNodes
function should return a valid htmlparser2
node tree.
function convertNodeToElement(node, index, transform)
Processes a node and returns the React element to be rendered. This function can be used in conjunction with the previously described transform
function to continue to process a node after modifying it.
import { convertNodeToElement } from 'react-html-parser';
node
: The node to processindex
(number): The index of the node in relation to it's parenttransform
: The transform function as described aboveimport { convertNodeToElement } from 'react-html-parser';
function transform(node, index) {
// convert <ul> to <ol>
if (node.type === 'tag' && node.name === 'ul') {
node.name = 'ol';
return convertNodeToElement(node, index, transform);
}
}
htmlparser2
The library exposes the htmlparser2
library it uses. This allows consumers
to use it without having to add it as a separate dependency.
See https://github.com/fb55/htmlparser2 for full details.
FAQs
Parse HTML into React components
The npm package react-html-parser receives a total of 170,925 weekly downloads. As such, react-html-parser popularity was classified as popular.
We found that react-html-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.