react-image-gallery
Advanced tools
Comparing version
'use strict'; | ||
Object.defineProperty(exports, '__esModule', { | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
var _createClass = (function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ('value' in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; })(); | ||
var _assign = require('babel-runtime/core-js/object/assign'); | ||
var _get = function get(_x, _x2, _x3) { var _again = true; _function: while (_again) { var object = _x, property = _x2, receiver = _x3; _again = false; if (object === null) object = Function.prototype; var desc = Object.getOwnPropertyDescriptor(object, property); if (desc === undefined) { var parent = Object.getPrototypeOf(object); if (parent === null) { return undefined; } else { _x = parent; _x2 = property; _x3 = receiver; _again = true; desc = parent = undefined; continue _function; } } else if ('value' in desc) { return desc.value; } else { var getter = desc.get; if (getter === undefined) { return undefined; } return getter.call(receiver); } } }; | ||
var _assign2 = _interopRequireDefault(_assign); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; } | ||
var _getPrototypeOf = require('babel-runtime/core-js/object/get-prototype-of'); | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError('Cannot call a class as a function'); } } | ||
var _getPrototypeOf2 = _interopRequireDefault(_getPrototypeOf); | ||
function _inherits(subClass, superClass) { if (typeof superClass !== 'function' && superClass !== null) { throw new TypeError('Super expression must either be null or a function, not ' + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } | ||
var _classCallCheck2 = require('babel-runtime/helpers/classCallCheck'); | ||
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2); | ||
var _createClass2 = require('babel-runtime/helpers/createClass'); | ||
var _createClass3 = _interopRequireDefault(_createClass2); | ||
var _possibleConstructorReturn2 = require('babel-runtime/helpers/possibleConstructorReturn'); | ||
var _possibleConstructorReturn3 = _interopRequireDefault(_possibleConstructorReturn2); | ||
var _inherits2 = require('babel-runtime/helpers/inherits'); | ||
var _inherits3 = _interopRequireDefault(_inherits2); | ||
var _react = require('react'); | ||
@@ -25,33 +39,71 @@ | ||
var ImageGallery = (function (_React$Component) { | ||
_inherits(ImageGallery, _React$Component); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
var MIN_INTERVAL = 500; | ||
function throttle(func, wait) { | ||
var context = void 0, | ||
args = void 0, | ||
result = void 0; | ||
var timeout = null; | ||
var previous = 0; | ||
var later = function later() { | ||
previous = new Date().getTime(); | ||
timeout = null; | ||
result = func.apply(context, args); | ||
if (!timeout) context = args = null; | ||
}; | ||
return function () { | ||
var now = new Date().getTime(); | ||
var remaining = wait - (now - previous); | ||
context = this; | ||
args = arguments; | ||
if (remaining <= 0 || remaining > wait) { | ||
if (timeout) { | ||
clearTimeout(timeout); | ||
timeout = null; | ||
} | ||
previous = now; | ||
result = func.apply(context, args); | ||
if (!timeout) context = args = null; | ||
} else if (!timeout) { | ||
timeout = setTimeout(later, remaining); | ||
} | ||
return result; | ||
}; | ||
} | ||
var ImageGallery = function (_React$Component) { | ||
(0, _inherits3.default)(ImageGallery, _React$Component); | ||
function ImageGallery(props) { | ||
_classCallCheck(this, ImageGallery); | ||
(0, _classCallCheck3.default)(this, ImageGallery); | ||
_get(Object.getPrototypeOf(ImageGallery.prototype), 'constructor', this).call(this, props); | ||
this.state = { | ||
var _this = (0, _possibleConstructorReturn3.default)(this, (0, _getPrototypeOf2.default)(ImageGallery).call(this, props)); | ||
_this.state = { | ||
currentIndex: props.startIndex, | ||
thumbnailsTranslateX: 0, | ||
containerWidth: 0 | ||
thumbsTranslateX: 0, | ||
offsetPercentage: 0, | ||
galleryWidth: 0 | ||
}; | ||
this._handleResize = this._handleResize.bind(this); | ||
_this._slideLeft = throttle(_this._slideLeft, MIN_INTERVAL, true); | ||
_this._slideRight = throttle(_this._slideRight, MIN_INTERVAL, true); | ||
_this._handleResize = _this._handleResize.bind(_this); | ||
_this._handleKeyDown = _this._handleKeyDown.bind(_this); | ||
return _this; | ||
} | ||
_createClass(ImageGallery, [{ | ||
(0, _createClass3.default)(ImageGallery, [{ | ||
key: 'componentDidUpdate', | ||
value: function componentDidUpdate(prevProps, prevState) { | ||
if (prevState.containerWidth !== this.state.containerWidth || prevProps.showThumbnails !== this.props.showThumbnails) { | ||
if (prevState.galleryWidth !== this.state.galleryWidth || prevProps.showThumbnails !== this.props.showThumbnails) { | ||
// adjust thumbnail container when window width is adjusted | ||
// when the container resizes, thumbnailsTranslateX | ||
// should always be negative (moving right), | ||
// if container fits all thumbnails its set to 0 | ||
this._setThumbnailsTranslateX(-this._getScrollX(this.state.currentIndex > 0 ? 1 : 0) * this.state.currentIndex); | ||
this._setThumbsTranslateX(-this._getThumbsTranslateX(this.state.currentIndex > 0 ? 1 : 0) * this.state.currentIndex); | ||
} | ||
if (prevState.currentIndex !== this.state.currentIndex) { | ||
// call back function if provided | ||
if (this.props.onSlide) { | ||
@@ -61,16 +113,3 @@ this.props.onSlide(this.state.currentIndex); | ||
// calculates thumbnail container position | ||
if (this.state.currentIndex === 0) { | ||
this._setThumbnailsTranslateX(0); | ||
} else { | ||
var indexDifference = Math.abs(prevState.currentIndex - this.state.currentIndex); | ||
var _scrollX = this._getScrollX(indexDifference); | ||
if (_scrollX > 0) { | ||
if (prevState.currentIndex < this.state.currentIndex) { | ||
this._setThumbnailsTranslateX(this.state.thumbnailsTranslateX - _scrollX); | ||
} else if (prevState.currentIndex > this.state.currentIndex) { | ||
this._setThumbnailsTranslateX(this.state.thumbnailsTranslateX + _scrollX); | ||
} | ||
} | ||
} | ||
this._updateThumbnailTranslateX(prevState); | ||
} | ||
@@ -88,6 +127,11 @@ } | ||
value: function componentDidMount() { | ||
this._handleResize(); | ||
var _this2 = this; | ||
window.setTimeout(function () { | ||
_this2._handleResize(), 300; | ||
}); | ||
if (this.props.autoPlay) { | ||
this.play(); | ||
} | ||
window.addEventListener('keydown', this._handleKeyDown); | ||
window.addEventListener('resize', this._handleResize); | ||
@@ -98,2 +142,3 @@ } | ||
value: function componentWillUnmount() { | ||
window.removeEventListener('keydown', this._handleKeyDown); | ||
window.removeEventListener('resize', this._handleResize); | ||
@@ -106,34 +151,26 @@ if (this._intervalId) { | ||
}, { | ||
key: 'slideToIndex', | ||
value: function slideToIndex(index, event) { | ||
var slideCount = this.props.items.length - 1; | ||
if (index < 0) { | ||
this.setState({ currentIndex: slideCount }); | ||
} else if (index > slideCount) { | ||
this.setState({ currentIndex: 0 }); | ||
} else { | ||
this.setState({ currentIndex: index }); | ||
} | ||
if (event) { | ||
if (this._intervalId) { | ||
// user event, reset interval | ||
this.pause(); | ||
this.play(); | ||
} | ||
} | ||
} | ||
}, { | ||
key: 'play', | ||
value: function play() { | ||
var _this = this; | ||
var _this3 = this; | ||
var callback = arguments.length <= 0 || arguments[0] === undefined ? true : arguments[0]; | ||
if (this._intervalId) { | ||
return; | ||
} | ||
var slideInterval = this.props.slideInterval; | ||
this._intervalId = window.setInterval(function () { | ||
if (!_this.state.hovering) { | ||
_this.slideToIndex(_this.state.currentIndex + 1); | ||
if (!_this3.state.hovering) { | ||
if (!_this3.props.infinite && !_this3._canSlideRight()) { | ||
_this3.pause(); | ||
} else { | ||
_this3.slideToIndex(_this3.state.currentIndex + 1); | ||
} | ||
} | ||
}, this.props.slideInterval); | ||
}, slideInterval > MIN_INTERVAL ? slideInterval : MIN_INTERVAL); | ||
if (this.props.onPlay && callback) { | ||
this.props.onPlay(this.state.currentIndex); | ||
} | ||
} | ||
@@ -143,2 +180,4 @@ }, { | ||
value: function pause() { | ||
var callback = arguments.length <= 0 || arguments[0] === undefined ? true : arguments[0]; | ||
if (this._intervalId) { | ||
@@ -148,11 +187,44 @@ window.clearInterval(this._intervalId); | ||
} | ||
if (this.props.onPause && callback) { | ||
this.props.onPause(this.state.currentIndex); | ||
} | ||
} | ||
}, { | ||
key: 'slideToIndex', | ||
value: function slideToIndex(index, event) { | ||
var slideCount = this.props.items.length - 1; | ||
var currentIndex = index; | ||
if (index < 0) { | ||
currentIndex = slideCount; | ||
} else if (index > slideCount) { | ||
currentIndex = 0; | ||
} | ||
this.setState({ | ||
previousIndex: this.state.currentIndex, | ||
currentIndex: currentIndex, | ||
offsetPercentage: 0, | ||
style: { | ||
transition: 'transform .45s ease-out' | ||
} | ||
}); | ||
if (event) { | ||
if (this._intervalId) { | ||
// user event, while playing, reset interval | ||
this.pause(false); | ||
this.play(false); | ||
} | ||
} | ||
} | ||
}, { | ||
key: '_wrapClick', | ||
value: function _wrapClick(func) { | ||
var _this2 = this; | ||
var _this4 = this; | ||
if (typeof func === 'function') { | ||
return function (event) { | ||
if (_this2._preventGhostClick === true) { | ||
if (_this4._preventGhostClick === true) { | ||
return; | ||
@@ -167,40 +239,35 @@ } | ||
value: function _touchEnd() { | ||
var _this3 = this; | ||
var _this5 = this; | ||
this._preventGhostClick = true; | ||
this._preventGhostClickTimer = window.setTimeout(function () { | ||
_this3._preventGhostClick = false; | ||
_this3._preventGhostClickTimer = null; | ||
_this5._preventGhostClick = false; | ||
_this5._preventGhostClickTimer = null; | ||
}, this._ghotClickDelay); | ||
} | ||
}, { | ||
key: '_setThumbnailsTranslateX', | ||
value: function _setThumbnailsTranslateX(x) { | ||
this.setState({ thumbnailsTranslateX: x }); | ||
} | ||
}, { | ||
key: '_handleResize', | ||
value: function _handleResize() { | ||
this.setState({ containerWidth: this._imageGallery.offsetWidth }); | ||
if (this._imageGallery) { | ||
this.setState({ galleryWidth: this._imageGallery.offsetWidth }); | ||
} | ||
} | ||
}, { | ||
key: '_getScrollX', | ||
value: function _getScrollX(indexDifference) { | ||
if (this.props.disableThumbnailScroll) { | ||
return 0; | ||
} | ||
if (this._thumbnails) { | ||
if (this._thumbnails.scrollWidth <= this.state.containerWidth) { | ||
return 0; | ||
} | ||
key: '_handleKeyDown', | ||
value: function _handleKeyDown(event) { | ||
var LEFT_ARROW = 37; | ||
var RIGHT_ARROW = 39; | ||
var key = parseInt(event.keyCode || event.which || 0); | ||
var totalThumbnails = this._thumbnails.children.length; | ||
// total scroll-x required to see the last thumbnail | ||
var totalScrollX = this._thumbnails.scrollWidth - this.state.containerWidth; | ||
// scroll-x required per index change | ||
var perIndexScrollX = totalScrollX / (totalThumbnails - 1); | ||
return indexDifference * perIndexScrollX; | ||
switch (key) { | ||
case LEFT_ARROW: | ||
if (this._canSlideLeft() && !this._intervalId) { | ||
this._slideLeft(); | ||
} | ||
break; | ||
case RIGHT_ARROW: | ||
if (this._canSlideRight() && !this._intervalId) { | ||
this._slideRight(); | ||
} | ||
break; | ||
} | ||
@@ -211,3 +278,3 @@ } | ||
value: function _handleMouseOverThumbnails(index) { | ||
var _this4 = this; | ||
var _this6 = this; | ||
@@ -221,4 +288,3 @@ if (this.props.slideOnThumbnailHover) { | ||
this._thumbnailTimer = window.setTimeout(function () { | ||
_this4.slideToIndex(index); | ||
_this4.pause(); | ||
_this6.slideToIndex(index); | ||
}, this._thumbnailDelay); | ||
@@ -233,4 +299,4 @@ } | ||
this._thumbnailTimer = null; | ||
if (this.props.autoPlay == true) { | ||
this.play(); | ||
if (this.props.autoPlay === true) { | ||
this.play(false); | ||
} | ||
@@ -251,25 +317,133 @@ } | ||
}, { | ||
key: '_handleImageError', | ||
value: function _handleImageError(event) { | ||
if (this.props.defaultImage && event.target.src.indexOf(this.props.defaultImage) === -1) { | ||
event.target.src = this.props.defaultImage; | ||
} | ||
} | ||
}, { | ||
key: '_handleOnSwiped', | ||
value: function _handleOnSwiped(ev, x, y, isFlick) { | ||
this.setState({ isFlick: isFlick }); | ||
} | ||
}, { | ||
key: '_handleOnSwipedTo', | ||
value: function _handleOnSwipedTo(index) { | ||
var slideTo = this.state.currentIndex; | ||
if (Math.abs(this.state.offsetPercentage) > 30 || this.state.isFlick) { | ||
slideTo += index; | ||
} | ||
if (index < 0) { | ||
if (!this._canSlideLeft()) { | ||
slideTo = this.state.currentIndex; | ||
} | ||
} else { | ||
if (!this._canSlideRight()) { | ||
slideTo = this.state.currentIndex; | ||
} | ||
} | ||
this.slideToIndex(slideTo); | ||
} | ||
}, { | ||
key: '_handleSwiping', | ||
value: function _handleSwiping(index, _, delta) { | ||
var offsetPercentage = index * (delta / this.state.galleryWidth * 100); | ||
this.setState({ offsetPercentage: offsetPercentage, style: {} }); | ||
} | ||
}, { | ||
key: '_canNavigate', | ||
value: function _canNavigate() { | ||
return this.props.items.length >= 2; | ||
} | ||
}, { | ||
key: '_canSlideLeft', | ||
value: function _canSlideLeft() { | ||
if (this.props.infinite) { | ||
return true; | ||
} else { | ||
return this.state.currentIndex > 0; | ||
} | ||
} | ||
}, { | ||
key: '_canSlideRight', | ||
value: function _canSlideRight() { | ||
if (this.props.infinite) { | ||
return true; | ||
} else { | ||
return this.state.currentIndex < this.props.items.length - 1; | ||
} | ||
} | ||
}, { | ||
key: '_updateThumbnailTranslateX', | ||
value: function _updateThumbnailTranslateX(prevState) { | ||
if (this.state.currentIndex === 0) { | ||
this._setThumbsTranslateX(0); | ||
} else { | ||
var indexDifference = Math.abs(prevState.currentIndex - this.state.currentIndex); | ||
var scrollX = this._getThumbsTranslateX(indexDifference); | ||
if (scrollX > 0) { | ||
if (prevState.currentIndex < this.state.currentIndex) { | ||
this._setThumbsTranslateX(this.state.thumbsTranslateX - scrollX); | ||
} else if (prevState.currentIndex > this.state.currentIndex) { | ||
this._setThumbsTranslateX(this.state.thumbsTranslateX + scrollX); | ||
} | ||
} | ||
} | ||
} | ||
}, { | ||
key: '_setThumbsTranslateX', | ||
value: function _setThumbsTranslateX(thumbsTranslateX) { | ||
this.setState({ thumbsTranslateX: thumbsTranslateX }); | ||
} | ||
}, { | ||
key: '_getThumbsTranslateX', | ||
value: function _getThumbsTranslateX(indexDifference) { | ||
if (this.props.disableThumbnailScroll) { | ||
return 0; | ||
} | ||
if (this._thumbnails) { | ||
if (this._thumbnails.scrollWidth <= this.state.galleryWidth) { | ||
return 0; | ||
} | ||
var totalThumbnails = this._thumbnails.children.length; | ||
// total scroll-x required to see the last thumbnail | ||
var totalScrollX = this._thumbnails.scrollWidth - this.state.galleryWidth; | ||
// scroll-x required per index change | ||
var perIndexScrollX = totalScrollX / (totalThumbnails - 1); | ||
return indexDifference * perIndexScrollX; | ||
} | ||
} | ||
}, { | ||
key: '_getAlignmentClassName', | ||
value: function _getAlignmentClassName(index) { | ||
var currentIndex = this.state.currentIndex; | ||
var alignment = ''; | ||
var LEFT = 'left'; | ||
var CENTER = 'center'; | ||
var RIGHT = 'right'; | ||
switch (index) { | ||
case currentIndex - 1: | ||
alignment = ' left'; | ||
alignment = ' ' + LEFT; | ||
break; | ||
case currentIndex: | ||
alignment = ' center'; | ||
alignment = ' ' + CENTER; | ||
break; | ||
case currentIndex + 1: | ||
alignment = ' right'; | ||
alignment = ' ' + RIGHT; | ||
break; | ||
} | ||
if (this.props.items.length >= 3) { | ||
if (this.props.items.length >= 3 && this.props.infinite) { | ||
if (index === 0 && currentIndex === this.props.items.length - 1) { | ||
// set first slide as right slide if were sliding right from last slide | ||
alignment = ' right'; | ||
alignment = ' ' + RIGHT; | ||
} else if (index === this.props.items.length - 1 && currentIndex === 0) { | ||
// set last slide as left slide if were sliding left from first slide | ||
alignment = ' left'; | ||
alignment = ' ' + LEFT; | ||
} | ||
@@ -281,38 +455,76 @@ } | ||
}, { | ||
key: '_handleImageLoad', | ||
value: function _handleImageLoad(event) { | ||
// slide images have an opacity of 0, onLoad the class 'loaded' is added | ||
// so that it transitions smoothly when navigating to non adjacent slides | ||
if (event.target.className.indexOf('loaded') === -1) { | ||
event.target.className += ' loaded'; | ||
key: '_getSlideStyle', | ||
value: function _getSlideStyle(index) { | ||
var _state = this.state; | ||
var currentIndex = _state.currentIndex; | ||
var offsetPercentage = _state.offsetPercentage; | ||
var basetranslateX = -100 * currentIndex; | ||
var totalSlides = this.props.items.length - 1; | ||
var translateX = basetranslateX + index * 100 + offsetPercentage; | ||
var zIndex = 1; | ||
if (this.props.infinite) { | ||
if (currentIndex === 0 && index === totalSlides) { | ||
// make the last slide the slide before the first | ||
translateX = -100 + offsetPercentage; | ||
} else if (currentIndex === totalSlides && index === 0) { | ||
// make the first slide the slide after the last | ||
translateX = 100 + offsetPercentage; | ||
} | ||
} | ||
// current index has more zIndex so slides wont fly by toggling infinite | ||
if (index === currentIndex) { | ||
zIndex = 3; | ||
} else if (index === this.state.previousIndex) { | ||
zIndex = 2; | ||
} | ||
var translate3d = 'translate3d(' + translateX + '%, 0, 0)'; | ||
return { | ||
WebkitTransform: translate3d, | ||
MozTransform: translate3d, | ||
msTransform: translate3d, | ||
OTransform: translate3d, | ||
transform: translate3d, | ||
zIndex: zIndex | ||
}; | ||
} | ||
}, { | ||
key: '_handleImageError', | ||
value: function _handleImageError(event) { | ||
if (this.props.defaultImage && event.target.src.indexOf(this.props.defaultImage) === -1) { | ||
event.target.src = this.props.defaultImage; | ||
} | ||
key: '_getThumbnailStyle', | ||
value: function _getThumbnailStyle() { | ||
var translate3d = 'translate3d(' + this.state.thumbsTranslateX + 'px, 0, 0)'; | ||
return { | ||
WebkitTransform: translate3d, | ||
MozTransform: translate3d, | ||
msTransform: translate3d, | ||
OTransform: translate3d, | ||
transform: translate3d | ||
}; | ||
} | ||
}, { | ||
key: '_hasMinSlidesToShowNav', | ||
value: function _hasMinSlidesToShowNav() { | ||
return this.props.items.length >= 2; | ||
key: '_slideLeft', | ||
value: function _slideLeft() { | ||
this.slideToIndex(this.state.currentIndex - 1); | ||
} | ||
}, { | ||
key: '_slideRight', | ||
value: function _slideRight() { | ||
this.slideToIndex(this.state.currentIndex + 1); | ||
} | ||
}, { | ||
key: 'render', | ||
value: function render() { | ||
var _this5 = this; | ||
var _this7 = this; | ||
var currentIndex = this.state.currentIndex; | ||
var thumbnailStyle = { | ||
MozTransform: 'translate3d(' + this.state.thumbnailsTranslateX + 'px, 0, 0)', | ||
WebkitTransform: 'translate3d(' + this.state.thumbnailsTranslateX + 'px, 0, 0)', | ||
OTransform: 'translate3d(' + this.state.thumbnailsTranslateX + 'px, 0, 0)', | ||
msTransform: 'translate3d(' + this.state.thumbnailsTranslateX + 'px, 0, 0)', | ||
transform: 'translate3d(' + this.state.thumbnailsTranslateX + 'px, 0, 0)' | ||
}; | ||
var swipePrev = this.slideToIndex.bind(this, currentIndex - 1); | ||
var swipeNext = this.slideToIndex.bind(this, currentIndex + 1); | ||
var thumbnailStyle = this._getThumbnailStyle(); | ||
var slideLeft = this._slideLeft.bind(this); | ||
var slideRight = this._slideRight.bind(this); | ||
var slides = []; | ||
@@ -323,7 +535,7 @@ var thumbnails = []; | ||
this.props.items.map(function (item, index) { | ||
var alignment = _this5._getAlignmentClassName(index); | ||
var alignment = _this7._getAlignmentClassName(index); | ||
var originalClass = item.originalClass ? ' ' + item.originalClass : ''; | ||
var thumbnailClass = item.thumbnailClass ? ' ' + item.thumbnailClass : ''; | ||
var slide = _react2['default'].createElement( | ||
var slide = _react2.default.createElement( | ||
'div', | ||
@@ -333,15 +545,25 @@ { | ||
className: 'image-gallery-slide' + alignment + originalClass, | ||
onClick: _this5._wrapClick(_this5.props.onClick), | ||
onTouchStart: _this5.props.onClick, | ||
onTouchEnd: _this5._touchEnd.bind(_this5) }, | ||
_react2['default'].createElement('img', { | ||
className: _this5.props.server ? 'loaded' : null, | ||
src: item.original, | ||
alt: item.originalAlt, | ||
onLoad: _this5._handleImageLoad, | ||
onError: _this5._handleImageError }), | ||
item.description | ||
style: (0, _assign2.default)(_this7._getSlideStyle(index), _this7.state.style), | ||
onClick: _this7._wrapClick(_this7.props.onClick), | ||
onTouchStart: _this7.props.onClick, | ||
onTouchEnd: _this7._touchEnd.bind(_this7) | ||
}, | ||
_react2.default.createElement( | ||
'div', | ||
{ className: 'image-gallery-image' }, | ||
_react2.default.createElement('img', { | ||
src: item.original, | ||
alt: item.originalAlt, | ||
onLoad: _this7.props.onImageLoad, | ||
onError: _this7._handleImageError.bind(_this7) | ||
}), | ||
item.description && _react2.default.createElement( | ||
'span', | ||
{ className: 'image-gallery-description' }, | ||
item.description | ||
) | ||
) | ||
); | ||
if (_this5.props.lazyLoad) { | ||
if (_this7.props.lazyLoad) { | ||
if (alignment) { | ||
@@ -354,38 +576,38 @@ slides.push(slide); | ||
if (_this5.props.showThumbnails) { | ||
thumbnails.push(_react2['default'].createElement( | ||
if (_this7.props.showThumbnails) { | ||
thumbnails.push(_react2.default.createElement( | ||
'a', | ||
{ | ||
onMouseOver: _this5._handleMouseOverThumbnails.bind(_this5, index), | ||
onMouseLeave: _this5._handleMouseLeaveThumbnails.bind(_this5, index), | ||
onMouseOver: _this7._handleMouseOverThumbnails.bind(_this7, index), | ||
onMouseLeave: _this7._handleMouseLeaveThumbnails.bind(_this7, index), | ||
key: index, | ||
className: 'image-gallery-thumbnail' + (currentIndex === index ? ' active' : '') + thumbnailClass, | ||
onTouchStart: _this5.slideToIndex.bind(_this5, index), | ||
onTouchEnd: _this5._touchEnd.bind(_this5), | ||
onClick: _this5._wrapClick(_this5.slideToIndex.bind(_this5, index)) }, | ||
_react2['default'].createElement('img', { | ||
onTouchStart: _this7.slideToIndex.bind(_this7, index), | ||
onTouchEnd: _this7._touchEnd.bind(_this7), | ||
onClick: _this7._wrapClick(_this7.slideToIndex.bind(_this7, index)) }, | ||
_react2.default.createElement('img', { | ||
src: item.thumbnail, | ||
alt: item.thumbnailAlt, | ||
onError: _this5._handleImageError }) | ||
onError: _this7._handleImageError.bind(_this7) }) | ||
)); | ||
} | ||
if (_this5.props.showBullets) { | ||
bullets.push(_react2['default'].createElement('li', { | ||
if (_this7.props.showBullets) { | ||
bullets.push(_react2.default.createElement('li', { | ||
key: index, | ||
className: 'image-gallery-bullet ' + (currentIndex === index ? 'active' : ''), | ||
onTouchStart: _this5.slideToIndex.bind(_this5, index), | ||
onTouchEnd: _this5._touchEnd.bind(_this5), | ||
onClick: _this5._wrapClick(_this5.slideToIndex.bind(_this5, index)) })); | ||
onTouchStart: _this7.slideToIndex.bind(_this7, index), | ||
onTouchEnd: _this7._touchEnd.bind(_this7), | ||
onClick: _this7._wrapClick(_this7.slideToIndex.bind(_this7, index)) })); | ||
} | ||
}); | ||
return _react2['default'].createElement( | ||
return _react2.default.createElement( | ||
'section', | ||
{ ref: function (i) { | ||
return _this5._imageGallery = i; | ||
{ ref: function ref(i) { | ||
return _this7._imageGallery = i; | ||
}, className: 'image-gallery' }, | ||
_react2['default'].createElement( | ||
_react2.default.createElement( | ||
'div', | ||
@@ -396,19 +618,27 @@ { | ||
className: 'image-gallery-content' }, | ||
this._hasMinSlidesToShowNav() ? [this.props.showNav && [_react2['default'].createElement('a', { | ||
key: 'leftNav', | ||
className: 'image-gallery-left-nav', | ||
onTouchStart: swipePrev, | ||
onTouchEnd: this._touchEnd.bind(this), | ||
onClick: this._wrapClick(swipePrev) }), _react2['default'].createElement('a', { | ||
key: 'rightNav', | ||
className: 'image-gallery-right-nav', | ||
onTouchStart: swipeNext, | ||
onTouchEnd: this._touchEnd.bind(this), | ||
onClick: this._wrapClick(swipeNext) })], _react2['default'].createElement( | ||
_reactSwipeable2['default'], | ||
this._canNavigate() ? [this.props.showNav && _react2.default.createElement( | ||
'span', | ||
{ key: 'navigation' }, | ||
this._canSlideLeft() && _react2.default.createElement('a', { | ||
className: 'image-gallery-left-nav', | ||
onTouchStart: slideLeft, | ||
onTouchEnd: this._touchEnd.bind(this), | ||
onClick: this._wrapClick(slideLeft) }), | ||
this._canSlideRight() && _react2.default.createElement('a', { | ||
className: 'image-gallery-right-nav', | ||
onTouchStart: slideRight, | ||
onTouchEnd: this._touchEnd.bind(this), | ||
onClick: this._wrapClick(slideRight) }) | ||
), _react2.default.createElement( | ||
_reactSwipeable2.default, | ||
{ | ||
className: 'image-gallery-swipe', | ||
key: 'swipeable', | ||
onSwipedLeft: swipeNext, | ||
onSwipedRight: swipePrev }, | ||
_react2['default'].createElement( | ||
onSwipingLeft: this._handleSwiping.bind(this, -1), | ||
onSwipingRight: this._handleSwiping.bind(this, 1), | ||
onSwiped: this._handleOnSwiped.bind(this), | ||
onSwipedLeft: this._handleOnSwipedTo.bind(this, 1), | ||
onSwipedRight: this._handleOnSwipedTo.bind(this, -1) | ||
}, | ||
_react2.default.createElement( | ||
'div', | ||
@@ -418,3 +648,3 @@ { className: 'image-gallery-slides' }, | ||
) | ||
)] : _react2['default'].createElement( | ||
)] : _react2.default.createElement( | ||
'div', | ||
@@ -424,6 +654,6 @@ { className: 'image-gallery-slides' }, | ||
), | ||
this.props.showBullets && _react2['default'].createElement( | ||
this.props.showBullets && _react2.default.createElement( | ||
'div', | ||
{ className: 'image-gallery-bullets' }, | ||
_react2['default'].createElement( | ||
_react2.default.createElement( | ||
'ul', | ||
@@ -434,6 +664,6 @@ { className: 'image-gallery-bullets-container' }, | ||
), | ||
this.props.showIndex && _react2['default'].createElement( | ||
this.props.showIndex && _react2.default.createElement( | ||
'div', | ||
{ className: 'image-gallery-index' }, | ||
_react2['default'].createElement( | ||
_react2.default.createElement( | ||
'span', | ||
@@ -443,3 +673,3 @@ { className: 'image-gallery-index-current' }, | ||
), | ||
_react2['default'].createElement( | ||
_react2.default.createElement( | ||
'span', | ||
@@ -449,3 +679,3 @@ { className: 'image-gallery-index-separator' }, | ||
), | ||
_react2['default'].createElement( | ||
_react2.default.createElement( | ||
'span', | ||
@@ -457,10 +687,10 @@ { className: 'image-gallery-index-total' }, | ||
), | ||
this.props.showThumbnails && _react2['default'].createElement( | ||
this.props.showThumbnails && _react2.default.createElement( | ||
'div', | ||
{ className: 'image-gallery-thumbnails' }, | ||
_react2['default'].createElement( | ||
_react2.default.createElement( | ||
'div', | ||
{ | ||
ref: function (t) { | ||
return _this5._thumbnails = t; | ||
ref: function ref(t) { | ||
return _this7._thumbnails = t; | ||
}, | ||
@@ -475,41 +705,44 @@ className: 'image-gallery-thumbnails-container', | ||
}]); | ||
return ImageGallery; | ||
})(_react2['default'].Component); | ||
}(_react2.default.Component); | ||
exports['default'] = ImageGallery; | ||
exports.default = ImageGallery; | ||
ImageGallery.propTypes = { | ||
items: _react2['default'].PropTypes.array.isRequired, | ||
showThumbnails: _react2['default'].PropTypes.bool, | ||
showBullets: _react2['default'].PropTypes.bool, | ||
showNav: _react2['default'].PropTypes.bool, | ||
showIndex: _react2['default'].PropTypes.bool, | ||
indexSeparator: _react2['default'].PropTypes.string, | ||
autoPlay: _react2['default'].PropTypes.bool, | ||
lazyLoad: _react2['default'].PropTypes.bool, | ||
slideInterval: _react2['default'].PropTypes.number, | ||
onSlide: _react2['default'].PropTypes.func, | ||
onClick: _react2['default'].PropTypes.func, | ||
startIndex: _react2['default'].PropTypes.number, | ||
defaultImage: _react2['default'].PropTypes.string, | ||
disableThumbnailScroll: _react2['default'].PropTypes.bool, | ||
slideOnThumbnailHover: _react2['default'].PropTypes.bool, | ||
server: _react2['default'].PropTypes.bool | ||
items: _react2.default.PropTypes.array.isRequired, | ||
showNav: _react2.default.PropTypes.bool, | ||
autoPlay: _react2.default.PropTypes.bool, | ||
lazyLoad: _react2.default.PropTypes.bool, | ||
infinite: _react2.default.PropTypes.bool, | ||
showIndex: _react2.default.PropTypes.bool, | ||
showBullets: _react2.default.PropTypes.bool, | ||
showThumbnails: _react2.default.PropTypes.bool, | ||
slideOnThumbnailHover: _react2.default.PropTypes.bool, | ||
disableThumbnailScroll: _react2.default.PropTypes.bool, | ||
defaultImage: _react2.default.PropTypes.string, | ||
indexSeparator: _react2.default.PropTypes.string, | ||
startIndex: _react2.default.PropTypes.number, | ||
slideInterval: _react2.default.PropTypes.number, | ||
onSlide: _react2.default.PropTypes.func, | ||
onPause: _react2.default.PropTypes.func, | ||
onPlay: _react2.default.PropTypes.func, | ||
onClick: _react2.default.PropTypes.func, | ||
onImageLoad: _react2.default.PropTypes.func | ||
}; | ||
ImageGallery.defaultProps = { | ||
lazyLoad: true, | ||
showThumbnails: true, | ||
items: [], | ||
showNav: true, | ||
autoPlay: false, | ||
lazyLoad: false, | ||
infinite: true, | ||
showIndex: false, | ||
showBullets: false, | ||
showIndex: false, | ||
showThumbnails: true, | ||
slideOnThumbnailHover: false, | ||
disableThumbnailScroll: false, | ||
indexSeparator: ' / ', | ||
autoPlay: false, | ||
disableThumbnailScroll: false, | ||
server: false, | ||
slideOnThumbnailHover: false, | ||
slideInterval: 4000, | ||
startIndex: 0 | ||
}; | ||
module.exports = exports['default']; | ||
startIndex: 0, | ||
slideInterval: 3000 | ||
}; |
@@ -1,2 +0,1 @@ | ||
var babelify = require('babelify') | ||
var babel = require('gulp-babel') | ||
@@ -10,6 +9,4 @@ var browserify = require('browserify') | ||
var sass = require('gulp-sass') | ||
var sourcemaps = require('gulp-sourcemaps') | ||
var source = require('vinyl-source-stream') | ||
var buffer = require('vinyl-buffer') | ||
var uglify = require('gulp-uglify') | ||
var watchify = require('watchify') | ||
@@ -19,2 +16,3 @@ | ||
connect.server({ | ||
host: '0.0.0.0', | ||
root: ['example', 'build'], | ||
@@ -27,3 +25,3 @@ port: 8001, | ||
gulp.task('sass', function () { | ||
gulp.src('./src/ImageGallery.scss') | ||
gulp.src('./src/image-gallery.scss') | ||
.pipe(sass()) | ||
@@ -37,12 +35,9 @@ .pipe(rename('image-gallery.css')) | ||
watchify(browserify({ | ||
entries: ['./example/app.js'], | ||
entries: './example/app.js', | ||
extensions: ['.jsx'], | ||
transform: [babelify] | ||
})) | ||
debug: true | ||
}).transform('babelify', {presets: ['es2015', 'react']})) | ||
.bundle() | ||
.pipe(source('example.js')) | ||
.pipe(buffer()) | ||
.pipe(sourcemaps.init({loadMaps: true})) | ||
.pipe(uglify()) | ||
.pipe(sourcemaps.write('.')) | ||
.pipe(gulp.dest('./example/')) | ||
@@ -53,5 +48,8 @@ .pipe(livereload()) | ||
gulp.task('source-js', function () { | ||
return gulp.src('./src/ImageGallery.react.jsx') | ||
return gulp.src('./src/ImageGallery.jsx') | ||
.pipe(concat('image-gallery.js')) | ||
.pipe(babel()) | ||
.pipe(babel({ | ||
plugins: ['transform-runtime'], | ||
presets: ['es2015', 'react'] | ||
})) | ||
.pipe(gulp.dest('./build')) | ||
@@ -58,0 +56,0 @@ }) |
{ | ||
"name": "react-image-gallery", | ||
"version": "0.5.13", | ||
"description": "Image gallery, Carousel, Slide Show component for React", | ||
"version": "0.6.0", | ||
"description": "React Image gallery, React Carousel, React Slide Show component", | ||
"main": "./build/image-gallery", | ||
@@ -19,2 +19,5 @@ "scripts": { | ||
"react-gallery", | ||
"react carousel", | ||
"react slideshow", | ||
"react gallery", | ||
"image gallery", | ||
@@ -35,6 +38,10 @@ "image slider", | ||
"devDependencies": { | ||
"babelify": "^6.1.3", | ||
"browserify": "^9.0.3", | ||
"babel-plugin-transform-runtime": "^6.7.5", | ||
"babel-preset-es2015": "^6.6.0", | ||
"babel-preset-react": "^6.5.0", | ||
"babel-runtime": "^6.6.1", | ||
"babelify": "^7.2.0", | ||
"browserify": "^13.0.0", | ||
"gulp": "^3.8.11", | ||
"gulp-babel": "^5.2.0", | ||
"gulp-babel": "^6.1.2", | ||
"gulp-concat": "^2.6.0", | ||
@@ -45,7 +52,4 @@ "gulp-connect": "^2.2.0", | ||
"gulp-sass": "^2.1.1", | ||
"gulp-sourcemaps": "^1.6.0", | ||
"gulp-uglify": "^1.2.0", | ||
"react": "^0.14.0", | ||
"react-addons-linked-state-mixin": "^0.14.0", | ||
"react-dom": "^0.14.0", | ||
"react": "^15.0.1", | ||
"react-dom": "^15.0.1", | ||
"vinyl-buffer": "^1.0.0", | ||
@@ -56,4 +60,4 @@ "vinyl-source-stream": "^1.0.0", | ||
"dependencies": { | ||
"react-swipeable": "^3.0.2" | ||
"react-swipeable": "^3.3.1" | ||
} | ||
} |
104
README.md
@@ -5,11 +5,12 @@ React Image Gallery | ||
[](https://badge.fury.io/js/react-image-gallery) | ||
[](http://www.npmjs.com/package/react-image-gallery) | ||
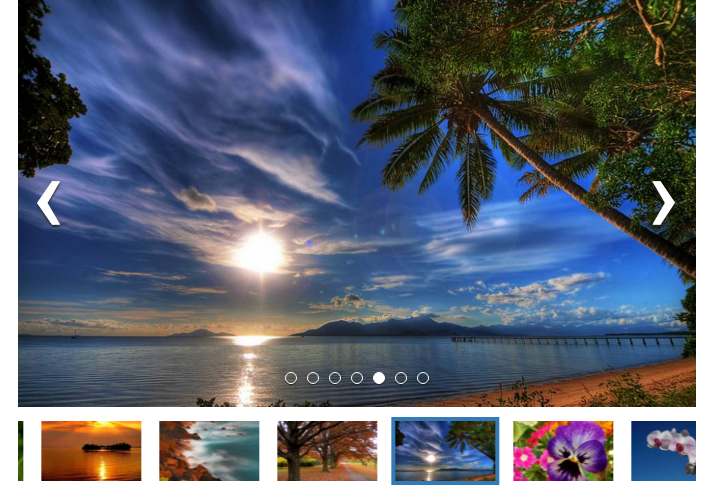 | ||
 | ||
React image gallery is a React component for building image carousels | ||
React image gallery is a React component for building image gallery and carousels | ||
Features of `react-image-gallery` | ||
* Mobile friendly | ||
* Thumbnail navigation | ||
* Responsive design | ||
* Thumbnail navigation | ||
* Mobile friendly | ||
@@ -28,3 +29,3 @@ ## Demo & Examples | ||
``` | ||
@import "../node_modules/react-image-gallery/src/ImageGallery"; | ||
@import "../node_modules/react-image-gallery/src/image-gallery"; | ||
``` | ||
@@ -38,41 +39,57 @@ | ||
### JSX | ||
### EXAMPLE | ||
Need more example? See example/app.js | ||
```js | ||
var ImageGallery = require('react-image-gallery'); | ||
import ImageGallery from 'react-image-gallery'; | ||
var images = [ | ||
{ | ||
original: 'http://lorempixel.com/1000/600/nature/1/', | ||
thumbnail: 'http://lorempixel.com/250/150/nature/1/', | ||
originalClass: 'featured-slide', | ||
thumbnailClass: 'featured-thumb', | ||
originalAlt: 'original-alt', | ||
thumbnailAlt: 'thumbnail-alt', | ||
description: 'Optional description...' | ||
}, | ||
{ | ||
original: 'http://lorempixel.com/1000/600/nature/2/', | ||
thumbnail: 'http://lorempixel.com/250/150/nature/2/' | ||
}, | ||
{ | ||
original: 'http://lorempixel.com/1000/600/nature/3/', | ||
thumbnail: 'http://lorempixel.com/250/150/nature/3/' | ||
class MyComponent extends React.Component { | ||
handleImageLoad(event) { | ||
console.log('Image loaded ', event.target) | ||
} | ||
]; | ||
handleSlide: function(index) { | ||
console.log('Slid to ' + index); | ||
}, | ||
handlePlay() { | ||
this._imageGallery.play() | ||
} | ||
render: function() { | ||
return ( | ||
<ImageGallery | ||
items={images} | ||
autoPlay={true} | ||
slideInterval={4000} | ||
onSlide={this.handleSlide}/> | ||
); | ||
handlePause() { | ||
this._imageGallery.pause() | ||
} | ||
render() { | ||
const images = [ | ||
{ | ||
original: 'http://lorempixel.com/1000/600/nature/1/', | ||
thumbnail: 'http://lorempixel.com/250/150/nature/1/', | ||
originalClass: 'featured-slide', | ||
thumbnailClass: 'featured-thumb', | ||
originalAlt: 'original-alt', | ||
thumbnailAlt: 'thumbnail-alt', | ||
description: 'Optional description...' | ||
}, | ||
{ | ||
original: 'http://lorempixel.com/1000/600/nature/2/', | ||
thumbnail: 'http://lorempixel.com/250/150/nature/2/' | ||
}, | ||
{ | ||
original: 'http://lorempixel.com/1000/600/nature/3/', | ||
thumbnail: 'http://lorempixel.com/250/150/nature/3/' | ||
} | ||
] | ||
return ( | ||
<div> | ||
<button onClick={this.handlePlay.bind(this)}>Play</button> | ||
<button onClick={this.handlePause.bind(this)}>Pause</button> | ||
<ImageGallery | ||
ref={i => this._imageGallery = i} | ||
items={images} | ||
slideInterval={2000} | ||
handleImageLoad={this.handleImageLoad}/> | ||
</div> | ||
); | ||
} | ||
} | ||
``` | ||
@@ -83,3 +100,5 @@ | ||
* `items`: (required) Array of objects, see example above, | ||
* `lazyLoad`: Boolean, default `true` | ||
* `infinite`: Boolean, default `true` | ||
* infinite sliding | ||
* `lazyLoad`: Boolean, default `false` | ||
* `showNav`: Boolean, default `true` | ||
@@ -89,4 +108,2 @@ * `showThumbnails`: Boolean, default `true` | ||
* `showIndex`: Boolean, default `false` | ||
* `server`: Boolean, default `false` | ||
* adds `loaded` class to all slide `<img>` | ||
* `autoPlay`: Boolean, default `false` | ||
@@ -102,3 +119,6 @@ * `disableThumbnailScroll`: Boolean, default `false` | ||
* `startIndex`: Integer, default `0` | ||
* `onSlide`: Function, `callback(index)` | ||
* `onImageLoad`: Function, `callback(event)` | ||
* `onSlide`: Function, `callback(currentIndex)` | ||
* `onPause`: Function, `callback(currentIndex)` | ||
* `onPlay`: Function, `callback(currentIndex)` | ||
* `onClick`: Function, `callback(event)` | ||
@@ -105,0 +125,0 @@ |
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
URL strings
Supply chain riskPackage contains fragments of external URLs or IP addresses, which the package may be accessing at runtime.
Found 1 instance in 1 package
55219
20.86%1389
33.17%145
16%18
5.88%1
Infinity%1
Infinity%Updated