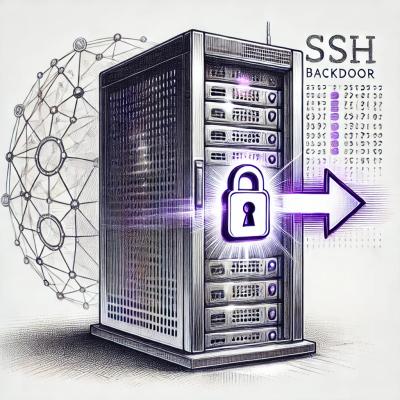
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
react-leaflet
Advanced tools
The react-leaflet package is a React wrapper for the Leaflet library, which is a popular open-source JavaScript library for interactive maps. It allows developers to easily integrate and manipulate maps within React applications.
Displaying a Map
This code sample demonstrates how to display a basic map using react-leaflet. The MapContainer component is used to create the map, and the TileLayer component is used to add a tile layer from OpenStreetMap.
import { MapContainer, TileLayer } from 'react-leaflet';
function MyMap() {
return (
<MapContainer center={[51.505, -0.09]} zoom={13} style={{ height: '100vh', width: '100%' }}>
<TileLayer
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
attribution="© <a href='https://www.openstreetmap.org/copyright'>OpenStreetMap</a> contributors"
/>
</MapContainer>
);
}
Adding Markers
This code sample shows how to add a marker to the map. The Marker component is used to place a marker at a specific position, and the Popup component is used to display a popup when the marker is clicked.
import { MapContainer, TileLayer, Marker, Popup } from 'react-leaflet';
function MyMap() {
return (
<MapContainer center={[51.505, -0.09]} zoom={13} style={{ height: '100vh', width: '100%' }}>
<TileLayer
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
attribution="© <a href='https://www.openstreetmap.org/copyright'>OpenStreetMap</a> contributors"
/>
<Marker position={[51.505, -0.09]}>
<Popup>
A pretty CSS3 popup. <br /> Easily customizable.
</Popup>
</Marker>
</MapContainer>
);
}
Drawing Shapes
This code sample demonstrates how to draw a polygon on the map. The Polygon component is used to create a polygon shape by specifying an array of latitude and longitude coordinates.
import { MapContainer, TileLayer, Polygon } from 'react-leaflet';
function MyMap() {
const polygon = [
[51.505, -0.09],
[51.51, -0.1],
[51.51, -0.12]
];
return (
<MapContainer center={[51.505, -0.09]} zoom={13} style={{ height: '100vh', width: '100%' }}>
<TileLayer
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
attribution="© <a href='https://www.openstreetmap.org/copyright'>OpenStreetMap</a> contributors"
/>
<Polygon positions={polygon} />
</MapContainer>
);
}
react-map-gl is a React wrapper for Mapbox GL JS, which is a powerful library for interactive maps. It offers more advanced features and customization options compared to react-leaflet, but it requires a Mapbox access token.
google-maps-react is a library for integrating Google Maps into React applications. It provides a simple API for adding maps and markers, but it is dependent on the Google Maps API, which may have usage limits and requires an API key.
react-google-maps is another library for using Google Maps in React applications. It offers a more declarative approach compared to google-maps-react and includes additional features like drawing tools and heatmaps.
FAQs
React components for Leaflet maps
We found that react-leaflet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.