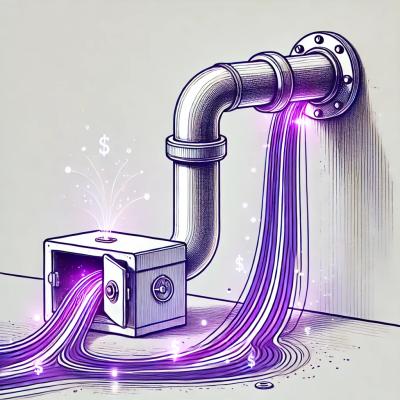
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
react-native-awesome-slider
Advanced tools
Slider component written using Reanimated v2, high performance/fps, also be used for video/audio scrubber.
First you have to follow installation instructions of Reanimated v2 and react-native-gesture-handler
If you react-native-gesture-handler version >= 2:
yarn add react-native-awesome-slider
else:
yarn add react-native-awesome-slider@1
Basic use:
import { useSharedValue } from 'react-native-reanimated';
import { Slider } from 'react-native-awesome-slider';
export const Example = () => {
const progress = useSharedValue(30);
const min = useSharedValue(0);
const max = useSharedValue(100);
return (
<Slider
style={styles.container}
progress={progress}
minimumValue={min}
maximumValue={max}
/>
);
};
Use the Theme object.
<Slider
theme={{
disableMinTrackTintColor: '#fff',
maximumTrackTintColor: '#fff',
minimumTrackTintColor: '#000',
cacheTrackTintColor: '#333',
bubbleBackgroundColor: '#666',
heartbeatColor: '#999',
}}
/>
For more usage, please view Example.
<Slider
onHapticFeedback={() => {
ReactNativeHapticFeedback.trigger('impactLight', {
enableVibrateFallback: true,
ignoreAndroidSystemSettings: false,
});
}}
/>
Set haptic mode, if you open 'step' prop, you need set hapticMode=HapticModeEnum.STEP, otherwise set to hapticMode=HapticModeEnum.BOTH.
✅ Finish!
react-native
's gesture events which may inadvertently trigger 'swipe to go back' events as you pan the slider. ❌react-native-awesome-slider
relies on reanimated
's ShareValue ability to run code directly in the UIThread
to enhance performance, and react-native-gesture-handle
won't interfere with your swiping gestures. ✨
TypeScript
.react-native-reanimated
and react-native-gesture-handler
.react-native-gesture-handler
v2The <Slider/>
component has the following configuration properties:
Name | Type | Description | Required | Default Value |
theme | object | The slider theme color | ❌ | { // Color to fill the progress in the seekbar minimumTrackTintColor: string, // Color to fill the background in the seekbar maximumTrackTintColor: string, // Color to fill the cache in the seekbar cacheTrackTintColor: string, // Color to fill the bubble backgrouundColor disableMinTrackTintColor: string, // Disabled color to fill the progress in the seekbar bubbleBackgroundColor: string // Color to fill the heartbeat animation in the seekbar heartbeatColor: string } |
style | ViewStyle | ❌ | ||
borderColor | string | Color of the border of the slider, also you can use containerStyle . | ❌ | transparent |
bubble | (number) => string | Get the current value of the slider as you slide it, and returns a string to be used inside the bubble. | ❌ | (number) => string |
progress | Animated.SharedValue<number> | Current value of the slider | ✅ | 0 |
cache | Animated.SharedValue<number> | Cache value of the slider | ❌ | 0 |
minimumValue | Animated.SharedValue<number> | An Animated.SharedValue from react-native-reanimated library which is the minimum value of the slider. | ✅ | undefined |
maximumValue | Animated.SharedValue<number> | An Animated.SharedValue from react-native-reanimated library which is the maximum value of the slider. | ✅ | undefined |
onSlidingStart | () => void | Callback called when the sliding interaction starts | ❌ | undefined |
onValueChange | (number) => void | Callback called when the slider value changes | ❌ | undefined |
onSlidingComplete | (number) => void | Callback called when the sliding interaction stops. The updated slider value will be passed as argument | ❌ | undefined |
renderBubble | () => React.ReactNode | A custom bubble component that will be rendered while sliding. | ❌ | See the <Bubble/> component |
setBubbleText | (string) => void | This function will be called while sliding and can be used to update the text in a custom bubble component. | ❌ | current slider value |
bubbleTranslateY | number | Value to pass to the container of the bubble as translateY | ❌ | 7 |
renderThumb | () => React.ReactNode | Render custom thumb image. If you need to customize thumb, you also need to set the thumb width | ❌ | ReactNode |
renderMark | ({ index }: { index: number }) => React.ReactNode | Render custom mark element. If you need to customize mark, you also need to set the mark width | ❌ | ReactNode |
thumbWidth | number | Thumb elements width | ❌ | 15 |
disable | boolean | Disable user interaction with the slider | ❌ | false |
disableTapEvent | boolean | Enable tap event change value. Defaults to `true` | ❌ | true |
bubbleMaxWidth | number | The maximum width of the bubble component | ❌ | 100 |
bubbleTextStyle | TextStyle | Bubble text style | ❌ | |
bubbleContainerStyle | ViewStyle | Bubble container text style | ❌ | |
isScrubbing | Animated.SharedValue<boolean> | callback slider is scrubbing status | ❌ | undefined |
onTap | () => void | A callback for when the slider is tapped.(Useful for video-player scrubbing.) | ❌ | undefined |
thumbScaleValue | Animated.SharedValue<number> | Control thumb’s transform-scale animation. | ❌ | undefined |
sliderHeight | number | The height of the slider component | ❌ | 30 |
containerStyle | ViewStyle | styles to be applied to the slider container component | ❌ | { width: '100%', height: 5, borderRadius: 2, borderColor: borderColor, overflow: 'hidden', borderWidth: 1, backgroundColor: maximumTrackTintColor, }, |
panHitSlop | object | pan gesture hit slop | ❌ | `{ top: 8, left: 0, bottom: 8, right: 0,} ` |
step | number(integer) | Step value of the slider. The value should be between 0 and maximumValue - minimumValue) | ❌ | undefined |
steps | number(integer) | Count of segmented sliders. | ❌ | undefined |
snapToStep | boolean | Enables or disables step snapping | ❌ | true |
markWidth | number | Step mark width, if you need custom mark style, you must be fix this width | ❌ | 4 |
marksStyle | ViewStyle | Step mark style | ❌ | {width: {markWidth}, height: 4, backgroundColor: '#fff', position: 'absolute', top: 2} |
onHapticFeedback | function | Haptic feedback callback | ❌ | undefined |
hapticMode | enum | haptic feedback mode | ❌ | HapticModeEnum.NONE |
panDirectionValue | enum | Current swipe direction | ❌ | undefined |
disableTrackFollow | boolean | Disable track follow thumb.(Commonly used in video audio players) | ❌ | false |
bubbleWidth | number | Bubble width, If you set this value, bubble positioning left & right will be clamp. | ❌ | 0 |
activeOffsetX | number | number[] | Range along X axis (in points) where fingers travels without activation of gesture. Moving outside of this range implies activation of gesture. | ❌ | undefined |
activeOffsetY | number | number[] | Range along Y axis (in points) where fingers travels without activation of gesture. Moving outside of this range implies activation of gesture. | ❌ | undefined |
failOffsetX | number | number[] | When the finger moves outside this range (in points) along X axis and gesture hasn't yet activated it will fail recognizing the gesture. Range can be given as an array or a single number | ❌ | undefined |
failOffsetY | number | number[] | When the finger moves outside this range (in points) along Y axis and gesture hasn't yet activated it will fail recognizing the gesture. Range can be given as an array or a single number | ❌ | undefined |
heartbeat | boolean | When 'heartbeat' is set to true, the progress bar color will animate back and forth between its current color and the color specified for the heartbeat. useful for loading state | ❌ | undefined |
FAQs
Slider component written using Reanimated v2, high performance/fps, also be used for video/audio scrubber.
We found that react-native-awesome-slider demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.