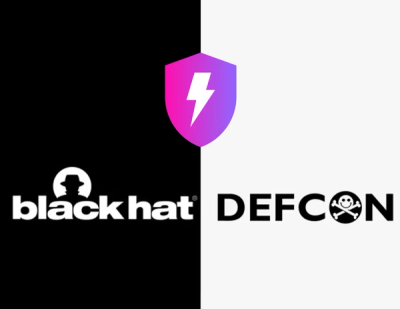
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
react-native-codegen
Advanced tools
The react-native-codegen package is a tool used in the React Native ecosystem to generate native code for JavaScript components and modules. It helps in creating type-safe interfaces between JavaScript and native code, ensuring that the data passed between them is correctly typed and validated.
Generating Native Modules
This feature allows you to define a native module in a JSON format, which react-native-codegen will then use to generate the corresponding native code. This ensures type safety and consistency between JavaScript and native code.
```json
{
"name": "MyModule",
"type": "module",
"methods": {
"myMethod": {
"type": "function",
"params": [
{ "name": "param1", "type": "string" },
{ "name": "param2", "type": "number" }
],
"returnType": "void"
}
}
}
```
Generating Native Components
This feature allows you to define a native component in a JSON format, which react-native-codegen will then use to generate the corresponding native code. This ensures that the component's props are correctly typed and validated.
```json
{
"name": "MyComponent",
"type": "component",
"props": {
"title": { "type": "string" },
"count": { "type": "number" }
}
}
```
Generating Event Emitters
This feature allows you to define an event emitter in a JSON format, which react-native-codegen will then use to generate the corresponding native code. This ensures that the events and their data are correctly typed and validated.
```json
{
"name": "MyEventEmitter",
"type": "eventEmitter",
"events": {
"onEvent": {
"type": "function",
"params": [
{ "name": "eventData", "type": "object" }
]
}
}
}
```
The react-native-create-library package is used to create a new native module library for React Native. It provides a boilerplate for creating native modules but does not offer the same level of type safety and code generation as react-native-codegen.
The react-native-builder-bob package is a CLI tool for creating and building React Native libraries. It focuses on setting up the project structure and build configuration but does not provide code generation capabilities like react-native-codegen.
yarn add --dev react-native-codegen
Note: We're using yarn
to install deps. Feel free to change commands to use npm
3+ and npx
if you like
v0.70.7
POST_NOTIFICATIONS
and deprecate POST_NOTIFICATION
(b5280bbc93 by @dcangulo)FAQs
⚛️ Code generation tools for React Native
The npm package react-native-codegen receives a total of 277,792 weekly downloads. As such, react-native-codegen popularity was classified as popular.
We found that react-native-codegen demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.