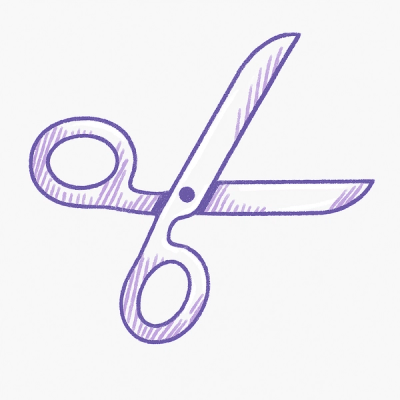
Security News
Knip Hits 500 Releases with v5.62.0, Improving TypeScript Config Detection and Plugin Integrations
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
react-native-file-viewer
Advanced tools
Native file viewer for react-native. Preview any type of file supported by the mobile device.
iOS: it uses QuickLook Framework
Android: it uses ACTION_VIEW
Intent to start the default app associated with the specified file.
Windows: Start the default app associated with the specified file.
$ npm install react-native-file-viewer --save
or
$ yarn add react-native-file-viewer
# RN >= 0.60
cd ios && pod install
# RN < 0.60
react-native link react-native-file-viewer
If your app is targeting Android 11 (API level 30) or newer, the following extra step is required, as described in Declaring package visibility needs and Package visibility in Android 11.
Specifically:
If your app targets Android 11 or higher and needs to interact with apps other than the ones that are visible automatically, add the element in your app's manifest file. Within the element, specify the other apps by package name, by intent signature, or by provider authority, as described in the following sections.
For example, if you know upfront that your app is supposed to open PDF files, the following lines should be added to your AndroidManifest.xml
.
...
</application>
+ <queries>
+ <intent>
+ <action android:name="android.intent.action.VIEW" />
+ <!-- If you don't know the MIME type in advance, set "mimeType" to "*/*". -->
+ <data android:mimeType="application/pdf" />
+ </intent>
+ </queries>
</manifest>
IMPORTANT: Try to be as granular as possible when defining your own queries. This might affect your Play Store approval, as mentioned in Package visibility filtering on Android.
If you publish your app on Google Play, your app's use of this permission is subject to approval based on an upcoming policy.
If your project is based on Expo, you need to eject your project by switching to the Bare workflow, in order to use this library.
Add the following to your Podfile:
pod 'RNFileViewer', :path => '../node_modules/react-native-file-viewer'
Libraries
➜ Add Files to [your project's name]
node_modules
➜ react-native-file-viewer
and add RNFileViewer.xcodeproj
libRNFileViewer.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)android/app/src/main/java/[...]/MainApplication.java
import com.vinzscam.reactnativefileviewer.RNFileViewerPackage;
to the imports at the top of the filenew RNFileViewerPackage()
to the list returned by the getPackages()
methodAppend the following lines to android/settings.gradle
:
include ':react-native-file-viewer'
project(':react-native-file-viewer').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-file-viewer/android')
Insert the following lines inside the dependencies block in android/app/build.gradle
:
compile project(':react-native-file-viewer')
Locate react-native-file-viewer
inside node_modules
folder and copy android/src/main/res/xml/file_viewer_provider_paths.xml
to your project res/xml/
directory
Add the following lines to AndroidManifest.xml
between the main <application></application>
tag:
...
<application>
...
<provider
android:name="com.vinzscam.reactnativefileviewer.FileProvider"
android:authorities="${applicationId}.provider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_viewer_provider_paths"
/>
</provider>
</application>
....
Follow the instructions in the 'Linking Libraries' documentation on the react-native-windows GitHub repo. For the first step of adding the project to the Visual Studio solution file, the path to the project should be ../node_modules/react-native-file-viewer/windows/RNFileViewer/RNFileViewer.csproj
.
import FileViewer from "react-native-file-viewer";
const path = FileViewer.open(path) // absolute-path-to-my-local-file.
.then(() => {
// success
})
.catch((error) => {
// error
});
import FileViewer from "react-native-file-viewer";
import DocumentPicker from "react-native-document-picker";
try {
const res = await DocumentPicker.pick({
type: [DocumentPicker.types.allFiles],
});
await FileViewer.open(res.uri);
} catch (e) {
// error
}
import FileViewer from "react-native-file-viewer";
import ImagePicker from "react-native-image-crop-picker";
ImagePicker.openPicker({})
.then((image) => FileViewer.open(image.path))
.catch((error) => {
// error
});
import FileViewer from "react-native-file-viewer";
const path = FileViewer.open(path, { showOpenWithDialog: true }) // absolute-path-to-my-local-file.
.then(() => {
// success
})
.catch((error) => {
// error
});
Since the library works only with absolute paths and Android assets folder doesn't have any absolute path, the file needs to be copied first. Use copyFileAssets of react-native-fs.
Example (using react-native-fs):
import FileViewer from "react-native-file-viewer";
import RNFS from "react-native-fs";
const file = "file-to-open.doc"; // this is your file name
// feel free to change main path according to your requirements
const dest = `${RNFS.DocumentDirectoryPath}/${file}`;
RNFS.copyFileAssets(file, dest)
.then(() => FileViewer.open(dest))
.then(() => {
// success
})
.catch((error) => {
/* */
});
No function about file downloading has been implemented in this package. Use react-native-fs or any similar library for this purpose.
Example (using react-native-fs):
import RNFS from "react-native-fs";
import FileViewer from "react-native-file-viewer";
import { Platform } from "react-native";
const url =
"https://github.com/vinzscam/react-native-file-viewer/raw/master/docs/react-native-file-viewer-certificate.pdf";
// *IMPORTANT*: The correct file extension is always required.
// You might encounter issues if the file's extension isn't included
// or if it doesn't match the mime type of the file.
// https://stackoverflow.com/a/47767860
function getUrlExtension(url) {
return url.split(/[#?]/)[0].split(".").pop().trim();
}
const extension = getUrlExtension(url);
// Feel free to change main path according to your requirements.
const localFile = `${RNFS.DocumentDirectoryPath}/temporaryfile.${extension}`;
const options = {
fromUrl: url,
toFile: localFile,
};
RNFS.downloadFile(options)
.promise.then(() => FileViewer.open(localFile))
.then(() => {
// success
})
.catch((error) => {
// error
});
open(filepath: string, options?: Object): Promise<void>
Parameter | Type | Description |
---|---|---|
filepath | string | The absolute path where the file is stored. The file needs to have a valid extension to be successfully detected. Use react-native-fs constants to determine the absolute path correctly. |
options (optional) | Object | Some options to customize the behaviour. See below. |
Parameter | Type | Description |
---|---|---|
displayName (optional) | string | Customize the QuickLook title (iOS only). |
onDismiss (optional) | function | Callback invoked when the viewer is being dismissed (iOS and Android only). |
showOpenWithDialog (optional) | boolean | If there is more than one app that can open the file, show an Open With dialogue box (Android only). |
showAppsSuggestions (optional) | boolean | If there is not an installed app that can open the file, open the Play Store with suggested apps (Android only). |
The library supports Android X and React Native 0.60+.
If you're using React Native < 0.60, please append the following snippet to your android/app/build.gradle
file:
preBuild.doFirst {
ant.replaceregexp(match:'androidx.core.content.', replace:'android.support.v4.content.', flags:'g', byline:true) {
fileset(dir: '../../node_modules/react-native-file-viewer/android/src/main/java/com/vinzscam/reactnativefileviewer', includes: '*.java')
}
}
If you prefer to not touch your gradle file, you can still use version 1.0.15
which is perfectly compatible.
FAQs
Native file viewer for react-native
The npm package react-native-file-viewer receives a total of 60,391 weekly downloads. As such, react-native-file-viewer popularity was classified as popular.
We found that react-native-file-viewer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.