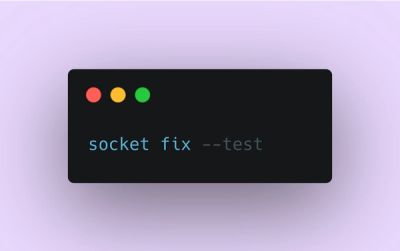
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
react-native-mmkv
Advanced tools
The fastest key/value storage for React Native. ~30x faster than AsyncStorage! Works on Android, iOS and Web.
react-native-mmkv is a fast, small, and easy-to-use key-value storage library for React Native. It leverages Facebook's MMKV storage library to provide efficient and secure storage solutions for mobile applications.
Basic Key-Value Storage
This feature allows you to store and retrieve basic key-value pairs. The code sample demonstrates how to set and get a string value using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const MMKV = new MMKVStorage.Loader().initialize();
// Set a value
MMKV.setString('username', 'john_doe');
// Get a value
const username = MMKV.getString('username');
console.log(username); // Output: john_doe
Object Storage
This feature allows you to store and retrieve objects. The code sample demonstrates how to set and get an object using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const MMKV = new MMKVStorage.Loader().initialize();
const user = { name: 'John Doe', age: 30 };
// Set an object
MMKV.setMap('user', user);
// Get an object
const storedUser = MMKV.getMap('user');
console.log(storedUser); // Output: { name: 'John Doe', age: 30 }
Encryption
This feature allows you to store data securely using encryption. The code sample demonstrates how to set and get an encrypted string value using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const MMKV = new MMKVStorage.Loader().withEncryption().initialize();
// Set a value
MMKV.setString('secret', 'my_secret_value');
// Get a value
const secret = MMKV.getString('secret');
console.log(secret); // Output: my_secret_value
Multi-Instance Support
This feature allows you to create multiple instances of storage, which can be useful for separating different types of data. The code sample demonstrates how to set and get values from different instances using MMKV.
import MMKVStorage from 'react-native-mmkv-storage';
const userStorage = new MMKVStorage.Loader().withInstanceID('user').initialize();
const settingsStorage = new MMKVStorage.Loader().withInstanceID('settings').initialize();
// Set values in different instances
userStorage.setString('username', 'john_doe');
settingsStorage.setBool('darkMode', true);
// Get values from different instances
const username = userStorage.getString('username');
const darkMode = settingsStorage.getBool('darkMode');
console.log(username); // Output: john_doe
console.log(darkMode); // Output: true
react-native-async-storage is a simple, unencrypted, asynchronous, persistent, key-value storage system that is global to the app. It is more widely used and has a larger community but lacks the performance and encryption features of react-native-mmkv.
react-native-sensitive-info provides secure storage for sensitive data, such as login credentials. It uses the device's secure storage mechanisms (Keychain on iOS and Keystore on Android). While it offers strong security, it may not be as fast as react-native-mmkv for general key-value storage.
redux-persist is a library used to persist and rehydrate a Redux store. It supports various storage backends, including AsyncStorage. While it is not a direct competitor, it can be used for state persistence in applications using Redux, offering more flexibility in terms of storage backends.
The fastest key/value storage for React Native. ~30x faster than AsyncStorage!
See README.md for more information.
FAQs
The fastest key/value storage for React Native. ~30x faster than AsyncStorage! Works on Android, iOS and Web.
The npm package react-native-mmkv receives a total of 231,445 weekly downloads. As such, react-native-mmkv popularity was classified as popular.
We found that react-native-mmkv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.