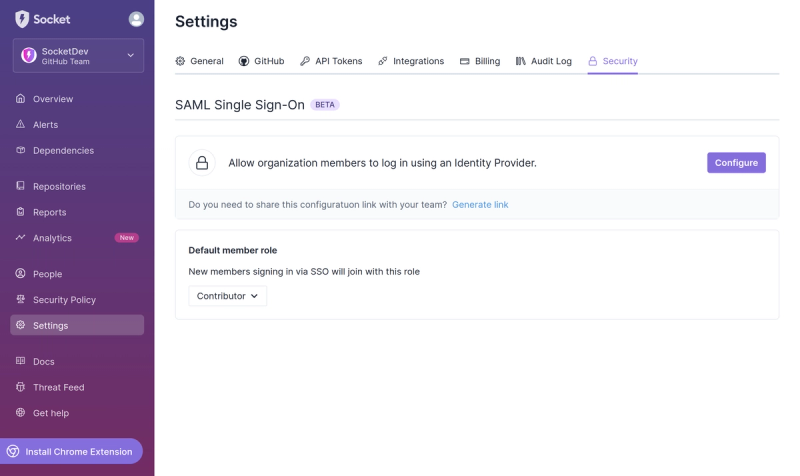
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
react-native-qrcode-package
Advanced tools
This package include QR code generator and QR code Scanner for React Native.
Readme
QR Code generator and QR Code Scanner for React Native.
npm i react-native-qrcode-package
Install dependency packages to generate qr code:
yarn add react-native-svg
Or
npm i react-native-svg
Install dependency packages to scan qr code:
yarn add react-native-camera
yarn add react-native-permissions
Or
npm i react-native-camera
npm i react-native-permissions
IMPORTANT New version of react-native-permissions (3.8.0) has breaking changes and doesn't work correctly on ios with camera permission. Use "react-native-permissions": "3.7.3" or below version.
After that for ios go to the folder your-project/ios and run pod install
.
WARNING
You may got this warn after install react-native-camera: ViewPropTypes will be removed from React Native. Migrate to ViewPropTypes exported from 'deprecated-react-native-prop-types'
. If you have warn then: go to the node-modules
folder -> find react-native-camera
folder -> src
folder -> find the file RNCamera.js
-> find inside the file: import ViewPropTypes from from 'react-native';
-> delete this import from 'react-native'
-> create new import: import {ViewPropTypes} from 'deprecated-react-native-prop-types';
This is one of the solutions to this warn.
With iOS 10 and higher you need to add the "Privacy - Camera Usage Description" key to the Info.plist of your project. This should be found in 'your_project/ios/your_project/Info.plist'
. Add the following code:
<key>NSCameraUsageDescription</key>
<string>Your message to user when the camera is accessed for the first time</string>
Also you will need to add the following code to your Podfile (as part of the react-native-permissions setup):
permissions_path = '../node_modules/react-native-permissions/ios'
pod 'Permission-Camera', :path => "#{permissions_path}/Camera"
You may also need to reset your simulator data after adding those permissions Device -> Erase All
With Android 7 and higher you need to add the "Vibration" permission to your AndroidManifest.xml of your project if you want to use vibration after a successful scan. This should be found in your android/app/src/main/AndroidManifest.xml
Add the following:
<uses-permission android:name="android.permission.VIBRATE"/>
You need to add the "missingDimensionStrategy" config for the 'react-native-camera' setting to 'general', this should be found in your android/app/build.gradle
add the following:
android {
...
defaultConfig {
...
missingDimensionStrategy 'react-native-camera', 'general' <-- insert this line
}
}
import GenerateQRCode from 'react-native-qrcode-package';
// Simple usage(all fields have default values except 'jsonObject')
render() {
return (
<View>
<GenerateQRCode
jsonObject={data} // data - it's your json file(data.json)
sizeQRcode={200}
/>
</View>
);
}
// error handle
handleError = (error: undefined) => {
console.error('Something went wrong', error);
};
render() {
return (
<View>
<GenerateQRCode
jsonObject={data}
onError={e => handleError(e)}
/>
</View>
);
}
import ScanQRCode from 'react-native-qrcode-package';
// Simple usage(all fields have default values except 'onQRCodeScanned')
handleScanJsonObject = (json: object) => {
console.log('QR Code data:', json);
};
render() {
return (
<View>
<ScanQRCode
onQRCodeScanned={handleScanJsonObject}
/>
</View>
);
}
// error handle
handleError = (error: undefined) => {
console.error('Something went wrong', error);
Alert.alert(
'Invalid QR Code',
'The scanned QR code does not contain valid JSON',
[
{
text: 'OK',
onPress: () => {},
},
],
);
};
render() {
return (
<View>
<ScanQRCode
onQRCodeScanned={handleScanJsonObject}
onError={handleError}
/>
</View>
);
}
Name | Default | Description |
---|---|---|
sizeQRCode | 150 | Size of rendered image in pixels |
jsonObject | object | Put your a JSON .json file of the QR code. Example: '{"brand": "Name","model": "Model name"}' |
colorQrCode | 'black' | Color of the QR code |
onError(error) | undefined | Callback fired when exception happened during the code generating process |
backgroundColorQRCode | 'white' | Color of the background QR code |
enableLinearGradient | false | Enable or disable linear gradient |
linearGradient | ['rgb(255,0,0)','rgb(0,255,255)'] | Array of 2 rgb colors used to create the linear gradient |
gradientDirection | [170,0,0,0] | The direction of the linear gradient |
logo | null | Image source object. Ex. {uri: 'base64string'} or {require('pathToImage')} |
logoSize | 20% of size | Size of the imprinted logo. Bigger logo equals less error correction in QR code |
logoBackgroundColor | 'transparent' | The logo gets a filled quadratic background with this color. Use 'transparent' if your logo already has its own backdrop. |
logoMargin | 2 | Logo distance to it's wrapper |
logoBorderRadius | 0 | Border-radius of logo image (Android isn't supported) |
quietZone | 0 | Quiet zone around the qr in pixels (Useful when saving image to gallery) |
getRef | null | Get SVG ref for further usage |
ecl | 'M' | Error correction level |
Prop | Type |
---|---|
sizeQRCode | number |
jsonObject | object |
colorQrCode | string |
onError(error) | Function |
backgroundColorQRCode | string |
enableLinearGradient | boolean |
linearGradient | string [] |
gradientDirection | string [] |
logo | ImageSourcePropType |
logoSize | number |
logoBackgroundColor | string |
logoMargin | number |
logoBorderRadius | number |
quietZone | number |
getRef(c: any) => any | Function |
ecl | 'L' or 'M' or 'Q' or 'H' |
Name | Default | Description |
---|---|---|
onQRCodeScanned(object) | - | Will call this specified method when a QR code is detected in the camera. |
vibrateAfterScan | false | Vibrate after Scanned QR |
reactivateCamera | true | Will reactivate the scanning ability of the component. |
reactivateScanTimeout | 0 | Use this to configure how long it should take (in milliseconds) before the ScanQRCode should reactivate. |
fadeImageIn | true | When set to true this ensures that the camera view fades in after the initial load up instead of being rendered immediately. Set this to false to prevent the animated fade in of the camera view. |
showScanMarker | false | Use this to show marker on the camera scanning window. |
cameraType | 'back' | Use this to control which camera to use for scanning QR codes.(propType: ['front', 'back']) |
customMarker | - | Pass a RN element/component to use it as a custom marker. |
containerStyle | - | Use this to pass styling for the outermost container. |
cameraStyle | - | Use this to pass or overwrite styling for the camera window rendered. |
cameraContainerStyle | - | Use this to pass or overwrite styling for the camera container (view) window rendered. |
markerStyle | - | Use this to add custom styling to the default marker. |
topViewStyle | - | Use this to pass or overwrite styling for the '' that contains the topContent prop. |
bottomViewStyle | - | Use this to pass or overwrite styling for the '' that contains the bottomContent prop. |
topContent | - | Use this to render any additional content at the top of the camera view.(propType: oneOfType([ PropTypes.element, PropTypes.string, ])) |
bottomContent | - | Use this to render any additional content at the bottom of the camera view.(propType: oneOfType([ PropTypes.element, PropTypes.string, ])) |
notAuthorizedView | - | Pass a RN element/component to use it when no permissions given to a camera (only IOS). |
permissionDialogTitle | 'Permission' | Use this to set permission dialog title (only Android).(propType: string) |
permissionDialogMessage | 'Requires camera permission to scan' | Use this to set permission dialog message (only Android).(propType: string) |
buttonPositive | 'OK' | Use this to set permission dialog button text (only Android).(propType: string) |
checkAndroid6Permissions | false | Use this to enable permission checking on Android 6. |
lightMode | RNCamera.Constants.FlashMode.off | Part of the react-native-camera package react-native-camera/flashMode:RNCamera.Constants.FlashMode.off (turns it off) / RNCamera.Constants.FlashMode.on(means camera will use flash in all photos taken) / RNCamera.Constants.FlashMode.auto(leaves your phone to decide when to use flash when taking photos, based on the lightning conditions that the camera observes) / RNCamera.Constants.FlashMode.torch(turns on torch mode, meaning the flash light will be turned on all the time (even before taking photo) just like a flashlight). |
onError(error: Error) | 'this is handling errors that occurred during scanning' | Callback fired when exception happened during the scanning process |
cameraScanTimeout | 0 | Use this to configure how long it should take (in milliseconds) before the ScanQRCode should be displayed. After that the camera will be inactive and press the view to reactivate it. |
cameraProps | - | Properties to be passed to the Camera component.(prop type: object) |
cameraTimeoutView | - | Pass an RN element/component to show it when the camera is inactive for cameraScanTimeout (another prop) milliseconds. If the cameraScanTimeout is 0 or not specified, this prop will never be used. |
Prop | Type |
---|---|
onQRCodeScanned(json: object) => void | Function |
vibrateAfterScan | boolean |
reactivateCamera | boolean |
reactivateScanTimeout | number |
fadeImageIn | boolean |
showScanMarker | boolean |
cameraType | 'front' |
customMarker | JSX.Element |
containerStyle | StyleProp |
cameraStyle | StyleProp |
cameraContainerStyle | StyleProp |
markerStyle | StyleProp |
topViewStyle | StyleProp |
bottomViewStyle | StyleProp |
topContent | JSX.Element or string |
bottomContent | JSX.Element or string |
notAuthorizedView | JSX.Element |
permissionDialogTitle | string |
permissionDialogMessage | string |
buttonPositive | string |
checkAndroid6Permissions | boolean |
lightMode | RNCamera.Constants.FlashMode.off or RNCamera.Constants.FlashMode.on or RNCamera.Constants.FlashMode.auto or RNCamera.Constants.FlashMode.torch |
onError(error: undefined) => void | Function |
cameraScanTimeout | number |
cameraProps | RNCameraProps |
cameraTimeoutView | JSX.Element |
FAQs
This package include QR code generator and QR code Scanner for React Native.
The npm package react-native-qrcode-package receives a total of 4 weekly downloads. As such, react-native-qrcode-package popularity was classified as not popular.
We found that react-native-qrcode-package demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.