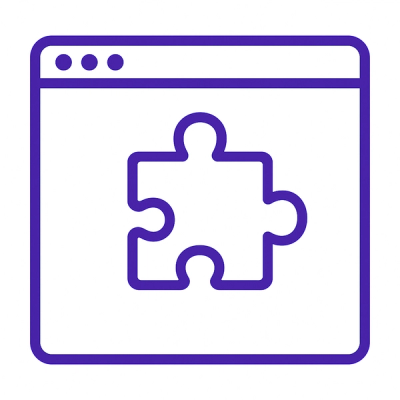
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
react-native-reanimated-carousel
Advanced tools
Simple carousel component.fully implemented using Reanimated 2.Infinitely scrolling, very smooth.
react-native-reanimated-carousel is a highly customizable and performant carousel component for React Native, leveraging the power of Reanimated 2 for smooth animations.
Basic Carousel
This code demonstrates a basic carousel with three items. The carousel is rendered using the `react-native-reanimated-carousel` package, and each item is displayed within a styled container.
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
import Carousel from 'react-native-reanimated-carousel';
const data = ['Item 1', 'Item 2', 'Item 3'];
const BasicCarousel = () => {
return (
<Carousel
width={300}
height={200}
data={data}
renderItem={({ item }) => (
<View style={styles.itemContainer}>
<Text>{item}</Text>
</View>
)}
/>
);
};
const styles = StyleSheet.create({
itemContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#fff',
borderRadius: 8,
shadowColor: '#000',
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.8,
shadowRadius: 2,
elevation: 1,
},
});
export default BasicCarousel;
Custom Animation
This code demonstrates a carousel with custom animations. The `customAnimation` prop is used to define a custom animation for the carousel items, and the `animationConfig` prop is used to configure the animation's easing and duration.
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
import Carousel from 'react-native-reanimated-carousel';
import { Easing } from 'react-native-reanimated';
const data = ['Item 1', 'Item 2', 'Item 3'];
const CustomAnimationCarousel = () => {
return (
<Carousel
width={300}
height={200}
data={data}
renderItem={({ item }) => (
<View style={styles.itemContainer}>
<Text>{item}</Text>
</View>
)}
customAnimation={(value) => ({
transform: [{ scale: value }],
opacity: value,
})}
animationConfig={{
easing: Easing.inOut(Easing.ease),
duration: 500,
}}
/>
);
};
const styles = StyleSheet.create({
itemContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#fff',
borderRadius: 8,
shadowColor: '#000',
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.8,
shadowRadius: 2,
elevation: 1,
},
});
export default CustomAnimationCarousel;
Looping Carousel
This code demonstrates a looping carousel. The `loop` prop is set to true, which makes the carousel items loop infinitely.
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
import Carousel from 'react-native-reanimated-carousel';
const data = ['Item 1', 'Item 2', 'Item 3'];
const LoopingCarousel = () => {
return (
<Carousel
width={300}
height={200}
data={data}
renderItem={({ item }) => (
<View style={styles.itemContainer}>
<Text>{item}</Text>
</View>
)}
loop
/>
);
};
const styles = StyleSheet.create({
itemContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#fff',
borderRadius: 8,
shadowColor: '#000',
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.8,
shadowRadius: 2,
elevation: 1,
},
});
export default LoopingCarousel;
react-native-snap-carousel is a popular package for creating carousels in React Native. It offers a wide range of customization options and supports both horizontal and vertical carousels. Compared to react-native-reanimated-carousel, it does not leverage Reanimated 2 for animations, which may result in less smooth animations.
react-native-swiper is another widely used package for creating carousels and swipers in React Native. It provides a simple API and supports various features like autoplay, pagination, and custom animations. However, it may not offer the same level of performance and smoothness as react-native-reanimated-carousel, which uses Reanimated 2.
react-native-looped-carousel is a package designed for creating looping carousels in React Native. It is easy to use and supports both horizontal and vertical carousels. While it is a good option for basic carousels, it may lack some of the advanced customization and animation capabilities provided by react-native-reanimated-carousel.
MIT
FAQs
Simple carousel component.fully implemented using Reanimated 2.Infinitely scrolling, very smooth.
The npm package react-native-reanimated-carousel receives a total of 216,279 weekly downloads. As such, react-native-reanimated-carousel popularity was classified as popular.
We found that react-native-reanimated-carousel demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.