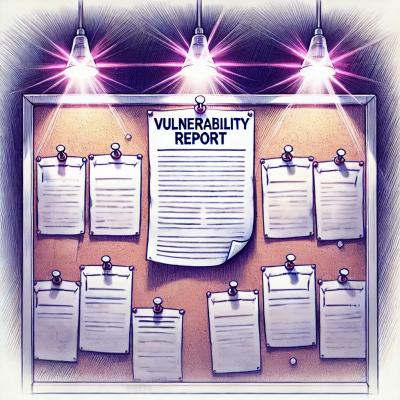
Security News
libxml2 Maintainer Ends Embargoed Vulnerability Reports, Citing Unsustainable Burden
Libxml2’s solo maintainer drops embargoed security fixes, highlighting the burden on unpaid volunteers who keep critical open source software secure.
react-native-trusted-device
Advanced tools
Trusded device library for react native from Fazpass company
npm install react-native-trusted-device
First you should call this code in your root view. It will save that credential in then local storage of the device.
import { initialize } from 'react-native-trusted-device';
// ...
Fazpass.initialize("eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZGVudGlmaWVyIjo0fQ.WEV3bCizw9U_hxRC6DxHOzZthuJXRE8ziI3b6bHUpEI",
1,(err:string)=>{
console.log(err)
// TODO when initialize status false & true
})
For enjoy of all feature make sue your app request this permission
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.READ_PHONE_NUMBERS" />
<uses-permission android:name="android.permission.READ_CONTACTS" />
<uses-permission android:name="android.permission.USE_BIOMETRIC" />
<uses-permission android:name="android.permission.RECEIVE_SMS" />
<uses-permission android:name="android.permission.READ_CALL_LOG" />
If you feel lazy to take care about it you can call this method
import { requestPermission } from 'react-native-trusted-device';
requestPermission()
If you want to listen incoming OTP by SMS or Misscall you need to call streamOtp
and close this stream on component will unmount
React.useEffect(() => {
streamOtp((otp:string)=>{
// Your OTP come here
})
return()=>{
closeStreamNotification()
}
}, [])
Request OTP that use phone number as the parameter
Fazpass.requestOtpByPhone(phone,
// This is your gateway
"1e1de010-71b2-47d6-a037-254182ff3696",
(_response:any)=>{
// This otp id will be use for validate that OTP
setOtpId(_response.id)
},
(_err:string)=>{
ToastAndroid.show(_err, ToastAndroid.SHORT);
})
If you want email as the parameter you can call requestOtpByEmail
This is example for validation OTP
Fazpass.validateOtp(otpId, otp,
(status:boolean)=>{
// validation status
setVerifyStatus("Verification status is: "+ status)
},
(err:string)=>{
})
Header enrichment only accept phone number as a parameter
Fazpass.heValidation(phone,
"6cb0b024-9721-4243-9010-fd9e386157ec",
(status:boolean)=>{
setHeMessage('Result of He valisation is ' + status)
},
(err:string)=>{
setHeMessage(err)
})
Available Method:
// you just need to fill one of them phone/email
Fazpass.checkDevice('PHONE_NUMBER', 'EMAIL',
(status:any)=>{
// CD status os cross device status, and TD is trusted Device
setCheckStatus(`Status cross device : ${status.cd} Status trusted device : ${status.td}`)
},
(err:string)=>{
ToastAndroid.show(err, 1)
})
Note: You can't call enroll or validate if you have not call check
const user = {
"email":"",
"phone":phone,
"name":"Andri nova riswanto",
"id_card":"",
"address":"Bogor"
}
Fazpass.enrollDeviceByFinger(user, "PIN", (status:boolean)=>{
ToastAndroid.show(`Enroll status ${status}`,1)
},
(err:string)=>{
ToastAndroid.show(err, 1)
})
// or you can enroll device by pin only
Fazpass.enrollDeviceByPin(user, "PIN", (status:boolean)=>{
ToastAndroid.show(`Enroll status ${status}`,1)
},
(err:string)=>{
ToastAndroid.show(err, 1)
})
Note: Don't enroll device if trusted device status is false, so better you validate this user & device first using OTP or Header enrichment
Fazpass.validateUser("PIN", (response:any)=>{
ToastAndroid.show(`Your confidence rate is : ${response.summary}`,1)
}, (err:string)=>{
ToastAndroid.show(`Something went wrong cause : ${err}`,1)
})
Fazpass.removeDevice('PIN',
(status:any)=>{
ToastAndroid.show(`Remove status ${status}`, 1)
},
(err:string)=>{
ToastAndroid.show(err, 1)
})
When check device there are 2 status (Trusted Device & Cross Device) so it is possible when trusted device is false
but cross device status is true
.
You can call method cross device to send notification that will request validation, It will make you no need to validate OTP or Header Enrichment
// make it true if you want validate cross device using pin
React.useEffect(() => {
Fazpass.initializeCrossDevice(false)
}, [])
React.useEffect(() => {
Fazpass.initializeCrossDeviceNonView(false, (onNotif:string)=>{
//this is state
setNotificated(true)
let a = onNotif.split(',')
//this is state
setNotificationMessage(`There was an intruder that try to login \n from device ${a[1]}`)
}, (onRunning:string)=>{
console.log(onRunning)
})
// Call this method also to handle foreground notification
streamNotification((device:string)=>{
//this is state
setNotificated(true)
let a = device.split(',')
//this is state
setNotificationMessage(`There was an intruder that try to login \n from device ${a[1]}`)
})
return()=>{
// You need to close the stream
closeStreamNotification()
}
}, [])
Note: you need to call this method in the first view on your app
We use bridging between native code and react so if you want to know what error that happen you can listen with call function
streamNativeError((data)=>{
// do some thing
})
and dont forget to close it when component willunmount
closeStreamNativeError()
We use native library so make sure you disable shrinkResource in gradle
buildTypes {
release {
minifyEnabled false
shrinkResources false
}
}
MIT
FAQs
test
The npm package react-native-trusted-device receives a total of 5 weekly downloads. As such, react-native-trusted-device popularity was classified as not popular.
We found that react-native-trusted-device demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Libxml2’s solo maintainer drops embargoed security fixes, highlighting the burden on unpaid volunteers who keep critical open source software secure.
Research
Security News
Socket investigates hidden protestware in npm packages that blocks user interaction and plays the Ukrainian anthem for Russian-language visitors.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.