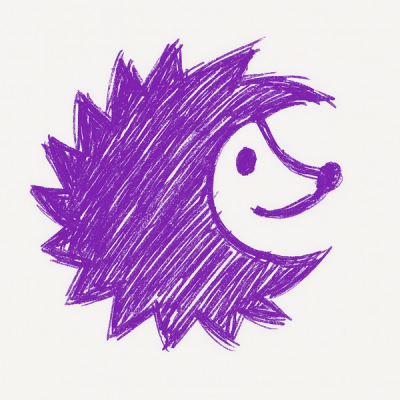
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
react-native-wordpress-editor
Advanced tools
React Native Wrapper for WordPress Rich Text Editor. The WordPress-Editor is the text editor used in the official WordPress mobile apps to create and edit pages & posts
React Native Wrapper for WordPress Rich Text Editor. The WordPress-Editor is the text editor used in the official WordPress mobile apps to create and edit pages & posts. In short it's a simple, straightforward way to visually edit HTML.
native code for the original editor (a git submodule) - actually taken from this leaner fork
react-native-navigation - native navigation library for React Native (required to natively display the editor within RN)
It isn't trivial to intergrate the WordPress editor with React Native because it is exposed in native code as a UIViewController
and not a UIView
. React Native only has internal support for integrating native UIViews
by wrapping them as React components.
In order to integrate a UIViewController
with RN, we have to turn to the library react-native-navigation which fully supports native app skeletons with View Controllers. If you're interested in how it's achieved, take a look at the following internal dependency of this awesome library.
Make sure your project relies on React Native >= 0.25
Make sure your project uses react-native-navigation and that you've followed the Installation instructions there
In your RN project root run:npm install react-native-wordpress-editor --save
Open your Xcode project and drag the folder node_modules/react-native-wordpress-editor/ios
into your project
First, create a placeholder screen for the editor. The main purpose of this screen is to handle navigation events. See an example here.
Note: Make sure your screen component has been registered with
Navigation.registerComponent
like all react-native-navigation screens need to be, example.
Now, to display your screen, from within one of your other app screens, push the editor:
this.props.navigator.push({
screen: 'example.EditorScreen',
title: 'Preview',
passProps: {
externalNativeScreenClass: 'RNWordPressEditorViewController',
externalNativeScreenProps: {
// the post to open in the editor, leave empty for no post
post: {
// title of the post
title: 'Hello WorldPress',
// html body of the post
body: 'cool HTML body <br><br> <img src="https://www.wpshrug.com/wp-content/uploads/2016/05/wordpress-winning-meme.jpg" />'
},
// if no post shown, these placeholders will appear in the relevant fields
placeHolders: {
title: 'title',
body: 'body'
}
}
}
});
Once the editor screen is displayed, you can communicate with it using a JS interface.
import EditorManager from 'react-native-wordpress-editor';
EditorManager.setEditingState(editing: boolean)
Switch between editing and preview modes (accepts a boolean).
EditorManager.resetStateToInitial()
Reset to the initial state right after the screen was pushed (with original props).
EditorManager.isPostChanged(): Promise<boolean>
Returns a promise of a boolean (since it's async) whether the state is still the initial one.
EditorManager.getPostData(): Promise<{title: string, body: string}>
Returns a promise of a simple object holding the title
and HTML body
of the post.
EditorManager.addImages(images: Array<{url: string}>)
Adds images at the current cursor location in the editor, takes an array of simple objects with the url
of each image.
This project depends on:
See LICENSE
FAQs
React Native Wrapper for WordPress Rich Text Editor. The WordPress-Editor is the text editor used in the official WordPress mobile apps to create and edit pages & posts
The npm package react-native-wordpress-editor receives a total of 89 weekly downloads. As such, react-native-wordpress-editor popularity was classified as not popular.
We found that react-native-wordpress-editor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.