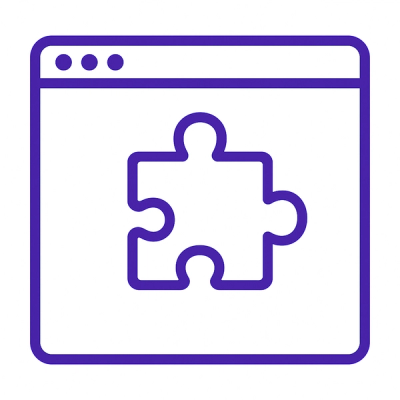
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
react-numeric-keyboard
Advanced tools
A numeric virtual keyboard for React. Especially for Progressive Web Applications and mobile views.
using npm
npm i react-numeric-keyboard
using yarn
yarn add react-numeric-keyboard
import { useState } from 'react';
import { NumericKeyboard } from 'react-numeric-keyboard';
function App() {
const [isOpen, setIsOpen] = useState(false);
const onChange = ({ value, name }) => {
console.log(value, name);
};
return <NumericKeyboard isOpen={isOpen} onChange={onChange} />;
}
props | Type | default | Description |
---|---|---|---|
isOpen | boolean | Required. Open or close the keyboard | |
onChange({value,name}) | VoidFunction | Required. Getting the total value and the name of each keyboard characters | |
mode | simple or spaced | simple | Keyboard's mode |
hasTransition | boolean | true | keyboard's opening and closing transition |
transitionTime | number | 300ms | keyboard's transition time. Only works if hasTransition prop is true. |
className | string | keyboard's classname | |
style | CSSProperties | keyboard's style | |
isKeyboardDisabled | boolean | false | Prevents keyboard's items from being clicked or touched |
backSpaceIcon | ReactNode | Custom backspace icon | |
leftIcon | ReactNode | Custom left corner icon | |
containerClassName | string | ClassName of the keyboard's items container | |
fullWidth | boolean | keyboard's container width | |
header | ReactNode | An optional header above the keyboard. Note that to prevent your header's overflow use box-sizing:border-box in your header component |
FAQs
A numeric keyboard for React
The npm package react-numeric-keyboard receives a total of 67 weekly downloads. As such, react-numeric-keyboard popularity was classified as not popular.
We found that react-numeric-keyboard demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.