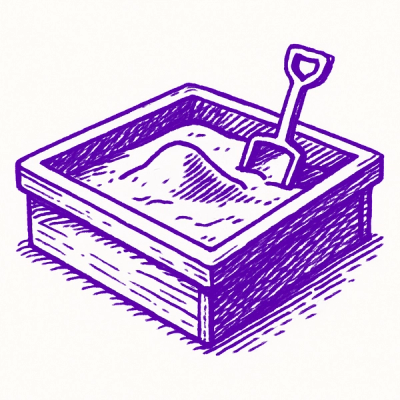
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
react-progress-stepper-ts
Advanced tools
React Progress Stepper
Minimal and beautiful stepper for React.
$ npm i react-progress-stepper-ts
$ yarn add react-progress-stepper-ts
import React from 'react';
import {
Stepper,
Step,
useStepper,
} from "react-progress-stepper-ts";
export default const App = () => {
const { step, incrementStep, decrementStep } = useStepper(0, 3);
return (
<>
<Stepper step={step}>
<Step></Step>
<Step></Step>
<Step></Step>
</Stepper>
<button onClick={decrementStep}>Prev</button>
<button onClick={incrementStep}>Next</button>
</>
)
}
Property | Type | Description |
---|---|---|
step | Integer | State to track the current step |
vertical | Boolean | Toggle vertical view |
dark | Boolean | Toggle dark mode |
numbered | Boolean | Toggle if each step is numbered or not |
theme | Object | Customize the appearance of the stepper |
Property | Type | Description |
---|---|---|
customContent | Function | Override step circle content |
You can customize the content of each step, as the example below:
import React from 'react';
import {
Stepper,
Step,
useStepper,
StepNumber,
StepTitle,
StepStatus,
StepDescription,
} from "react-progress-stepper-ts";
export default const App = () => {
const { step, incrementStep, decrementStep } = useStepper(0, 3);
return (
<>
<Stepper step={step}>
<Step>
<StepNumber />
<StepTitle>Title</StepTitle>
<StepStatus />
<StepDescription>Description</StepDescription>
</Step>
<Step>
<StepNumber />
<StepTitle>Title</StepTitle>
<StepStatus />
<StepDescription>Description</StepDescription>
</Step>
<Step>
<StepNumber />
<StepTitle>Title</StepTitle>
<StepStatus />
<StepDescription>Description</StepDescription>
</Step>
</Stepper>
</>
)
}
Property | Type | Description |
---|---|---|
text | String | Customize text |
StepTitle comes with no property, you can pass text as children.
Property | Type | Description |
---|---|---|
textProgress | String | Customize text |
textCompleted | String | Customize text |
textPending | String | Customize text |
StepDescription comes with no property, you can pass text as children.
Hook to handle the state of stepper easily, you could write your own logic to handle the state.
To work properly you need to provide two arguments to useStepper:
useStepper provides several utilities:
You can customize the appearance of the stepper in two ways:
{
light: {
step: {
pending: {
background: "#ededed",
color: "#a1a3a7",
},
progress: {
background: "#3c3fed",
color: "#ffffff",
},
completed: {
background: "#23c275",
color: "#ffffff",
},
},
content: {
pending: {
stepNumber: { color: "#a1a3a7" },
title: { color: "#a1a3a7" },
status: { background: "#f2f2f2", color: "#a1a3a7" },
description: { color: "#a1a3a7" },
},
progress: {
stepNumber: { color: "#131b26" },
title: { color: "#131b26" },
status: { background: "#e7e9fd", color: "#3c3fed" },
description: { color: "#131b26" },
},
completed: {
stepNumber: { color: "#131b26" },
title: { color: "#131b26" },
status: { background: "#e9faf2", color: "#23c275" },
description: { color: "#131b26" },
},
},
progressBar: {
pending: {
background: "#ededed",
},
progress: {
background: "#e7e9fd",
fill: "#3c3fed",
},
completed: {
background: "#e9faf2",
fill: "#23c275",
},
},
},
dark: {
step: {
pending: {
background: "#1a1a1a",
color: "#767676",
},
progress: {
background: "#19b6fe",
color: "#ffffff",
},
completed: {
background: "#23c275",
color: "#ffffff",
},
},
content: {
pending: {
stepNumber: { color: "#767676" },
title: { color: "#767676" },
status: { background: "#1a1a1a", color: "#767676" },
description: { color: "#767676" },
},
progress: {
stepNumber: { color: "#ece4d9" },
title: { color: "#ece4d9" },
status: { background: "#08374c", color: "#19b6fe" },
description: { color: "#ece4d9" },
},
completed: {
stepNumber: { color: "#ece4d9" },
title: { color: "#ece4d9" },
status: { background: "#0b3a23", color: "#23c275" },
description: { color: "#ece4d9" },
},
},
progressBar: {
pending: {
background: "#1a1a1a",
},
progress: {
background: "#08374c",
fill: "#19b6fe",
},
completed: {
background: "#0b3a23",
fill: "#23c275",
},
},
},
}
.step {
width: 3em;
height: 3em;
}
.step.progress {
background: #6ab04c;
}
React Progress Stepper is released under the MIT license, feel free to use it, share and modify.
FAQs
Unknown package
We found that react-progress-stepper-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.