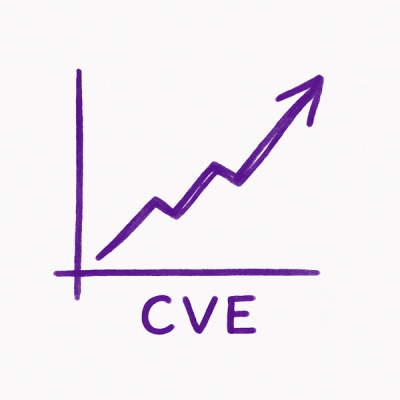
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
react-roulette
Advanced tools
A very simple (and buggy) React Roulette you can roll that I made in a couple of hours.
This is a very simple React component wich allows you to display an interactive roulette that can be thrown and it returns the selected value to be used.
You can use this component by installing with npm:
npm i react-roulette
Then import it in your app:
import React from 'react';
import Roulette from 'react-roulette';
const App = () => {
const items = ['Apple', 'Banana', 'Cherry'];
const colors = ['#F76156', '#FBD1A2', '#BED558'];
return (
<div className="App">
<h1>React Roulette</h1>
<Roulette items={items} colors={colors} />
</div>
);
}
export default App;
Required props:
items
- Array of items to display.colors
- Array of colors to use. If it's smaller tha than the items it's used in loop.Optional props:
frameColor
- Color for the frame and the arrow. Default: white
.centerColor
- Color for the center button. Default: #333
.image
- Url of an image to use inside the center button.clickSpeed
- Speed to rotate the roulette when the center button is clicked. Default: 50
.friction
- Friction applied to the rotation. Default: 0.005
.onChange
- Function triggered when the roulette stops. Returns the element where it landed as an argument.FAQs
A very simple (and buggy) React Roulette you can roll that I made in a couple of hours.
The npm package react-roulette receives a total of 3 weekly downloads. As such, react-roulette popularity was classified as not popular.
We found that react-roulette demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.