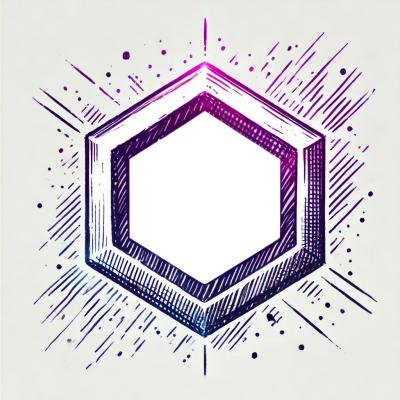
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
react-seed
Advanced tools
A boilerplate for building React apps with ES6 and webpack.
git clone --depth=1 https://github.com/badsyntax/react-seed.git my-project
npm install -g yo
npm install -g generator-react-seed
yo react-seed
npm start
- Build and start the app in dev mode at http://localhost:8000npm test
- Run the testsnpm run build
- Run a production build### Writing components:
// Filename: Menu.jsx
'use strict';
import './_Menu.scss';
import React from 'react';
import MenuItem from './MenuItem';
let { Component, PropTypes } = React;
export default class Menu extends Component {
static defaultProps = {
items: []
};
static propTypes = {
items: PropTypes.array.isRequired
};
render() {
return (
<ul className={'menu'}>
{this.props.items.map((item) => {
return (<MenuItem item={item} />);
}, this)}
</ul>
);
}
}
###Writing tests:
// Filename: __tests__/Menu-test.jsx
'use strict';
import React from 'react/addons';
import { expect } from 'chai';
import Menu from '../Menu.jsx';
import MenuItem from '../MenuItem.jsx';
// Here we create a mocked MenuItem component.
class MockedMenuItem extends MenuItem {
render() {
return (
<li className={'mocked-menu-item'}>{this.props.item.label}</li>
);
}
}
// Here we set the mocked MenuItem component.
Menu.__Rewire__('MenuItem', MockedMenuItem);
describe('Menu', () => {
let { TestUtils } = React.addons;
let menuItems = [
{ id: 1, label: 'Option 1' },
{ id: 2, label: 'Option 2' }
];
let menu = TestUtils.renderIntoDocument(
<Menu items={menuItems} />
);
let menuElem = React.findDOMNode(menu);
let items = menuElem.querySelectorAll('li');
it('Should render the menu items', () => {
expect(items.length).to.equal(2);
});
it('Should render the menu item labels', () => {
Array.prototype.forEach.call(items, (item, i) => {
expect(item.textContent.trim()).to.equal(menuItems[i].label);
});
})
it('Should render the mocked menu item', () => {
expect(menuElem.querySelectorAll('li')[0].className).to.equal('mocked-menu-item');
});
});
import
Sass and CSS files from within your JavaScript component files:
// Filename: app.jsx
import 'normalize.css/normalize.css';
import './scss/app.scss';
webpack/loaders.js
file.All required .html
files are compiled with lodash.template and synced into the ./build
directory:
// Filename: app.jsx
import './index.html';
webpack/loaders.js
file.var
. Use let
and const
.npm version patch
git push && git push --tags
npm login
(Optional)npm publish
This project was initially forked from https://github.com/tcoopman/react-es6-browserify
Copyright (c) 2015 Richard Willis
FAQs
Seed project for React apps using ES6 & webpack.
The npm package react-seed receives a total of 14 weekly downloads. As such, react-seed popularity was classified as not popular.
We found that react-seed demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.