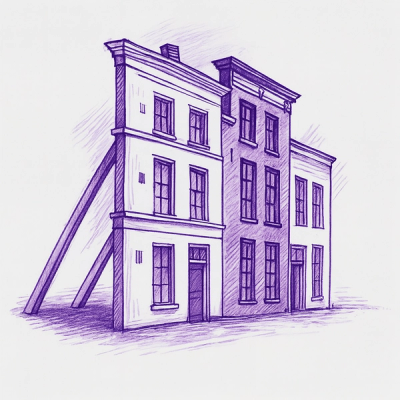
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
react-simple-media
Advanced tools
Easy-to-use media queries for your React project
react-simple-media
dependency to your project# Using npm
npm add react-simple-media
# Using yarn
yarn add react-simple-media
# Using pnpm
pnpm add react-simple-media
MediaQueryProvider
+ import { MediaQueryProvider } from 'react-simple-media'
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(
+ <MediaQueryManager>
<React.StrictMode>
<App />
</React.StrictMode>
+ </MediaQueryManager>
);
useMediaQuery
in any component!// App.tsx
import React, { useMemo } from 'react'
import { useMediaQuery } from 'react-simple-media'
const App: React.FC = () => {
const mediaQuery = useMediaQuery()
// You can use it in your code.
const isDesktopOrHigher = useMemo(() => {
return mediaQuery.isLessThan('desktop') && mediaQuery.isGreaterThan('mobileWide')
}, [mediaQuery.breakpoint])
return (
<div>
<h1>This is my App!</h1>
{/* Or directly in the markup! */}
{mediaQuery.isLessThan('tablet') && <div>I will be visible only on mobile!</div>}
</div>
)
}
react-simple-media
dependency to your project# Using npm
npm add react-simple-media
# Using yarn
yarn add react-simple-media
# Using pnpm
pnpm add react-simple-media
MediaQueryProvider
& initialize the MediaQueryManager
(for SSR)Before
// pages/_app.tsx
function MyApp({ Component, pageProps }: AppProps) {
return (
<Component {...pageProps} />
)
}
After
// pages/_app.tsx
import { MediaQueryManager, MediaQueryProvider } from 'react-simple-media'
type InitialProps = {
contextBreakpoint: string
}
const mediaQueryManager = new MediaQueryManager()
function MyApp({ Component, contextBreakpoint, pageProps }: AppProps & InitialProps) {
return (
<MediaQueryProvider contextBreakpoint={contextBreakpoint}>
<Component {...pageProps} />
</MediaQueryProvider>
)
}
// Detect the breakpoint on the server.
MyApp.getInitialProps = async ({ ctx }: AppContextType) => {
const contextBreakpoint = await mediaQueryManager.detectBreakpoint(
ctx.req?.headers.cookie,
ctx.req?.headers['user-agent'],
)
return {
contextBreakpoint,
} as InitialProps
}
MediaQueryManager
Note: you can initialize an endless amount of
MediaQueryManager
, but each new one will refer to the first one.
const mediaQueryManager = new MediaQueryManager({
// ...
})
breakpoints
An object where the key is the name of the mediaQuery, and the value is the breakpoint size.
cookieName
breakpoint
The key for the document cookie.
defaultBreakpoints
console
, desktop
, embedded
, mobile
, smarttv
, tablet
, wearable
An object where the key is the name of the detected device, and the value is the breakpoint key.
fallbackBreakpoint
desktop
The breakpoint key to be used, if the device was not detected.
{
breakpoints: {
desktop: 1024,
desktopMedium: 1280,
desktopWide: 1600,
mobile: 320,
mobileMedium: 375,
mobileWide: 425,
tablet: 768,
},
cookieName: 'breakpoint',
defaultBreakpoints: {
desktop: 'desktop',
mobile: 'mobile',
tablet: 'tablet',
},
fallbackBreakpoint: 'desktop',
}
MediaQueryManager
detectBreakpoint(cookie?: string, userAgent?: string): Promise<string>
getMediaQueries(): Record<string, string>
usePreset(key: keyof typeof PRESETS): MediaQueryManager<Record<string, number>>
MediaQueryManagerOptions
defaultBreakpoints: Partial<Record<'console' | 'desktop' | 'embedded' | 'mobile' | 'smarttv' | 'tablet' | 'wearable', string>>
breakpoints: Record<string, number>
cookieName: string
fallbackBreakpoint: string
useMediaQuery
readonly breakpoint: string
isGreaterThan(input: string): boolean
isGreaterOrEquals(input: string): any
isLessThan(input: string): boolean
match(input: string): boolean
matches(...input: string[]): boolean
Copyright (c) mvrlin mvrlin@pm.me
FAQs
Define media queries for your React project
The npm package react-simple-media receives a total of 0 weekly downloads. As such, react-simple-media popularity was classified as not popular.
We found that react-simple-media demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.