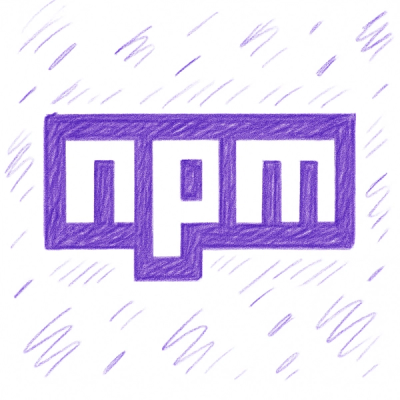
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
react-table
Advanced tools
Hooks for building lightweight, fast and extendable datagrids for React
The react-table package is a lightweight, flexible, and extensible data grid library for React. It allows developers to build complex, interactive, and performant data grids and tables with minimal effort. It provides hooks and utilities to turn any table into a fully functional data grid, including features like sorting, filtering, pagination, and more.
Sorting
This feature allows users to sort data in the table by clicking on the column headers. The code sample demonstrates how to set up a basic table with sortable columns using react-table hooks.
const { useTable, useSortBy } = require('react-table');
function Table({ columns, data }) {
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } = useTable({ columns, data }, useSortBy);
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps(column.getSortByToggleProps())}>
{column.render('Header')}
<span>
{column.isSorted ? (column.isSortedDesc ? ' 🔽' : ' 🔼') : ''}
</span>
</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => {
return <td {...cell.getCellProps()}>{cell.render('Cell')}</td>;
})}
</tr>
);
})}
</tbody>
</table>
);
}
Pagination
This feature enables pagination for the table data, allowing users to navigate through pages. The code sample shows how to implement pagination controls and functionality using react-table hooks.
const { useTable, usePagination } = require('react-table');
function Table({ columns, data }) {
const {
getTableProps,
getTableBodyProps,
headerGroups,
page,
prepareRow,
canPreviousPage,
canNextPage,
pageOptions,
pageCount,
gotoPage,
nextPage,
previousPage,
setPageSize,
state: { pageIndex, pageSize },
} = useTable({ columns, data }, usePagination);
// Table structure similar to the sorting example
return (
<div>
{/* Table structure here */}
<div className="pagination">
<button onClick={() => gotoPage(0)} disabled={!canPreviousPage}>{'<<'}</button>
<button onClick={() => previousPage()} disabled={!canPreviousPage}>{'<'}</button>
<button onClick={() => nextPage()} disabled={!canNextPage}>{'>'}</button>
<button onClick={() => gotoPage(pageCount - 1)} disabled={!canNextPage}>{'>>'}</button>
<span>
Page{' '}
<strong>
{pageIndex + 1} of {pageOptions.length}
</strong>
</span>
<select
value={pageSize}
onChange={e => {
setPageSize(Number(e.target.value));
}}
>
{[10, 20, 30, 40, 50].map(pageSize => (
<option key={pageSize} value={pageSize}>
Show {pageSize}
</option>
))}
</select>
</div>
</div>
);
}
Filtering
This feature allows users to filter table data based on input. The code sample illustrates how to add a simple text input for filtering data in a specific column using react-table hooks.
const { useTable, useFilters } = require('react-table');
function Table({ columns, data }) {
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow, setFilter } = useTable({ columns, data }, useFilters);
// Table and header structure similar to the sorting example
return (
<div>
<input
value={filterInput}
onChange={e => setFilter('columnName', e.target.value)}
placeholder={`Search ${'columnName'}`}
/>
{/* Table structure here */}
</div>
);
}
ag-Grid is a feature-rich data grid library for React. It offers a wide range of functionalities out of the box, such as sorting, filtering, and pagination, similar to react-table. However, ag-Grid is known for its enterprise-level features, including complex data integration, custom cell rendering, and support for large datasets, making it more suitable for complex applications.
material-table is built on Material-UI and offers a modern design and user experience. It provides similar functionalities to react-table, like sorting, filtering, and pagination, but with a focus on Material Design. It's a good choice for projects already using Material-UI and looking for a table solution that integrates well with that design system.
react-data-grid is another alternative to react-table, focusing on performance and flexibility. It supports features like sorting, filtering, and pagination, but it's particularly noted for its performance with large datasets and its extensible cell formatting and editing capabilities. It's a solid choice for applications that require high performance and customizable data grids.
FAQs
Hooks for building lightweight, fast and extendable datagrids for React
The npm package react-table receives a total of 1,337,547 weekly downloads. As such, react-table popularity was classified as popular.
We found that react-table demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.