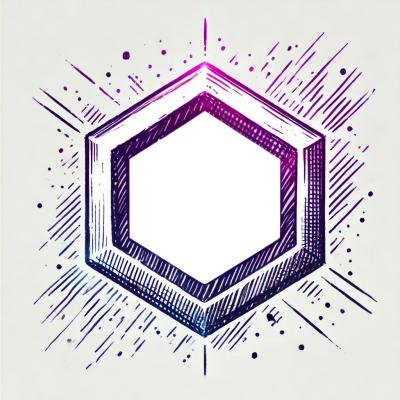
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
react-text-to-speech
Advanced tools
An easy-to-use React.js component that leverages the Web Speech API to convert text to speech.
An easy-to-use React.js component that leverages the Web Speech API to convert text to speech.
Install react-text-to-speech
using your preferred package manager:
# Using npm:
npm install react-text-to-speech --save
# Using Yarn:
yarn add react-text-to-speech
# Using pnpm:
pnpm add react-text-to-speech
# Using Bun:
bun add react-text-to-speech
react-text-to-speech provides two primary methods to integrate text-to-speech functionality into your React.js applications: the useSpeech
hook and the <Speech>
component.
useSpeech
Hookimport React from "react";
import { useSpeech } from "react-text-to-speech";
export default function App() {
const {
Text, // Component that renders the processed text
speechStatus, // Current speech status
isInQueue, // Indicates if the speech is active or queued
start, // Starts or queues the speech
pause, // Pauses the speech
stop, // Stops or removes the speech from the queue
} = useSpeech({ text: "This library is awesome!" });
return (
<div style={{ display: "flex", flexDirection: "column", rowGap: "1rem" }}>
<Text />
<div style={{ display: "flex", columnGap: "0.5rem" }}>
{speechStatus !== "started" ? <button onClick={start}>Start</button> : <button onClick={pause}>Pause</button>}
<button onClick={stop}>Stop</button>
</div>
</div>
);
}
For more details on using the useSpeech
hook, refer to the documentation.
<Speech>
Componentimport React from "react";
import Speech from "react-text-to-speech";
export default function App() {
return <Speech text="This library is awesome!" />;
}
For more details on using the <Speech>
component, refer to the documentation.
Check out the live demo to see it in action.
Explore the documentation to get started quickly.
Show your ❤️ and support by giving a ⭐ on GitHub. You can also support the project by upvoting and sharing it on Product Hunt. Any suggestions are welcome! Take a look at the contributing guide.
This project is licensed under the MIT License.
FAQs
An easy-to-use React.js component that leverages the Web Speech API to convert text to speech.
The npm package react-text-to-speech receives a total of 0 weekly downloads. As such, react-text-to-speech popularity was classified as not popular.
We found that react-text-to-speech demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.