react-timepicker
Advanced tools
Comparing version 1.0.4 to 1.1.0
Package.describe({ | ||
name: 'universe:react-timepicker', | ||
version: '1.0.4', | ||
version: '1.1.0', | ||
documentation: 'README.md', | ||
@@ -10,6 +10,6 @@ summary: 'React timepicker in Android KitKat style', | ||
Package.onUse(function (api) { | ||
api.versionsFrom('1.2.0.2'); | ||
api.versionsFrom('1.2.1'); | ||
api.use('react-runtime@0.13.3_7'); | ||
api.use('universe:modules@0.6.1', { weak:true }); | ||
api.use('react-runtime@0.14.1_1'); | ||
api.use('universe:modules@0.6.1', { weak: true }); | ||
@@ -16,0 +16,0 @@ api.addFiles('timepicker.js'); |
@@ -7,3 +7,3 @@ { | ||
"version": "1.0.4", | ||
"version": "1.1.0", | ||
"description": "React timepicker in Android KitKat style", | ||
@@ -10,0 +10,0 @@ |
# react-timepicker | ||
`Timepicker` is a [React]() timepicker component that looks like Android KitKat one. | ||
`Timepicker` is a [React](https://facebook.github.io/react/) timepicker component that looks like Android KitKat one. | ||
### [Demo][] | ||
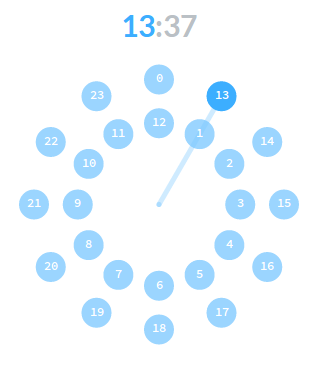 | ||
### Example | ||
[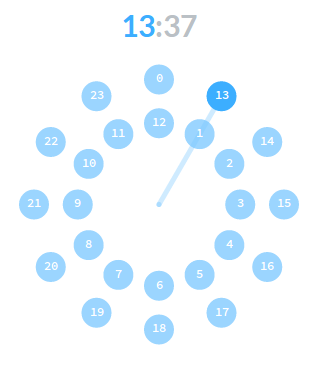](https://jsfiddle.net/radekm/o7syg3q9/embedded/result/) | ||
### Dependencies | ||
### Install | ||
From [npm](https://www.npmjs.com/package/react-timepicker) | ||
* [React][] | ||
### How To | ||
Using [npm][] | ||
```sh | ||
npm install react-timepicker | ||
npm install react-timepicker --save | ||
``` | ||
Using [Meteor][] | ||
From [Atmosphere](https://atmospherejs.com/universe/react-timepicker) | ||
@@ -24,31 +20,66 @@ ```sh | ||
### Usage | ||
```js | ||
### Quick Start | ||
```javascript | ||
'use strict'; | ||
import React from 'react'; | ||
import ReactDOM from 'react-dom'; | ||
import Timepicker from 'react-timepicker'; | ||
// Remember to include timepicker.css | ||
<Timepicker {...timepickerOptions} /> | ||
class TimepickerExample extends React.Component { | ||
onChange (hours, minutes) { | ||
// ... | ||
}, | ||
render () { | ||
return ( | ||
<Timepicker onChange={this.onChange} /> | ||
); | ||
} | ||
} | ||
ReactDOM.render(<TimepickerExample />, document.getElementById('timepicker-example')); | ||
``` | ||
### Options | ||
```js | ||
// Everything is optional. | ||
var timepickerOptions = { | ||
mode: Timepicker.MODE_HOURS, // or Timepicker.MODE_MINUTES | ||
### Prop Values | ||
#### mode | ||
`React.PropTypes.any` | ||
size: 300, | ||
radius: 125, | ||
Initial mode - `Timepicker.MODE_HOURS` or `Timepicker.MODE_MINUTES` **(default: `Timepicker.MODE_HOURS`)**. | ||
hours: 0, | ||
minutes: 0, | ||
#### size | ||
`React.PropTypes.number` | ||
militaryTime: true // 24 hours if true, 12 otherwise | ||
Clock size in pixels **(default: 300)**. | ||
onChange: function (hours, minutes) {}, | ||
onChangeMode: function (mode) {} | ||
}; | ||
``` | ||
#### radius | ||
`React.PropTypes.number` | ||
[npm]: https://npmjs.org/ | ||
[React]: https://facebook.github.io/react/ | ||
[Meteor]: https://www.meteor.com/ | ||
Clock radius in pixels **(default: 125)**. | ||
[Demo]: https://jsfiddle.net/radekm/o7syg3q9/embedded/result/ | ||
#### hours | ||
`React.PropTypes.number` | ||
Initial hours **(default: 0)**. | ||
#### minutes | ||
`React.PropTypes.number` | ||
Initial minutes **(default: 0)**. | ||
#### militaryTime | ||
`React.PropTypes.bool` | ||
Military (24-hour) time switch **(default: `true`)**. | ||
#### onChange | ||
`React.PropTypes.func` | ||
Callback function when a hour or a minute is changed. Passes 2 parameters: new hours and minutes. | ||
#### onChangeMode | ||
`React.PropTypes.func` | ||
Callback function when mode is changed. Passes 1 parameter: new mode. |
@@ -262,5 +262,10 @@ 'use strict'; | ||
var hand1Length = Math.hypot(hand1.getAttribute('x1') - hand1.getAttribute('x2'), hand1.getAttribute('y1') - hand1.getAttribute('y2')); | ||
var hand2Length = Math.hypot(hand2.getAttribute('x1') - hand2.getAttribute('x2'), hand2.getAttribute('y1') - hand2.getAttribute('y2')); | ||
var dx1 = hand1.getAttribute('x1') - hand1.getAttribute('x2'); | ||
var dy1 = hand1.getAttribute('y1') - hand1.getAttribute('y2'); | ||
var dx2 = hand2.getAttribute('x1') - hand2.getAttribute('x2'); | ||
var dy2 = hand2.getAttribute('y1') - hand2.getAttribute('y2'); | ||
var hand1Length = Math.ceil(Math.sqrt(dx1 * dx1 + dy1 * dy1)); | ||
var hand2Length = Math.ceil(Math.sqrt(dx2 * dx2 + dy2 * dy2)); | ||
hand1.style.strokeDasharray = hand1Length; | ||
@@ -469,4 +474,4 @@ hand2.style.strokeDasharray = hand2Length; | ||
positions.push([ | ||
size / 2 + radius * (militaryTime ? index > 12 ? 1 : 0.65 : 1) * Math.cos((index % 12 / 6 - 0.5) * Math.PI), | ||
size / 2 + radius * (militaryTime ? index > 12 ? 1 : 0.65 : 1) * Math.sin((index % 12 / 6 - 0.5) * Math.PI) | ||
Math.round(size / 2 + radius * (militaryTime ? index > 12 ? 1 : 0.65 : 1) * Math.cos((index % 12 / 6 - 0.5) * Math.PI)), | ||
Math.round(size / 2 + radius * (militaryTime ? index > 12 ? 1 : 0.65 : 1) * Math.sin((index % 12 / 6 - 0.5) * Math.PI)) | ||
]); | ||
@@ -489,4 +494,4 @@ } | ||
positions.push([ | ||
size / 2 + radius * Math.cos((index / 30 - 0.5) * Math.PI), | ||
size / 2 + radius * Math.sin((index / 30 - 0.5) * Math.PI) | ||
Math.round(size / 2 + radius * Math.cos((index / 30 - 0.5) * Math.PI)), | ||
Math.round(size / 2 + radius * Math.sin((index / 30 - 0.5) * Math.PI)) | ||
]); | ||
@@ -493,0 +498,0 @@ } |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
37591
494
85