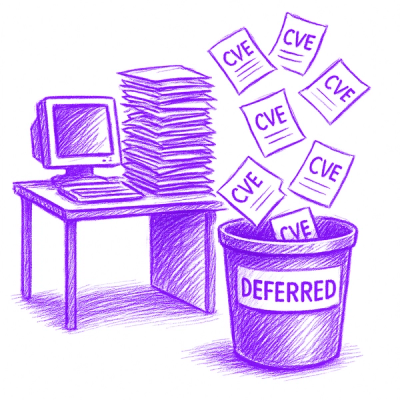
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
react-timer-mixin
Advanced tools
TimerMixin provides timer functions for executing code in the future that are safely cleaned up when the component unmounts
Using bare setTimeout, setInterval, setImmediate and requestAnimationFrame calls is very dangerous because if you forget to cancel the request before the component is unmounted, you risk the callback throwing an exception.
If you include TimerMixin, then you can replace your calls to
setTimeout(fn, 500)
with this.setTimeout(fn, 500)
(just prepend this.
) and
everything will be properly cleaned up for you.
Install the module directly from npm:
npm install react-timer-mixin
var React = require('react');
var TimerMixin = require('react-timer-mixin');
var Component = React.createClass({
mixins: [TimerMixin],
componentDidMount() {
this.setTimeout(
() => { console.log('I do not leak!'); },
500
);
}
});
FAQs
TimerMixin provides timer functions for executing code in the future that are safely cleaned up when the component unmounts
The npm package react-timer-mixin receives a total of 68,924 weekly downloads. As such, react-timer-mixin popularity was classified as popular.
We found that react-timer-mixin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.