react-ultimate-pagination
Advanced tools
Comparing version
@@ -76,3 +76,3 @@ (function webpackUniversalModuleDefinition(root, factory) { | ||
/******/ // Load entry module and return exports | ||
/******/ return __webpack_require__(__webpack_require__.s = 5); | ||
/******/ return __webpack_require__(__webpack_require__.s = 3); | ||
/******/ }) | ||
@@ -110,4 +110,4 @@ /************************************************************************/ | ||
var ultimate_pagination_utils_1 = __webpack_require__(4); | ||
var ultimate_pagination_item_factories_1 = __webpack_require__(3); | ||
var ultimate_pagination_utils_1 = __webpack_require__(5); | ||
var ultimate_pagination_item_factories_1 = __webpack_require__(4); | ||
function getPaginationModel(options) { | ||
@@ -227,2 +227,89 @@ if (options == null) { | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.ITEM_TYPES = exports.createUltimatePagination = undefined; | ||
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; | ||
var _react = __webpack_require__(2); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _ultimatePagination = __webpack_require__(1); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
var renderItemComponentFunctionFactory = function renderItemComponentFunctionFactory(itemTypeToComponent, currentPage, onChange) { | ||
var onItemClickFunctionFactory = function onItemClickFunctionFactory(value) { | ||
return function () { | ||
if (onChange && currentPage !== value) { | ||
onChange(value); | ||
} | ||
}; | ||
}; | ||
return function (item) { | ||
var ItemComponent = itemTypeToComponent[item.type]; | ||
var onItemClick = onItemClickFunctionFactory(item.value); | ||
return _react2.default.createElement(ItemComponent, _extends({ onClick: onItemClick }, item)); | ||
}; | ||
}; | ||
var createUltimatePagination = exports.createUltimatePagination = function createUltimatePagination(_ref) { | ||
var itemTypeToComponent = _ref.itemTypeToComponent, | ||
_ref$WrapperComponent = _ref.WrapperComponent, | ||
WrapperComponent = _ref$WrapperComponent === undefined ? 'div' : _ref$WrapperComponent; | ||
var UltimatePaginationComponent = function UltimatePaginationComponent(props) { | ||
var currentPage = props.currentPage, | ||
totalPages = props.totalPages, | ||
boundaryPagesRange = props.boundaryPagesRange, | ||
siblingPagesRange = props.siblingPagesRange, | ||
hideEllipsis = props.hideEllipsis, | ||
hidePreviousAndNextPageLinks = props.hidePreviousAndNextPageLinks, | ||
hideFirstAndLastPageLinks = props.hideFirstAndLastPageLinks, | ||
onChange = props.onChange; | ||
var paginationModel = (0, _ultimatePagination.getPaginationModel)({ | ||
currentPage: currentPage, | ||
totalPages: totalPages, | ||
boundaryPagesRange: boundaryPagesRange, | ||
siblingPagesRange: siblingPagesRange, | ||
hideEllipsis: hideEllipsis, | ||
hidePreviousAndNextPageLinks: hidePreviousAndNextPageLinks, | ||
hideFirstAndLastPageLinks: hideFirstAndLastPageLinks | ||
}); | ||
var renderItemComponent = renderItemComponentFunctionFactory(itemTypeToComponent, currentPage, onChange); | ||
return _react2.default.createElement( | ||
WrapperComponent, | ||
null, | ||
paginationModel.map(renderItemComponent) | ||
); | ||
}; | ||
UltimatePaginationComponent.propTypes = { | ||
currentPage: _react2.default.PropTypes.number.isRequired, | ||
totalPages: _react2.default.PropTypes.number.isRequired, | ||
boundaryPagesRange: _react2.default.PropTypes.number, | ||
siblingPagesRange: _react2.default.PropTypes.number, | ||
hideEllipsis: _react2.default.PropTypes.bool, | ||
hidePreviousAndNextPageLinks: _react2.default.PropTypes.bool, | ||
hideFirstAndLastPageLinks: _react2.default.PropTypes.bool, | ||
onChange: _react2.default.PropTypes.func | ||
}; | ||
return UltimatePaginationComponent; | ||
}; | ||
exports.ITEM_TYPES = _ultimatePagination.ITEM_TYPES; | ||
/***/ }), | ||
/* 4 */ | ||
/***/ (function(module, exports, __webpack_require__) { | ||
"use strict"; | ||
var ultimate_pagination_constants_1 = __webpack_require__(0); | ||
@@ -295,3 +382,3 @@ exports.createFirstEllipsis = function (pageNumber) { | ||
/***/ }), | ||
/* 4 */ | ||
/* 5 */ | ||
/***/ (function(module, exports, __webpack_require__) { | ||
@@ -311,89 +398,2 @@ | ||
/***/ }), | ||
/* 5 */ | ||
/***/ (function(module, exports, __webpack_require__) { | ||
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.ITEM_TYPES = exports.createUltimatePagination = undefined; | ||
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; | ||
var _react = __webpack_require__(2); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _ultimatePagination = __webpack_require__(1); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
var renderItemComponentFunctionFactory = function renderItemComponentFunctionFactory(itemTypeToComponent, currentPage, onChange) { | ||
var onItemClickFunctionFactory = function onItemClickFunctionFactory(value) { | ||
return function () { | ||
if (onChange && currentPage !== value) { | ||
onChange(value); | ||
} | ||
}; | ||
}; | ||
return function (item) { | ||
var ItemComponent = itemTypeToComponent[item.type]; | ||
var onItemClick = onItemClickFunctionFactory(item.value); | ||
return _react2.default.createElement(ItemComponent, _extends({ onClick: onItemClick }, item)); | ||
}; | ||
}; | ||
var createUltimatePagination = exports.createUltimatePagination = function createUltimatePagination(_ref) { | ||
var itemTypeToComponent = _ref.itemTypeToComponent, | ||
_ref$WrapperComponent = _ref.WrapperComponent, | ||
WrapperComponent = _ref$WrapperComponent === undefined ? 'div' : _ref$WrapperComponent; | ||
var UltimatePaginationComponent = function UltimatePaginationComponent(props) { | ||
var currentPage = props.currentPage, | ||
totalPages = props.totalPages, | ||
boundaryPagesRange = props.boundaryPagesRange, | ||
siblingPagesRange = props.siblingPagesRange, | ||
hideEllipsis = props.hideEllipsis, | ||
hidePreviousAndNextPageLinks = props.hidePreviousAndNextPageLinks, | ||
hideFirstAndLastPageLinks = props.hideFirstAndLastPageLinks, | ||
onChange = props.onChange; | ||
var paginationModel = (0, _ultimatePagination.getPaginationModel)({ | ||
currentPage: currentPage, | ||
totalPages: totalPages, | ||
boundaryPagesRange: boundaryPagesRange, | ||
siblingPagesRange: siblingPagesRange, | ||
hideEllipsis: hideEllipsis, | ||
hidePreviousAndNextPageLinks: hidePreviousAndNextPageLinks, | ||
hideFirstAndLastPageLinks: hideFirstAndLastPageLinks | ||
}); | ||
var renderItemComponent = renderItemComponentFunctionFactory(itemTypeToComponent, currentPage, onChange); | ||
return _react2.default.createElement( | ||
WrapperComponent, | ||
null, | ||
paginationModel.map(renderItemComponent) | ||
); | ||
}; | ||
UltimatePaginationComponent.propTypes = { | ||
currentPage: _react2.default.PropTypes.number.isRequired, | ||
totalPages: _react2.default.PropTypes.number.isRequired, | ||
boundaryPagesRange: _react2.default.PropTypes.number, | ||
siblingPagesRange: _react2.default.PropTypes.number, | ||
hideEllipsis: _react2.default.PropTypes.bool, | ||
hidePreviousAndNextPageLinks: _react2.default.PropTypes.bool, | ||
hideFirstAndLastPageLinks: _react2.default.PropTypes.bool, | ||
onChange: _react2.default.PropTypes.func | ||
}; | ||
return UltimatePaginationComponent; | ||
}; | ||
exports.ITEM_TYPES = _ultimatePagination.ITEM_TYPES; | ||
/***/ }) | ||
@@ -400,0 +400,0 @@ /******/ ]); |
@@ -1,2 +0,2 @@ | ||
!function(e,t){"object"==typeof exports&&"object"==typeof module?module.exports=t(require("react")):"function"==typeof define&&define.amd?define(["react"],t):"object"==typeof exports?exports.reactUltimatePagination=t(require("react")):e.reactUltimatePagination=t(e.React)}(this,function(e){return function(e){function t(a){if(n[a])return n[a].exports;var r=n[a]={i:a,l:!1,exports:{}};return e[a].call(r.exports,r,r.exports,t),r.l=!0,r.exports}var n={};return t.m=e,t.c=n,t.i=function(e){return e},t.d=function(e,n,a){t.o(e,n)||Object.defineProperty(e,n,{configurable:!1,enumerable:!0,get:a})},t.n=function(e){var n=e&&e.__esModule?function(){return e.default}:function(){return e};return t.d(n,"a",n),n},t.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},t.p="",t(t.s=5)}([function(e,t,n){"use strict";t.ITEM_TYPES={PAGE:"PAGE",ELLIPSIS:"ELLIPSIS",FIRST_PAGE_LINK:"FIRST_PAGE_LINK",PREVIOUS_PAGE_LINK:"PREVIOUS_PAGE_LINK",NEXT_PAGE_LINK:"NEXT_PAGE_LINK",LAST_PAGE_LINK:"LAST_PAGE_LINK"},t.ITEM_KEYS={FIRST_ELLIPSIS:-1,SECOND_ELLIPSIS:-2,FIRST_PAGE_LINK:-3,PREVIOUS_PAGE_LINK:-4,NEXT_PAGE_LINK:-5,LAST_PAGE_LINK:-6}},function(e,t,n){"use strict";function a(e){if(null==e)throw new Error("getPaginationModel(): options object should be a passed");var t=Number(e.totalPages);if(isNaN(t))throw new Error("getPaginationModel(): totalPages should be a number");if(t<0)throw new Error("getPaginationModel(): totalPages shouldn't be a negative number");var n=Number(e.currentPage);if(isNaN(n))throw new Error("getPaginationModel(): currentPage should be a number");if(n<0)throw new Error("getPaginationModel(): currentPage shouldn't be a negative number");if(n>t)throw new Error("getPaginationModel(): currentPage shouldn't be greater than totalPages");var a=null==e.boundaryPagesRange?1:Number(e.boundaryPagesRange);if(isNaN(a))throw new Error("getPaginationModel(): boundaryPagesRange should be a number");if(a<0)throw new Error("getPaginationModel(): boundaryPagesRange shouldn't be a negative number");var o=null==e.siblingPagesRange?1:Number(e.siblingPagesRange);if(isNaN(o))throw new Error("getPaginationModel(): siblingPagesRange should be a number");if(o<0)throw new Error("getPaginationModel(): siblingPagesRange shouldn't be a negative number");var u=Boolean(e.hidePreviousAndNextPageLinks),s=Boolean(e.hideFirstAndLastPageLinks),P=Boolean(e.hideEllipsis),g=P?0:1,l=[],c=i.createPageFunctionFactory(e);if(s||l.push(i.createFirstPageLink(e)),u||l.push(i.createPreviousPageLink(e)),1+2*g+2*o+2*a>=t){var E=r.createRange(1,t).map(c);l.push.apply(l,E)}else{var p=1,d=a,f=r.createRange(p,d).map(c),_=t+1-a,I=t,L=r.createRange(_,I).map(c),T=Math.min(Math.max(n-o,d+g+1),_-g-2*o-1),S=T+2*o,v=r.createRange(T,S).map(c);if(l.push.apply(l,f),!P){var b=T-1,h=b===d+1,y=h?c:i.createFirstEllipsis,N=y(b);l.push(N)}if(l.push.apply(l,v),!P){var A=S+1,M=A===_-1,m=M?c:i.createSecondEllipsis,R=m(A);l.push(R)}l.push.apply(l,L)}return u||l.push(i.createNextPageLink(e)),s||l.push(i.createLastPageLink(e)),l}var r=n(4),i=n(3);t.getPaginationModel=a;var o=n(0);t.ITEM_TYPES=o.ITEM_TYPES,t.ITEM_KEYS=o.ITEM_KEYS},function(t,n){t.exports=e},function(e,t,n){"use strict";var a=n(0);t.createFirstEllipsis=function(e){return{type:a.ITEM_TYPES.ELLIPSIS,key:a.ITEM_KEYS.FIRST_ELLIPSIS,value:e,isActive:!1}},t.createSecondEllipsis=function(e){return{type:a.ITEM_TYPES.ELLIPSIS,key:a.ITEM_KEYS.SECOND_ELLIPSIS,value:e,isActive:!1}},t.createFirstPageLink=function(e){var t=e.currentPage;return{type:a.ITEM_TYPES.FIRST_PAGE_LINK,key:a.ITEM_KEYS.FIRST_PAGE_LINK,value:1,isActive:1===t}},t.createPreviousPageLink=function(e){var t=e.currentPage;return{type:a.ITEM_TYPES.PREVIOUS_PAGE_LINK,key:a.ITEM_KEYS.PREVIOUS_PAGE_LINK,value:Math.max(1,t-1),isActive:1===t}},t.createNextPageLink=function(e){var t=e.currentPage,n=e.totalPages;return{type:a.ITEM_TYPES.NEXT_PAGE_LINK,key:a.ITEM_KEYS.NEXT_PAGE_LINK,value:Math.min(n,t+1),isActive:t===n}},t.createLastPageLink=function(e){var t=e.currentPage,n=e.totalPages;return{type:a.ITEM_TYPES.LAST_PAGE_LINK,key:a.ITEM_KEYS.LAST_PAGE_LINK,value:n,isActive:t===n}},t.createPageFunctionFactory=function(e){var t=e.currentPage;return function(e){return{type:a.ITEM_TYPES.PAGE,key:e,value:e,isActive:e===t}}}},function(e,t,n){"use strict";function a(e,t){for(var n=[],a=e;a<=t;a++)n.push(a);return n}t.createRange=a},function(e,t,n){"use strict";function a(e){return e&&e.__esModule?e:{default:e}}Object.defineProperty(t,"__esModule",{value:!0}),t.ITEM_TYPES=t.createUltimatePagination=void 0;var r=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var a in n)Object.prototype.hasOwnProperty.call(n,a)&&(e[a]=n[a])}return e},i=n(2),o=a(i),u=n(1),s=function(e,t,n){var a=function(e){return function(){n&&t!==e&&n(e)}};return function(t){var n=e[t.type],i=a(t.value);return o.default.createElement(n,r({onClick:i},t))}};t.createUltimatePagination=function(e){var t=e.itemTypeToComponent,n=e.WrapperComponent,a=void 0===n?"div":n,r=function(e){var n=e.currentPage,r=e.totalPages,i=e.boundaryPagesRange,P=e.siblingPagesRange,g=e.hideEllipsis,l=e.hidePreviousAndNextPageLinks,c=e.hideFirstAndLastPageLinks,E=e.onChange,p=(0,u.getPaginationModel)({currentPage:n,totalPages:r,boundaryPagesRange:i,siblingPagesRange:P,hideEllipsis:g,hidePreviousAndNextPageLinks:l,hideFirstAndLastPageLinks:c}),d=s(t,n,E);return o.default.createElement(a,null,p.map(d))};return r.propTypes={currentPage:o.default.PropTypes.number.isRequired,totalPages:o.default.PropTypes.number.isRequired,boundaryPagesRange:o.default.PropTypes.number,siblingPagesRange:o.default.PropTypes.number,hideEllipsis:o.default.PropTypes.bool,hidePreviousAndNextPageLinks:o.default.PropTypes.bool,hideFirstAndLastPageLinks:o.default.PropTypes.bool,onChange:o.default.PropTypes.func},r};t.ITEM_TYPES=u.ITEM_TYPES}])}); | ||
!function(e,t){"object"==typeof exports&&"object"==typeof module?module.exports=t(require("react")):"function"==typeof define&&define.amd?define(["react"],t):"object"==typeof exports?exports.reactUltimatePagination=t(require("react")):e.reactUltimatePagination=t(e.React)}(this,function(e){return function(e){function t(a){if(n[a])return n[a].exports;var r=n[a]={i:a,l:!1,exports:{}};return e[a].call(r.exports,r,r.exports,t),r.l=!0,r.exports}var n={};return t.m=e,t.c=n,t.i=function(e){return e},t.d=function(e,n,a){t.o(e,n)||Object.defineProperty(e,n,{configurable:!1,enumerable:!0,get:a})},t.n=function(e){var n=e&&e.__esModule?function(){return e.default}:function(){return e};return t.d(n,"a",n),n},t.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},t.p="",t(t.s=3)}([function(e,t,n){"use strict";t.ITEM_TYPES={PAGE:"PAGE",ELLIPSIS:"ELLIPSIS",FIRST_PAGE_LINK:"FIRST_PAGE_LINK",PREVIOUS_PAGE_LINK:"PREVIOUS_PAGE_LINK",NEXT_PAGE_LINK:"NEXT_PAGE_LINK",LAST_PAGE_LINK:"LAST_PAGE_LINK"},t.ITEM_KEYS={FIRST_ELLIPSIS:-1,SECOND_ELLIPSIS:-2,FIRST_PAGE_LINK:-3,PREVIOUS_PAGE_LINK:-4,NEXT_PAGE_LINK:-5,LAST_PAGE_LINK:-6}},function(e,t,n){"use strict";function a(e){if(null==e)throw new Error("getPaginationModel(): options object should be a passed");var t=Number(e.totalPages);if(isNaN(t))throw new Error("getPaginationModel(): totalPages should be a number");if(t<0)throw new Error("getPaginationModel(): totalPages shouldn't be a negative number");var n=Number(e.currentPage);if(isNaN(n))throw new Error("getPaginationModel(): currentPage should be a number");if(n<0)throw new Error("getPaginationModel(): currentPage shouldn't be a negative number");if(n>t)throw new Error("getPaginationModel(): currentPage shouldn't be greater than totalPages");var a=null==e.boundaryPagesRange?1:Number(e.boundaryPagesRange);if(isNaN(a))throw new Error("getPaginationModel(): boundaryPagesRange should be a number");if(a<0)throw new Error("getPaginationModel(): boundaryPagesRange shouldn't be a negative number");var o=null==e.siblingPagesRange?1:Number(e.siblingPagesRange);if(isNaN(o))throw new Error("getPaginationModel(): siblingPagesRange should be a number");if(o<0)throw new Error("getPaginationModel(): siblingPagesRange shouldn't be a negative number");var u=Boolean(e.hidePreviousAndNextPageLinks),s=Boolean(e.hideFirstAndLastPageLinks),P=Boolean(e.hideEllipsis),g=P?0:1,l=[],c=i.createPageFunctionFactory(e);if(s||l.push(i.createFirstPageLink(e)),u||l.push(i.createPreviousPageLink(e)),1+2*g+2*o+2*a>=t){var E=r.createRange(1,t).map(c);l.push.apply(l,E)}else{var p=a,d=r.createRange(1,p).map(c),f=t+1-a,_=t,I=r.createRange(f,_).map(c),L=Math.min(Math.max(n-o,p+g+1),f-g-2*o-1),T=L+2*o,S=r.createRange(L,T).map(c);if(l.push.apply(l,d),!P){var v=L-1,b=v===p+1,h=b?c:i.createFirstEllipsis,y=h(v);l.push(y)}if(l.push.apply(l,S),!P){var N=T+1,A=N===f-1,M=A?c:i.createSecondEllipsis,m=M(N);l.push(m)}l.push.apply(l,I)}return u||l.push(i.createNextPageLink(e)),s||l.push(i.createLastPageLink(e)),l}var r=n(5),i=n(4);t.getPaginationModel=a;var o=n(0);t.ITEM_TYPES=o.ITEM_TYPES,t.ITEM_KEYS=o.ITEM_KEYS},function(t,n){t.exports=e},function(e,t,n){"use strict";Object.defineProperty(t,"__esModule",{value:!0}),t.ITEM_TYPES=t.createUltimatePagination=void 0;var a=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var a in n)Object.prototype.hasOwnProperty.call(n,a)&&(e[a]=n[a])}return e},r=n(2),i=function(e){return e&&e.__esModule?e:{default:e}}(r),o=n(1),u=function(e,t,n){var r=function(e){return function(){n&&t!==e&&n(e)}};return function(t){var n=e[t.type],o=r(t.value);return i.default.createElement(n,a({onClick:o},t))}};t.createUltimatePagination=function(e){var t=e.itemTypeToComponent,n=e.WrapperComponent,a=void 0===n?"div":n,r=function(e){var n=e.currentPage,r=e.totalPages,s=e.boundaryPagesRange,P=e.siblingPagesRange,g=e.hideEllipsis,l=e.hidePreviousAndNextPageLinks,c=e.hideFirstAndLastPageLinks,E=e.onChange,p=(0,o.getPaginationModel)({currentPage:n,totalPages:r,boundaryPagesRange:s,siblingPagesRange:P,hideEllipsis:g,hidePreviousAndNextPageLinks:l,hideFirstAndLastPageLinks:c}),d=u(t,n,E);return i.default.createElement(a,null,p.map(d))};return r.propTypes={currentPage:i.default.PropTypes.number.isRequired,totalPages:i.default.PropTypes.number.isRequired,boundaryPagesRange:i.default.PropTypes.number,siblingPagesRange:i.default.PropTypes.number,hideEllipsis:i.default.PropTypes.bool,hidePreviousAndNextPageLinks:i.default.PropTypes.bool,hideFirstAndLastPageLinks:i.default.PropTypes.bool,onChange:i.default.PropTypes.func},r};t.ITEM_TYPES=o.ITEM_TYPES},function(e,t,n){"use strict";var a=n(0);t.createFirstEllipsis=function(e){return{type:a.ITEM_TYPES.ELLIPSIS,key:a.ITEM_KEYS.FIRST_ELLIPSIS,value:e,isActive:!1}},t.createSecondEllipsis=function(e){return{type:a.ITEM_TYPES.ELLIPSIS,key:a.ITEM_KEYS.SECOND_ELLIPSIS,value:e,isActive:!1}},t.createFirstPageLink=function(e){var t=e.currentPage;return{type:a.ITEM_TYPES.FIRST_PAGE_LINK,key:a.ITEM_KEYS.FIRST_PAGE_LINK,value:1,isActive:1===t}},t.createPreviousPageLink=function(e){var t=e.currentPage;return{type:a.ITEM_TYPES.PREVIOUS_PAGE_LINK,key:a.ITEM_KEYS.PREVIOUS_PAGE_LINK,value:Math.max(1,t-1),isActive:1===t}},t.createNextPageLink=function(e){var t=e.currentPage,n=e.totalPages;return{type:a.ITEM_TYPES.NEXT_PAGE_LINK,key:a.ITEM_KEYS.NEXT_PAGE_LINK,value:Math.min(n,t+1),isActive:t===n}},t.createLastPageLink=function(e){var t=e.currentPage,n=e.totalPages;return{type:a.ITEM_TYPES.LAST_PAGE_LINK,key:a.ITEM_KEYS.LAST_PAGE_LINK,value:n,isActive:t===n}},t.createPageFunctionFactory=function(e){var t=e.currentPage;return function(e){return{type:a.ITEM_TYPES.PAGE,key:e,value:e,isActive:e===t}}}},function(e,t,n){"use strict";function a(e,t){for(var n=[],a=e;a<=t;a++)n.push(a);return n}t.createRange=a}])}); | ||
//# sourceMappingURL=react-ultimate-pagination.min.js.map |
{ | ||
"name": "react-ultimate-pagination", | ||
"version": "1.0.0", | ||
"version": "1.0.1", | ||
"description": "React.js pagination component based on ultimate-pagination", | ||
@@ -37,6 +37,8 @@ "main": "lib/react-ultimate-pagination.js", | ||
"dependencies": { | ||
"react": "^15.0.1", | ||
"react-dom": "^15.0.1", | ||
"ultimate-pagination": "1.0.0" | ||
}, | ||
"peerDependencies": { | ||
"react": "^0.14.0 || ^15.0.0", | ||
"react-dom": "^0.14.0 || ^15.0.0" | ||
}, | ||
"devDependencies": { | ||
@@ -43,0 +45,0 @@ "babel-cli": "^6.7.7", |
@@ -110,3 +110,3 @@ [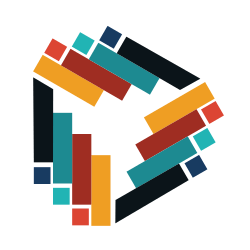](https://github.com/ultimate-pagination/react-ultimate-pagination) | ||
- **hidePreviousAndNextPageLinks**: *bool, optional, default: false* - boolean flag to hide previous and next page links | ||
- **hideFirstAndLastPageLink**: *bool, optional, default: false* - boolean flag to hide first and last page links | ||
- **hideFirstAndLastPageLinks**: *bool, optional, default: false* - boolean flag to hide first and last page links | ||
- **onChange**: *function* - callback that will be called with new page when it should be changed by user interaction (*optional*) | ||
@@ -113,0 +113,0 @@ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
0
-100%91471
-0.02%- Removed
- Removed