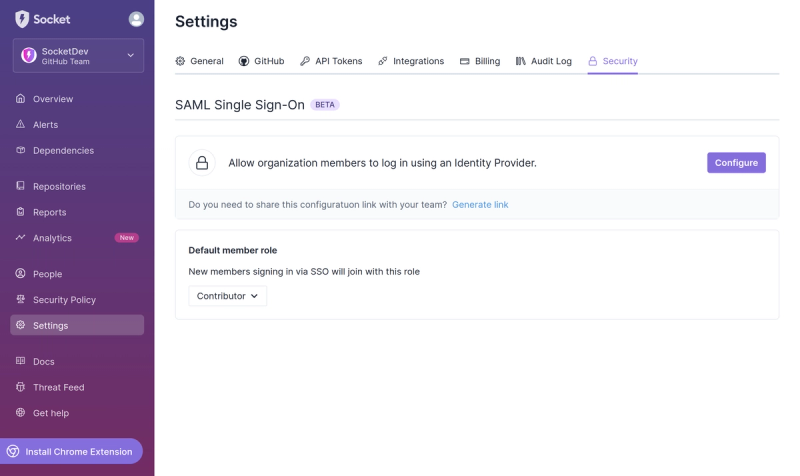
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
react-use-pagination
Advanced tools
Changelog
Readme
import { usePagination } from "react-use-pagination";
function App() {
const [data] = React.useState([]); // <- your data
const {
currentPage,
totalPages,
setNextPage,
setPreviousPage,
nextEnabled,
previousEnabled,
startIndex,
endIndex,
} = usePagination({ totalItems: data.length });
return (
<div>
<MyDataTable data={data.slice(startIndex, endIndex)} />
<button onClick={setPreviousPage} disabled={!previousEnabled}>
Previous Page
</button>
<span>
Current Page: {currentPage} of {totalPages}
</span>
<button onClick={setNextPage} disabled={!nextEnabled}>
Next Page
</button>
</div>
);
}
const paginationState = usePagination(options);
options
type Options = {
totalItems: number;
initialPage?: number; // (default: 0)
initialPageSize?: number; // (default: 0)
};
paginationState
type PaginationState = {
// The current page
currentPage: number;
// The first index of the page window
startIndex: number;
// The last index of the page window
endIndex: number;
// Whether the next button should be enabled
nextEnabled: number;
// Whether the previous button should be enabled
previousEnabled: number;
// The total page size
pageSize: number;
// Jump directly to a page
setPage: (page: number) => void;
// Jump to the next page
setNextPage: () => void;
// Jump to the previous page
setPreviousPage: () => void;
// Set the page size
setPageSize: (pageSize: number, nextPage?: number = 0) => void;
};
startIndex
and endIndex
can be used to implement client-side pagination. The simplest possible usage is to pass these properties directly to Array.slice
:
const [data] = React.useState(["apple", "banana", "cherry"]);
const { startIndex, endIndex } = usePagination({ totalItems: data.length, initialPageSize: 1 });
return (
<ul>
{data.slice(startIndex, endIndex).map((item) => (
<li>{item}</li>
))}
</ul>
);
startIndex
and pageSize
can be used to implement a standard limit/offset (also known as top/skip) type of pagination:
// Keep track of length separately from data, since data fetcher depends on pagination state
const [length, setLength] = React.useState(0);
// Pagination hook
const { startIndex, pageSize } = usePagination({ totalItems: length, initialPageSize: 1 });
// Fetch Data
const [_, data] = usePromise(
React.useCallback(
() => fetchUsers({ offset: startIndex, limit: pageSize }),
[startIndex, pageSize]
)
);
// When data changes, update length
React.useEffect(() => {
setLength(data.length);
}, [data]);
return (
<ul>
{data.slice(startIndex, endIndex).map((item) => (
<li>{item}</li>
))}
</ul>
);
FAQs
A React hook to help manage pagination state
The npm package react-use-pagination receives a total of 4,995 weekly downloads. As such, react-use-pagination popularity was classified as popular.
We found that react-use-pagination demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.