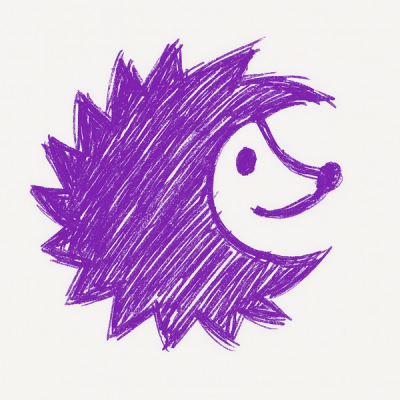
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
react-video-js-player
Advanced tools
React wrapper for VideoJS. Live Demo
npm install --save react-video-js-player
import React, { Component } from 'react';
import VideoPlayer from 'react-video-js-player';
class VideoApp extends Component {
player = {}
state = {
video: {
src: "http://www.example.com/path/to/video.mp4",
poster: "http://www.example.com/path/to/video_poster.jpg"
}
}
onPlayerReady(player){
console.log("Player is ready: ", player);
this.player = player;
}
onVideoPlay(duration){
console.log("Video played at: ", duration);
}
onVideoPause(duration){
console.log("Video paused at: ", duration);
}
onVideoTimeUpdate(duration){
console.log("Time updated: ", duration);
}
onVideoSeeking(duration){
console.log("Video seeking: ", duration);
}
onVideoSeeked(from, to){
console.log(`Video seeked from ${from} to ${to}`);
}
onVideoEnd(){
console.log("Video ended");
}
render() {
return (
<div>
<VideoPlayer
controls={true}
src={this.state.video.src}
poster={this.state.video.poster}
width="720"
height="420"
onReady={this.onPlayerReady.bind(this)}
onPlay={this.onVideoPlay.bind(this)}
onPause={this.onVideoPause.bind(this)}
onTimeUpdate={this.onVideoTimeUpdate.bind(this)}
onSeeking={this.onVideoSeeking.bind(this)}
onSeeked={this.onVideoSeeked.bind(this)}
onEnd={this.onVideoEnd.bind(this)}
/>
</div>
);
}
}
export default VideoApp;
onReady will return
videojs
instance. Which means you can use all the APIs provided by VideoJS.
List of VideoJS APIs
Since most of the VideoJS plugins needs
videojs
instance to get initialized, it is very easy to integrate any of the available plugins by making use ofvideojs
instance returnd by onReady event.
List of VideoJS plugins
Prop Name | Prop Type | Default Value | Description |
---|---|---|---|
src | string | "" | Video file path |
poster | string | "" | Video poster file path |
width | string | number | auto | Video player width |
height | string | number | auto | Video player height |
controls | boolean | true | Video player control bar toggle |
autoplay | boolean | false | Video will start playing automatically if true |
preload | string | auto | video tag preload attribute |
playbackRates | array | [0.5, 1, 1.5, 2] | Video speed control |
hideControls | array | [] | List of controls to hide. ['play','volume','seekbar','timer','playbackrates','fullscreen'] |
bigPlayButton | boolean | true | Big play button visibility toggle |
bigPlayButtonCentered | boolean | true | Big play button center position toggle |
className | string | "" | Video player wrapper class. It can be used for custom player skin. |
Method Name | Description |
---|---|
onReady | It will fire when video player is ready to be used. It returns videojs instance. |
onPlay | It will fire when video starts playing anytime. It returns current time of the video |
onPause | It will fire when video is paused. It returns current time of the video |
onTimeUpdate | It keeps firing while video is in playing state. It returns current time of the video |
onSeeking | It will fire when video is beeing seeked using seekbar. It returns current time of the video |
onSeeked | It will fire after seeking is done. It returns seek start time and seek end time for the video. |
onEnd | It will fire when video is finished playing. |
FAQs
React wrapper for VideoJS
The npm package react-video-js-player receives a total of 1,913 weekly downloads. As such, react-video-js-player popularity was classified as popular.
We found that react-video-js-player demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.