react.js-nested-tree
Advanced tools
Comparing version 1.0.4 to 1.1.0
@@ -1,1 +0,1 @@ | ||
export { default as Tree } from "./treeList"; | ||
export { default as Tree, Item, TreeItem } from "./treeList"; |
export { default } from "./TreeList"; | ||
export { TreeItemProps as TreeItem, Item } from "./types"; |
@@ -1,1 +0,1 @@ | ||
export { TreeListProps, TreeProps, ItemProps, TreeItemProps } from "./types"; | ||
export { TreeListProps, TreeProps, ItemProps, TreeItemProps, Item, } from "./types"; |
@@ -1,2 +0,2 @@ | ||
/// <reference types="react" /> | ||
import React from "react"; | ||
export interface TreeListProps { | ||
@@ -35,3 +35,3 @@ treeData: Item[]; | ||
} | ||
interface Item { | ||
export interface Item { | ||
id: number | string; | ||
@@ -41,2 +41,1 @@ children?: Item[] | null; | ||
} | ||
export {}; |
@@ -1,3 +0,2 @@ | ||
/// <reference types="react" /> | ||
import { FC } from 'react'; | ||
import React, { FC } from 'react'; | ||
@@ -8,2 +7,3 @@ interface TreeListProps { | ||
itemClassName?: string; | ||
subTreeClassName?: string; | ||
ItemComponent: React.ComponentType<TreeItemProps>; | ||
@@ -24,3 +24,3 @@ subTreeStyle?: React.CSSProperties; | ||
id: number | string; | ||
children: Item[] | null | undefined; | ||
children?: Item[] | null; | ||
[key: string]: any; | ||
@@ -31,2 +31,2 @@ } | ||
export { TreeList as Tree }; | ||
export { type Item, TreeList as Tree, type TreeItemProps as TreeItem }; |
{ | ||
"name": "react.js-nested-tree", | ||
"version": "1.0.4", | ||
"version": "1.1.0", | ||
"main": "dist/cjs/index.js", | ||
"description": "This is react.js nested tree component", | ||
"description": "The react.js-nested-tree package is a versatile React component designed for creating nested, hierarchical tree structures. This package is especially useful for applications that require a visual representation of nested data, such as file explorers, category trees, or organizational charts.", | ||
"files": [ | ||
@@ -7,0 +7,0 @@ "dist" |
426
README.md
# react.js-nested-tree | ||
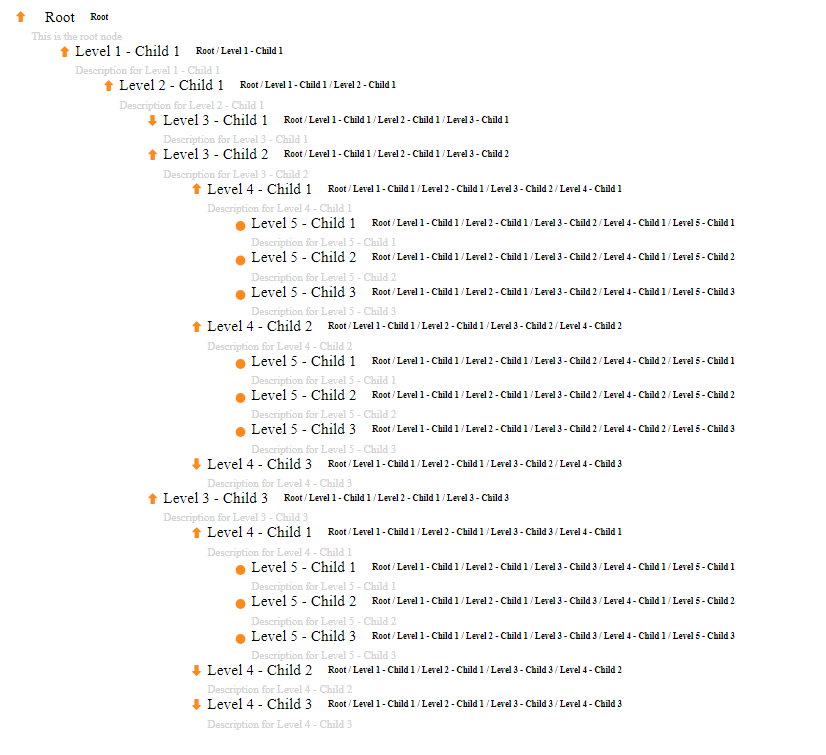 | ||
## Project Structure | ||
``` | ||
react.js-nested-tree/ | ||
├── src/ | ||
│ ├── assets | ||
│ ├── TreeItems.tsx | ||
│ │── index.ts | ||
| │── App.tsx | ||
| └── App.css | ||
│ | ||
├── package.json | ||
├── package-lock.json | ||
└── .gitignore | ||
## Installation: | ||
```bash | ||
npm install react.js-nested-tree | ||
# or | ||
yarn add react.js-nested-tree | ||
``` | ||
## Package Configuration | ||
## Key Features: | ||
```json | ||
{ | ||
"name": "react_nested-tree", | ||
"private": true, | ||
"version": "0.0.0", | ||
"type": "module", | ||
"scripts": { | ||
"dev": "vite", | ||
"build": "tsc && vite build", | ||
"lint": "eslint . --ext ts,tsx --report-unused-disable-directives --max-warnings 0", | ||
"preview": "vite preview" | ||
}, | ||
"dependencies": { | ||
"react": "^18.2.0", | ||
"react-dom": "^18.2.0", | ||
"react.js-nested-tree": "^1.0.0" | ||
}, | ||
- Declarative Approach: Allows developers to define tree structures using a simple and intuitive JSON format. | ||
- Dynamic Data Handling: Supports dynamic loading of tree nodes, making it suitable for large datasets or data fetched from remote sources. | ||
- Customizable Appearance: Provides options for customizing the appearance and behavior of tree nodes, including icons, styles, and animations. | ||
- Interactivity: Supports interactive features such as node selection, expansion, and collapse, making it user-friendly and interactive. | ||
- Performance Optimization: Designed with performance in mind, ensuring smooth operation even with complex and deeply nested structures. | ||
} | ||
``` | ||
## Usage | ||
@@ -52,5 +28,21 @@ | ||
import { TreeItems } from "./TreeItems"; | ||
import { treeData } from "./treeData"; | ||
import { Tree } from "react.js-nested-tree"; | ||
function App() { | ||
const treeData = [ | ||
{ | ||
id: 1, | ||
name: "Root Node", | ||
children: [ | ||
{ | ||
id: 2, | ||
name: "Child Node 1", | ||
children: [ | ||
{ id: 3, name: "Grandchild Node 1" }, | ||
{ id: 4, name: "Grandchild Node 2" }, | ||
], | ||
}, | ||
{ id: 5, name: "Child Node 2" }, | ||
], | ||
}, | ||
]; | ||
return ( | ||
@@ -71,362 +63,14 @@ <div> | ||
- ./TreeItems | ||
## API: | ||
```tsx | ||
import arrow from "./assets/arrow.svg"; | ||
import circle from "./assets/circle.svg"; | ||
- data: An array of objects representing the tree structure. Each object should have a unique id property. Optional children properties can be added for nested nodes. | ||
- Customization Options: Various props are available for customization, including node rendering functions, event handlers for node actions (e.g., onNodeClick, onNodeExpand), and styling options. | ||
export const TreeItems = ({ isParent, item, path, open, setOpen }: any) => { | ||
return ( | ||
<div className="container"> | ||
<div | ||
className="icon_container" | ||
onClick={() => { | ||
setOpen((open: any) => !open); | ||
}} | ||
> | ||
{isParent ? ( | ||
<img | ||
src={arrow} | ||
alt="arrow" | ||
className="icon" | ||
style={{ rotate: open && "180deg" }} | ||
/> | ||
) : ( | ||
<img | ||
src={circle} | ||
alt="circle" | ||
className="icon" | ||
width={22} | ||
height={22} | ||
/> | ||
)} | ||
</div> | ||
<div className="info_container"> | ||
<div className="info_box"> | ||
<span className="title">{item?.name} </span> | ||
<span className="path"> | ||
{path?.map((p: any) => p.name).join(" / ")} | ||
</span> | ||
</div> | ||
</div> | ||
</div> | ||
); | ||
}; | ||
``` | ||
## Conclusion: | ||
- ./App.css | ||
The react.js-nested-tree package offers a robust solution for displaying hierarchical data in a React application. Its flexibility, ease of use, and performance optimizations make it an excellent choice for developers looking to implement nested tree structures with minimal hassle. | ||
```css | ||
.container { | ||
display: flex; | ||
gap: 4px; | ||
justify-content: start; | ||
align-items: start; | ||
} | ||
.info_container, | ||
.icon_container { | ||
cursor: pointer; | ||
} | ||
.info_box { | ||
display: flex; | ||
justify-content: center; | ||
align-items: center; | ||
gap: 14px; | ||
} | ||
.description { | ||
font-size: 10px; | ||
color: lightgray; | ||
align-self: flex-start; | ||
} | ||
.path { | ||
font-size: 8px; | ||
font-weight: bold; | ||
color: black; | ||
} | ||
.title { | ||
font-size: 14px; | ||
} | ||
.icon { | ||
width: 12px; | ||
} | ||
.info_container { | ||
display: flex; | ||
gap: 4px; | ||
justify-content: start; | ||
align-items: center; | ||
} | ||
``` | ||
### Demo | ||
./treeData | ||
> [react-js-nested-tree-demo](https://codesandbox.io/p/live/06e9de8a-a26d-43f5-909c-3e15204b2b2a) | ||
```js | ||
export const treeData = [ | ||
{ | ||
id: "1", | ||
name: "Root", | ||
description: "This is the root node", | ||
children: [ | ||
{ | ||
id: "2", | ||
name: "Level 1 - Child 1", | ||
description: "Description for Level 1 - Child 1", | ||
children: [ | ||
{ | ||
id: "3", | ||
name: "Level 2 - Child 1", | ||
description: "Description for Level 2 - Child 1", | ||
children: [ | ||
{ | ||
id: "4", | ||
name: "Level 3 - Child 1", | ||
description: "Description for Level 3 - Child 1", | ||
children: [ | ||
{ | ||
id: "5", | ||
name: "Level 4 - Child 1", | ||
description: "Description for Level 4 - Child 1", | ||
children: [ | ||
{ | ||
id: "6", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "7", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "8", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "9", | ||
name: "Level 4 - Child 2", | ||
description: "Description for Level 4 - Child 2", | ||
children: [ | ||
{ | ||
id: "10", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "11", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "12", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "13", | ||
name: "Level 4 - Child 3", | ||
description: "Description for Level 4 - Child 3", | ||
children: [ | ||
{ | ||
id: "14", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "15", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "16", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "17", | ||
name: "Level 3 - Child 2", | ||
description: "Description for Level 3 - Child 2", | ||
children: [ | ||
{ | ||
id: "18", | ||
name: "Level 4 - Child 1", | ||
description: "Description for Level 4 - Child 1", | ||
children: [ | ||
{ | ||
id: "19", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "20", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "21", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "22", | ||
name: "Level 4 - Child 2", | ||
description: "Description for Level 4 - Child 2", | ||
children: [ | ||
{ | ||
id: "23", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "24", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "25", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "26", | ||
name: "Level 4 - Child 3", | ||
description: "Description for Level 4 - Child 3", | ||
children: [ | ||
{ | ||
id: "27", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "28", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "29", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "30", | ||
name: "Level 3 - Child 3", | ||
description: "Description for Level 3 - Child 3", | ||
children: [ | ||
{ | ||
id: "31", | ||
name: "Level 4 - Child 1", | ||
description: "Description for Level 4 - Child 1", | ||
children: [ | ||
{ | ||
id: "32", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "33", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "34", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "35", | ||
name: "Level 4 - Child 2", | ||
description: "Description for Level 4 - Child 2", | ||
children: [ | ||
{ | ||
id: "36", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "37", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "38", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
{ | ||
id: "39", | ||
name: "Level 4 - Child 3", | ||
description: "Description for Level 4 - Child 3", | ||
children: [ | ||
{ | ||
id: "40", | ||
name: "Level 5 - Child 1", | ||
description: "Description for Level 5 - Child 1", | ||
children: null, | ||
}, | ||
{ | ||
id: "41", | ||
name: "Level 5 - Child 2", | ||
description: "Description for Level 5 - Child 2", | ||
children: null, | ||
}, | ||
{ | ||
id: "42", | ||
name: "Level 5 - Child 3", | ||
description: "Description for Level 5 - Child 3", | ||
children: null, | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
]; | ||
``` |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
10828
16
91
75