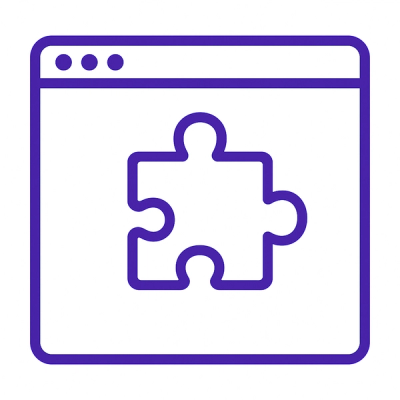
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
The `read-cache` npm package is designed to read and cache the contents of files in memory. This can significantly improve performance for applications that need to read the same files multiple times, as it avoids unnecessary disk I/O after the initial read. The cache is automatically updated if the file changes, ensuring that the application always has access to the latest version of the file contents.
Reading and caching file contents
This feature allows you to read the contents of a file and cache it for future reads. The function returns a promise that resolves with the file's contents.
const readCache = require('read-cache');
readCache('path/to/file.txt').then(contents => {
console.log(contents);
});
Synchronously reading and caching file contents
Similar to the asynchronous version, this feature allows for synchronous reading and caching of file contents, useful in scenarios where asynchronous operations are not possible or desired.
const readCache = require('read-cache');
const contents = readCache.sync('path/to/file.txt');
console.log(contents);
While `node-cache` is a general-purpose in-memory cache module for Node.js and does not specifically target file content caching, it can be used to manually implement a similar functionality by reading file contents and storing them in the cache. Compared to `read-cache`, `node-cache` offers more flexibility in what can be cached but requires more setup for caching file contents.
This package provides a simple in-memory cache similar to `node-cache`. It can be used to cache any JavaScript object, including file contents. Like `node-cache`, it offers more general caching capabilities but lacks the file-specific optimizations and automatic updates provided by `read-cache`.
Reads and caches the entire contents of a file until it is modified.
$ npm i read-cache
// foo.js
var readCache = require('read-cache');
readCache('foo.js').then(function (contents) {
console.log(contents);
});
Returns a promise that resolves with the file's contents.
Returns the content of the file.
Returns the content of cached file or null.
Clears the contents of the cache.
MIT © Bogdan Chadkin
FAQs
Reads and caches the entire contents of a file until it is modified
The npm package read-cache receives a total of 12,359,631 weekly downloads. As such, read-cache popularity was classified as popular.
We found that read-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.