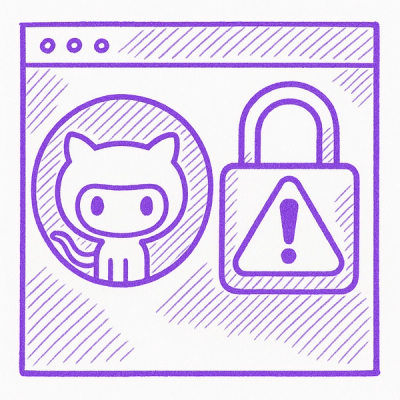
Research
/Security News
Toptal’s GitHub Organization Hijacked: 10 Malicious Packages Published
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.
readdir-glob
Advanced tools
The readdir-glob npm package is designed to read directories and list files in them, potentially filtering by patterns using glob syntax. It combines the functionality of reading directories recursively (like `fs.readdir` or `fs.readdirSync` but with support for deep traversal) and filtering files using glob patterns, which specify sets of filenames with wildcard characters.
Reading directories with glob patterns
This feature allows you to read through directories and subdirectories to find files that match a specific glob pattern. In the provided code sample, `readdir-glob` is used to find all JavaScript files (`**/*.js`) in the current directory and its subdirectories. Events like 'match' and 'end' are used to handle the found files and the completion of the directory reading process.
const readdirGlob = require('readdir-glob');
const baseDirectory = '.';
const options = { glob: '**/*.js' };
const globber = readdirGlob(baseDirectory, options);
globber.on('match', function(match) {
console.log('Matched file:', match);
});
globber.on('end', function() {
console.log('Finished reading directory.');
});
Filtering with negation patterns
This feature demonstrates how to use negation patterns to exclude certain directories or files from the search. In the example, all JavaScript files are matched except those within any `node_modules` directory. This is particularly useful for node.js projects where you might want to ignore dependencies.
const readdirGlob = require('readdir-glob');
const baseDirectory = '.';
const options = { glob: ['**/*.js', '!**/node_modules/**'] };
const globber = readdirGlob(baseDirectory, options);
globber.on('match', function(match) {
console.log('Matched file:', match);
});
globber.on('end', function() {
console.log('Finished reading directory.');
});
The `glob` package is one of the most popular packages for matching files using the glob syntax. It provides a similar functionality to `readdir-glob` but focuses more on the pattern matching aspect and does not inherently support reading directories in a structured manner like `readdir-glob` does.
Similar to `readdir-glob`, `fast-glob` allows for searching files using glob patterns. It is designed to be faster and more efficient, especially for large file systems. It supports all the basic features of glob syntax and adds some additional options for performance optimization.
This package is another alternative that provides glob functionality in Node.js environments. While similar in purpose to `readdir-glob`, `node-glob` focuses more on the globbing aspect and might not offer the same level of directory traversal and reading capabilities.
Recursive version of fs.readdir wih stream API and glob filtering.
Uses the minimatch
library to do its matching.
Requirements:
Compared to glob
, readdir-glob
is memory efficient: no matter the file system size, or the number of returned files, the memory usage is constant.
The CPU cost is proportional to the number of files in root
folder, minus the number files in options.skip
folders.
Advice: For better performances use options.skip
to restrict the search as much as possible.
Install with npm
npm i readdir-glob
const readdirGlob = require('readdir-glob');
const globber = readdirGlob('.', {pattern: '**/*.js'});
globber.on('match', match => {
// m.relative: relative path of the matched file
// m.absolute: absolute path of the matched file
// m.stat: stat of the matched file (only if stat:true option is used)
});
globber.on('error', err => {
console.error('fatal error', err);
});
globber.on('end', (m) => {
console.log('done');
});
root
{String}
Path to be read recursively, default: '.'
options
{Object}
Options, default: {}
Returns a EventEmitter reading given root recursively.
options
: The options object passed in.paused
: Boolean which is set to true when calling pause()
.aborted
Boolean which is set to true when calling abort()
. There is no way at this time to continue a glob search after aborting.match
: Every time a match is found, this is emitted with the specific thing that matched.end
: When the matching is finished, this is emitted with all the matches found.error
: Emitted when an unexpected error is encountered.pause()
: Temporarily stop the searchresume()
: Resume the searchabort()
: Stop the search foreverpattern
: Glob pattern or Array of Glob patterns to match the found files with. A file has to match at least one of the provided patterns to be returned.ignore
: Glob pattern or Array of Glob patterns to exclude matches. If a file or a folder matches at least one of the provided patterns, it's not returned. It doesn't prevent files from folder content to be returned. Note: ignore
patterns are always in dot:true
mode.skip
: Glob pattern or Array of Glob patterns to exclude folders. If a folder matches one of the provided patterns, it's not returned, and it's not explored: this prevents any of its children to be returned. Note: skip
patterns are always in dot:true
mode.mark
: Add a /
character to directory matches.stat
: Set to true to stat all results. This reduces performance.silent
: When an unusual error is encountered when attempting to read a directory, a warning will be printed to stderr. Set the silent
option to true to suppress these warnings.nodir
: Do not match directories, only files.follow
: Follow symlinked directories. Note that requires to stat all results, and so reduces performance.The following options apply only if pattern
option is set, and are forwarded to minimatch
:
dot
: Allow pattern
to match filenames starting with a period, even if the pattern does not explicitly have a period in that spot.noglobstar
: Disable **
matching against multiple folder names.nocase
: Perform a case-insensitive match. Note: on case-insensitive filesystems, non-magic patterns will match by default, since stat
and readdir
will not raise errors.matchBase
: Perform a basename-only match if the pattern does not contain any slash characters. That is, *.js
would be treated as equivalent to **/*.js
, matching all js files in all directories.Unit-test set is based on node-glob tests.
FAQs
Recursive fs.readdir with streaming API and glob filtering.
The npm package readdir-glob receives a total of 9,164,569 weekly downloads. As such, readdir-glob popularity was classified as popular.
We found that readdir-glob demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.
Research
/Security News
Socket researchers investigate 4 malicious npm and PyPI packages with 56,000+ downloads that install surveillance malware.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.