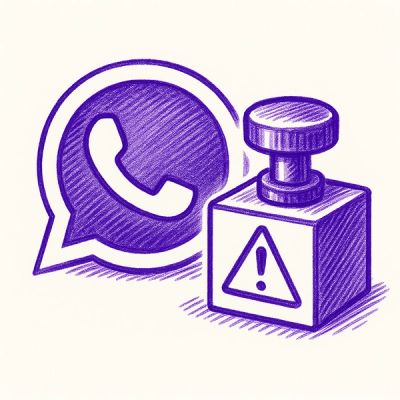
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Scan through all redis keys and containers, calling back each value individually.
Recursively scans the keyspace of a Redis 2.8+ instance using SCAN, HSCAN, ZSCAN, & SSCAN as well as Lists.
Fairly safe in a production environment as it does NOT use KEYS * to iterate.
Optionally pass a redis pattern to filter from.
npm install redisscan
var redisScan = require('redisscan');
var redis = require('redis').createClient();
redisScan({
redis: redis,
pattern: 'awesome:key:prefix:*',
keys_only: false,
each_callback: function (type, key, subkey, length, value, cb) {
console.log(type, key, subkey, length, value);
cb();
},
done_callback: function (err) {
console.log("-=-=-=-=-=--=-=-=-");
redis.quit();
}
});
redis
: required node-redis
client instancepattern
: optional wildcard key pattern to match, e.g: some:key:pattern:*
docskeys_only
: optional boolean -- returns nothing but keys, no types,lengths,values etc. (defaults to false
)count_amt
: optional positive/non-zero integer -- redis hint for work done per SCAN operation (defaults to 10) docseach_callback
: required function (type, key, subkey, length, value, next)
This is called for every string, and every subkey/value in a container when not using keys_only
, so outer keys may show up multiple times.
type
may be "string"
, "hash"
, "set"
, "zset"
, "list"
key
is the redis keysubkey
may be null
or populated with a hash keylength
is the length of a set or listvalue
is the value of the key or subkey when appropriatenext()
should be called as a function with no arguments if successful or an Error
object if not.done_callback
: optional function called when scanning completes with one argument, and Error
object if an error ws raisedIf values are changing, there is no guarantee on value integrity. This is not atomic. I recommend using a lock pattern with this function.
License MIT (c) 2014 Nathanael C. Fritz
FAQs
Scan through all redis keys and containers, calling back each value individually.
The npm package redisscan receives a total of 511 weekly downloads. As such, redisscan popularity was classified as not popular.
We found that redisscan demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.